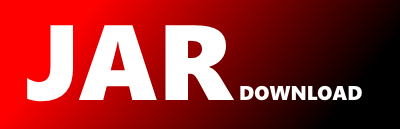
com.sap.it.commons.structure.StructureTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-core Show documentation
Show all versions of odata-core Show documentation
SAP Cloud Platform SDK for service development
The newest version!
package com.sap.it.commons.structure;
import com.sap.it.commons.lang.Checks;
public class StructureTools {
private static final String HOST_MUST_NOT_BE_NULL = "Host must not be null";
private StructureTools() {
}
/**
* Peek for an adapter of an {@link Adaptable} object.
*
* @param host
* Adaptable object that can be {@code null}.
* @param adapterClass
* class to adapt to.
* @return {@code null} if host is {@code null} else the result is
* {@link Adaptable#getAdapter(Class)}.
*/
public static T peekAdapter(Adaptable host, Class adapterClass) {
return host != null ? host.getAdapter(adapterClass) : null;
}
/**
* Peek for an adapter for an object that potentially implements
* {@link Adaptable} interface.
*
* @param host
* any object that can be {@code null}.
* @param adapterClass
* class to adapt to.
* @return {@code null} if host is {@code null} or the object does not
* implement the {@link Adaptable} interface else the result is
* {@link Adaptable#getAdapter(Class)}.
*/
public static T peekAdapter(Object potentialHost, Class adapterClass) {
if (potentialHost instanceof Adaptable) {
Adaptable adaptable = (Adaptable) potentialHost;
return adaptable.getAdapter(adapterClass);
} else {
return null;
}
}
/**
* Get an adapter for an {@link Adaptable} object. Result is never
* {@code null}.
*
* @param host
* any object. Throws IllegalArgumentException if {@code null}.
* @param adapterClass
* class to adapt to.
* @return the adapter object. Is never {@code null}.
* @throws IllegalArgumentException
* if host is {@code null} or no adapter has been found.
*/
public static T grantAdapter(Adaptable host, Class adapterClass) {
Checks.nonNullArgument(host, HOST_MUST_NOT_BE_NULL);
return returnAdapter(host.getAdapter(adapterClass), adapterClass);
}
/**
* Get an adapter for an object that potentially implements
* {@link Adaptable} interface. Result is never {@code null}.
*
* @param host
* any object. Throws IllegalArgumentException if {@code null}.
* @param adapterClass
* class to adapt to.
* @return the adapter object. Is never {@code null}.
* @throws IllegalArgumentException
* if host is {@code null} or no adapter has been found.
*/
public static T grantAdapter(Object potentialHost, Class adapterClass) {
if (potentialHost instanceof Adaptable) {
Adaptable adaptable = (Adaptable) potentialHost;
return returnAdapter(adaptable.getAdapter(adapterClass), adapterClass);
} else {
Checks.nonNullArgument(potentialHost, HOST_MUST_NOT_BE_NULL);
throw new IllegalArgumentException("Host is not adaptable");
}
}
private static final T returnAdapter(T t, Class adapterClass) {
if (t != null) {
return t;
}
throw new IllegalArgumentException("Host does not provide adapter for type: "
+ (adapterClass != null ? adapterClass.getName() : "null"));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy