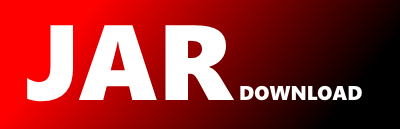
com.sap.it.commons.jmx.MBeanInfoCache Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-core Show documentation
Show all versions of odata-core Show documentation
SAP Cloud Platform SDK for service development
The newest version!
package com.sap.it.commons.jmx;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
import java.util.concurrent.ConcurrentHashMap;
import javax.management.MBeanAttributeInfo;
import javax.management.MBeanOperationInfo;
import javax.management.MBeanParameterInfo;
class MBeanInfoCache {
private static final Map> PRIMITIVE_CLASSES = new HashMap>(16);
static {
Class>[] prims = { boolean.class, byte.class, short.class, int.class, long.class, float.class, double.class, char.class };
for (Class> c : prims) {
PRIMITIVE_CLASSES.put(c.getName(), c);
}
}
private final Class> mbeanInterface;
private final Map attributes = new ConcurrentHashMap();
private final Map operations = new ConcurrentHashMap();
public MBeanInfoCache(Class> mbeanInterface) {
this.mbeanInterface = mbeanInterface;
}
public Method methodFor(MBeanOperationInfo op) {
Method result = operations.get(op);
if (result == null) {
final MBeanParameterInfo[] params = op.getSignature();
final String[] paramTypes = new String[params.length];
for (int i = 0; i < params.length; i++) {
paramTypes[i] = params[i].getType();
}
result = findMethod(op.getName(), paramTypes);
if (result != null) {
operations.put(op, result);
}
}
return result;
}
public Method methodFor(MBeanAttributeInfo attr) {
Method result = attributes.get(attr);
if (result == null) {
String methodWithAnnotation;
if (attr.isIs()) {
methodWithAnnotation = "is" + attr.getName(); //$NON-NLS-1$
} else {
methodWithAnnotation = "get" + attr.getName(); //$NON-NLS-1$
}
// Open issue: is it possible to have a writeble non-readable
// attribute?
result = findMethod(methodWithAnnotation, new String[0]);
if (result != null) {
attributes.put(attr, result);
}
}
return result;
}
public Class> getMbeanInterface() {
return mbeanInterface;
}
private final Method findMethod(String name, String... paramTypes) {
try {
final ClassLoader loader = mbeanInterface.getClassLoader();
final Class>[] paramClasses = new Class[paramTypes.length];
for (int i = 0; i < paramTypes.length; i++) {
paramClasses[i] = classForName(paramTypes[i], loader);
}
return mbeanInterface.getMethod(name, paramClasses);
} catch (RuntimeException e) {
// avoid accidentally catching unexpected runtime exceptions
throw e;
} catch (Exception e) {
return null;
}
}
private static Class> classForName(String name, ClassLoader loader) throws ClassNotFoundException {
Class> c = PRIMITIVE_CLASSES.get(name);
if (c == null) {
c = Class.forName(name, false, loader);
}
return c;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy