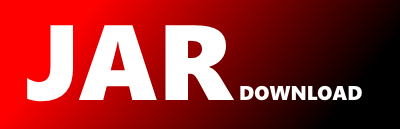
com.sap.it.commons.tools.IoTools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odata-core Show documentation
Show all versions of odata-core Show documentation
SAP Cloud Platform SDK for service development
The newest version!
package com.sap.it.commons.tools;
import java.io.Closeable;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.logging.Level;
import java.util.logging.Logger;
public class IoTools {
private static final String LOGGER_NAME = IoTools.class.getName();
private static final int DEFAULT_BLOCK_SIZE = 1024 * 4;
public static final OutputStream NULL_OUTPUT_STREAM = new OutputStream() {
@Override
public void write(int b) {
}
@Override
public void write(byte[] b, int off, int len) {
}
@Override
public void write(byte[] b) {
}
};
private IoTools() {
}
public static IOException closeConsumeException(Closeable closeable, String details) {
try {
close(closeable);
return null;
} catch (IOException e) {
Logger.getLogger(LOGGER_NAME).log(Level.WARNING, "Failed to close {0}", details != null ? details : closeable.toString());
return e;
}
}
public static IOException closeConsumeException(Closeable closeable) {
return closeConsumeException(closeable, null);
}
public static void close(Closeable closeable) throws IOException {
if (closeable != null) {
closeable.close();
}
}
/**
* Copies InputStream to OutputStream up to a specified amount of bytes.
*
* @param is
* the InputStream
* @param os
* the OutputStream
* @param max
* maximum number of bytes to be copied
* @return number of copied bytes
* @throws IOException
*/
public static long copy(InputStream is, OutputStream os, long max) throws IOException {
byte[] buff = new byte[DEFAULT_BLOCK_SIZE];
int count = 0;
long total = 0;
int len = (int) Math.min(max, (long) DEFAULT_BLOCK_SIZE);
while (len > 0 && (count = is.read(buff, 0, len)) > 0) {
os.write(buff, 0, count);
total += count;
len = (int) Math.min(max - total, (long) DEFAULT_BLOCK_SIZE);
}
return total;
}
public static long copy(InputStream is, OutputStream os) throws IOException {
byte[] buff = new byte[DEFAULT_BLOCK_SIZE];
int count = 0;
long total = 0;
while ((count = is.read(buff)) > 0) {
os.write(buff, 0, count);
total += count;
}
return total;
}
public static long consume(InputStream is) throws IOException {
return copy(is, NULL_OUTPUT_STREAM);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy