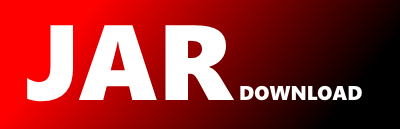
com.sap.it.commons.tools.CollectionTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-core Show documentation
Show all versions of odata-core Show documentation
SAP Cloud Platform SDK for service development
The newest version!
package com.sap.it.commons.tools;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import java.util.NoSuchElementException;
public final class CollectionTools {
/**
* Private constructor to avoid instantiation of this class.
*/
private CollectionTools() {
}
/**
* Returns the the initialCapacity value for creating a (Hash-)Map that can
* store elements
without reallocation.
*
* @param elements
* number of elements to be stored.
*
* @return the initialCapacity value for the Map constructor.
*/
public static int computeMapInitialSize(int elements) {
return Math.max((int) (elements / 0.75) + 1, 16);
}
/**
* Return the first element of a collection. If the collection is empty null
* is returned.
*
* @param collection
* @return the first element or {@code null}.
*/
public static T firstOf(Collection collection) {
if (collection == null || collection.isEmpty()) {
return null;
}
if (collection instanceof List) {
return ((List) collection).get(0);
} else {
return collection.iterator().next();
}
}
/**
* Return if a collection at least contains one element.
*
* @param collection
* @return true if the collection is neither empty nor null.
*/
public static boolean hasContent(Collection> collection) {
return collection != null && !collection.isEmpty();
}
/**
* Return if a collection is null or empty.
*
* @param collection
* @return true if the collection is empty or null.
*/
public static boolean isEmpty(Collection> collection) {
return collection == null || collection.isEmpty();
}
/**
* Cluster a collection into part of size elements. If last part has
* potentially less than size elements.
*
* @param collection
* collection to cluster
* @param clusterSize
* maximum elements per cluster
* @return allays a collection is returned. Containing no elements if the
* collection input parameter was null or an empty collection.
*/
public static Collection> cluster(Collection collection, int clusterSize) {
if (clusterSize <= 0) {
throw new IllegalArgumentException("clusterSize must be greater than 0");
}
if (isEmpty(collection)) {
return Collections.emptyList();
}
List> res = new ArrayList>();
Iterator it = collection.iterator();
while (it.hasNext()) {
ArrayList part = new ArrayList();
int cnt = clusterSize;
do {
part.add(it.next());
} while ((--cnt > 0) && it.hasNext());
res.add(part);
}
return res;
}
public static String asString(Collection> collection, String separator, String collectionEmptyValue, boolean skipNullValues) {
if (CollectionTools.isEmpty(collection)) {
return collectionEmptyValue;
}
final StringBuilder sb = new StringBuilder();
for (Object o : collection) {
if (skipNullValues && o == null) {
continue;
}
if (sb.length() > 0) {
sb.append(separator);
}
sb.append(o);
}
if (skipNullValues && sb.length() == 0) {
return collectionEmptyValue;
}
return sb.toString();
}
public static String asString(Collection> collection, String separator, String collectionEmptyValue) {
return asString(collection, separator, collectionEmptyValue, false);
}
public static String asString(Collection> collection, String separator) {
return asString(collection, separator, StringTools.EMPTY_STRING);
}
public static > T nullify(T data) {
return data == null || data.isEmpty() ? null : data;
}
@SuppressWarnings("rawtypes")
public static Iterator EMPTY_ITERATOR = new Iterator() {
@Override
public boolean hasNext() {
return false;
}
@Override
public Object next() {
throw new NoSuchElementException();
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
};
@SuppressWarnings("unchecked")
public static Iterator emptyIterator() {
return (Iterator) EMPTY_ITERATOR;
}
@SuppressWarnings("rawtypes")
public static Iterable EMPTY_ITERABLE = new Iterable() {
@Override
public Iterator iterator() {
return emptyIterator();
}
};
@SuppressWarnings("unchecked")
public static final Iterable emptyIterable() {
return (Iterable) EMPTY_ITERABLE;
}
public static final boolean addAll(Collection collection, Iterator extends E> iterator) {
boolean res = false;
while (iterator.hasNext()) {
res |= collection.add(iterator.next());
}
return res;
}
public static final boolean addAll(Collection collection, Iterable extends E> iterable) {
boolean res = false;
for (E o : iterable) {
res |= collection.add(o);
}
return res;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy