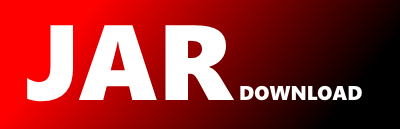
com.sap.it.commons.tools.DigestTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-core Show documentation
Show all versions of odata-core Show documentation
SAP Cloud Platform SDK for service development
The newest version!
package com.sap.it.commons.tools;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class DigestTools {
private DigestTools() {
}
public static final String MD5 = "md5";
static MessageDigest getDigest(String digest) {
try {
return MessageDigest.getInstance(digest);
} catch (NoSuchAlgorithmException e) {
throw new IllegalArgumentException("Digest " + digest + " not found", e);
}
}
private static final char[] HEX_DIGITS = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F' };
static char[] encodeHexAsChars(byte[] data) {
int length = data.length;
char[] res = new char[length * 2];
int j = 0;
for (int i = 0; i < length; ++i) {
res[j++] = HEX_DIGITS[(0xF0 & data[i]) >>> 4];
res[j++] = HEX_DIGITS[(0x0F & data[i])];
}
return res;
}
static String encodeHexString(byte[] data) {
return String.valueOf(encodeHexAsChars(data));
}
public static String compute(String algorithm, String data) {
MessageDigest dig = getDigest(algorithm);
int length = data.length();
for (int i = 0; i < length; ++i) {
char c = data.charAt(i);
dig.update((byte) (c >> 8));
dig.update((byte) (c));
}
return encodeHexString(dig.digest());
}
public static String computeMD5(String data) {
return compute(MD5, data);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy