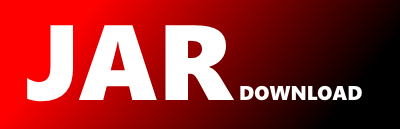
com.sap.it.commons.jmx.JMXTools Maven / Gradle / Ivy
package com.sap.it.commons.jmx;
import java.io.IOException;
import java.lang.management.ManagementFactory;
import javax.management.AttributeNotFoundException;
import javax.management.InstanceAlreadyExistsException;
import javax.management.InstanceNotFoundException;
import javax.management.MBeanException;
import javax.management.MBeanRegistrationException;
import javax.management.MBeanServer;
import javax.management.MBeanServerConnection;
import javax.management.NotCompliantMBeanException;
import javax.management.NotificationBroadcaster;
import javax.management.ObjectName;
import javax.management.ReflectionException;
import javax.management.StandardMBean;
import com.sap.it.commons.lang.Checks;
/**
* The {@link JMXTools} simplifies the usage of JMX. It offers a set of static
* operations for registering and unregistering MBeans at the MBean server.
*/
public final class JMXTools {
// Not instantiable.
private JMXTools() {
}
/**
* Unregisters the MBean with the given object name.
*
* @param objectName
* The object name of a registered MBean
* @throws InstanceNotFoundException
* @throws MBeanRegistrationException
*/
public static void unregisterMBean(ObjectName objectName) throws MBeanRegistrationException, InstanceNotFoundException {
MBeanServer platformMBeanServer = ManagementFactory.getPlatformMBeanServer();
if (platformMBeanServer.isRegistered(objectName)) {
platformMBeanServer.unregisterMBean(objectName);
}
}
/**
* Registers the object
under the given objectName
* at the local MBean server
*
* @param object
* The MBean implementation implementing
* mbeanInterface
* @param objectName
* The object name that identifies the MBean in the server
*
* @throws NotCompliantMBeanException
* @throws MBeanRegistrationException
* @throws InstanceAlreadyExistsException
*/
public static final void registerMBean(Object object, ObjectName objectName) throws InstanceAlreadyExistsException,
MBeanRegistrationException, NotCompliantMBeanException {
ManagementFactory.getPlatformMBeanServer().registerMBean(object, objectName);
}
/**
* Wraps the mbeanImplementation
implementing the
* mbeanImplementation
interface
*
* @param
* Compiler check that mbeanImplementation
* implements mbeanInterface
* @param mbeanImplementation
* The MBean implementation implementing
* mbeanInterface
* @param mbeanInterface
* The MBean interface
* @throws NotCompliantMBeanException
*/
public static final StandardMBean wrapAnnotatedStandardMBean(T mbeanImplementation, Class mbeanInterface)
throws NotCompliantMBeanException {
return new AnnotatedStandardMBean(mbeanImplementation, mbeanInterface);
}
/**
* Wraps the mbeanImplementation
implementing the
* mbeanImplementation
interface
*
* mbeanImplementation
is able to process JMX event
* notifications.
*
* @param
* Compiler check that mbeanImplementation
* implements mbeanInterface
* @param mbeanImplementation
* The MBean implementation implementing
* mbeanInterface
* @param mbeanInterface
* The MBean interface
* @throws NotCompliantMBeanException
*/
public static final StandardMBean wrapAnnotatedBroadcastingMBean(T mbeanImplementation, Class mbeanInterface)
throws NotCompliantMBeanException {
if (!(mbeanImplementation instanceof NotificationBroadcaster)) {
throw new IllegalArgumentException("Implementation does not implement the NotificationBroadcaster interface.");
}
return new AnnotatedBroadcastingStandardMBean(mbeanImplementation, mbeanInterface);
}
//
// Convenience methods to wrap potential JMX exceptions with JMXRuntimeException
//
/**
* Gets an MBean attribute by invoking
* {@link MBeanServerConnection#getAttribute(ObjectName name, String attribute)}
* an wraps all exception by {@link JMXRuntimeException}.
*
* @param connection
* MBeanServerConnection
* @param name
* @param attribute
*
* @see MBeanServerConnection#getAttribute(ObjectName name, String
* attribute)
*
* @return
*/
public static Object getMBeanAttributeWrapExceptions(MBeanServerConnection connection, ObjectName name, String attribute) {
Checks.nonNull(name, "objectName must not be null");
Checks.nonNull(attribute, "attribute must not be null");
Exception ex = null;
try {
return connection.getAttribute(name, attribute);
} catch (InstanceNotFoundException e) {
ex = e;
} catch (ReflectionException e) {
ex = e;
} catch (AttributeNotFoundException e) {
ex = e;
} catch (MBeanException e) {
ex = e;
} catch (IOException e) {
ex = e;
}
String message = "Failed to aquire " + attribute + " for ObjectName = " + name;
throw new JMXRuntimeException(message, ex);
}
public static void unregisterMBeanWrapExceptions(ObjectName objectName) {
try {
unregisterMBean(objectName);
} catch (MBeanRegistrationException e) {
throw new JMXRuntimeException(e);
} catch (InstanceNotFoundException e) {
throw new JMXRuntimeException(e);
}
}
public static final void registerMBeanWrapExceptions(Object object, ObjectName objectName) {
try {
registerMBean(object, objectName);
} catch (InstanceAlreadyExistsException e) {
throw new JMXRuntimeException(e);
} catch (MBeanRegistrationException e) {
throw new JMXRuntimeException(e);
} catch (NotCompliantMBeanException e) {
throw new JMXRuntimeException(e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy