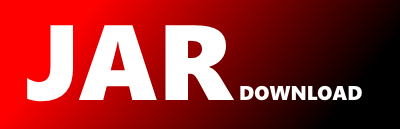
com.sap.it.commons.tools.JAXBTools Maven / Gradle / Ivy
package com.sap.it.commons.tools;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.io.StringWriter;
import javax.xml.bind.JAXBContext;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.JAXBException;
import javax.xml.bind.Marshaller;
import javax.xml.bind.Unmarshaller;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.namespace.QName;
import javax.xml.transform.stream.StreamSource;
public final class JAXBTools {
private final JAXBContext jc;
private final Class clazz;
/**
* Create JAXBTools for the given class. Can be used for caching purposes to
* not create JAXBContext during every call
*
* @param clazz
* @throws JAXBException
*/
public JAXBTools(Class clazz) throws JAXBException {
this.clazz = clazz;
jc = JAXBContext.newInstance(clazz);
}
@SuppressWarnings({ "unchecked", "rawtypes" })
public static Object wrap(Object obj) {
if (obj != null) {
XmlRootElement xmlRoot = obj.getClass().getAnnotation(XmlRootElement.class);
if (xmlRoot == null) {
return new JAXBElement(new QName(obj.getClass().getCanonicalName()), obj.getClass(), obj);
}
}
return obj;
}
private static Marshaller createMarshaller(JAXBContext jc, Object obj, Boolean formatted) throws JAXBException {
Marshaller m = jc.createMarshaller();
m.setProperty(Marshaller.JAXB_FORMATTED_OUTPUT, formatted);
return m;
}
public static T deSerialize(Class clazz, StreamSource source) throws JAXBException {
JAXBContext jc = JAXBContext.newInstance(clazz);
Unmarshaller u = jc.createUnmarshaller();
JAXBElement x = u.unmarshal(source, clazz);
return x.getValue();
}
public T deSerialize(StreamSource source) throws JAXBException {
Unmarshaller u = jc.createUnmarshaller();
JAXBElement x = u.unmarshal(source, clazz);
return x.getValue();
}
public static String serialize(Object obj) throws JAXBException {
Marshaller m = createMarshaller(JAXBContext.newInstance(obj.getClass()), obj, Boolean.TRUE);
StringWriter sw = new StringWriter(1024);
m.marshal(wrap(obj), sw);
return sw.toString();
}
public String serialize(Object obj, boolean formatted) throws JAXBException {
Marshaller m = createMarshaller(jc, obj, formatted);
StringWriter sw = new StringWriter(1024);
m.marshal(wrap(obj), sw);
return sw.toString();
}
public static String serializeUnFormatted(Object obj) throws JAXBException {
Marshaller m = createMarshaller(JAXBContext.newInstance(obj.getClass()), obj, Boolean.FALSE);
StringWriter sw = new StringWriter(1024);
m.marshal(wrap(obj), sw);
return sw.toString();
}
public static void serialize(Object obj, File file) throws JAXBException {
Marshaller m = createMarshaller(JAXBContext.newInstance(obj.getClass()), obj, Boolean.TRUE);
m.marshal(wrap(obj), file);
}
public static T deSerialize(Class clazz, InputStream in) throws JAXBException, IOException {
return deSerialize(clazz, new StreamSource(in));
}
public static T deSerialize(Class clazz, File file) throws JAXBException, IOException {
FileInputStream fis = new FileInputStream(file);
try {
return deSerialize(clazz, fis);
} finally {
IoTools.closeConsumeException(fis);
}
}
public static T deSerialize(Class clazz, String data) throws JAXBException {
return deSerialize(clazz, new StreamSource(new StringReader(data)));
}
@SuppressWarnings("unchecked")
public static T clone(T object) throws JAXBException {
return deSerialize((Class) object.getClass(), serialize(object));
}
// Configuration
public static File getFileLocation(Class> clazz) {
String configLocation = System.getProperty("com.sap.it.op.config");
return new File(configLocation, clazz.getCanonicalName() + ".xml");
}
public static boolean saveFileConfig(Object obj) {
try {
Marshaller m = createMarshaller(JAXBContext.newInstance(obj.getClass()), obj, Boolean.TRUE);
m.marshal(wrap(obj), getFileLocation(obj.getClass()));
return true;
} catch (JAXBException e) {
return false;
}
}
public static T loadFileConfig(Class clazz) {
File file = getFileLocation(clazz);
if (file.exists()) {
try {
return deSerialize(clazz, new StreamSource(file));
} catch (JAXBException e) {
return null;
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy