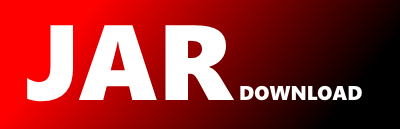
com.sap.cloud.sdk.service.prov.v4.util.ChangeSetUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of odatav4-prov Show documentation
Show all versions of odatav4-prov Show documentation
SAP Cloud Platform SDK for service development
/*******************************************************************************
* (c) 201X SAP SE or an SAP affiliate company. All rights reserved.
******************************************************************************/
package com.sap.cloud.sdk.service.prov.v4.util;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.List;
import java.util.Locale;
import org.apache.olingo.commons.api.http.HttpStatusCode;
import org.apache.olingo.server.api.ODataApplicationException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.sap.cloud.sdk.service.prov.annotation.repository.AnnotatedClassMethod;
import com.sap.cloud.sdk.service.prov.annotation.repository.AnnotationRepository;
import com.sap.cloud.sdk.service.prov.api.ChangeSetHandler;
import com.sap.cloud.sdk.service.prov.api.operations.ChangeSet;
public class ChangeSetUtil {
private static Logger log = LoggerFactory.getLogger(ChangeSetUtil.class);
private static final String CHANGESET = "ChangeSet";
private static final String GENERIC_ENDUSER_ERROR = "Internal Server Error; check log for details";
private ChangeSetHandler changeSet;
String serviceName;
public ChangeSetUtil(ChangeSetHandler handler,String serviceName){
this.changeSet = handler;this.serviceName=serviceName;
}
public void invokeChangeSetOperation() throws ODataApplicationException {
if (log.isDebugEnabled()) {
log.debug("Find method name for annoted operation: " + CHANGESET + " service name: " + serviceName);
}
boolean isDuplicate = false;
boolean isPresent = false;
List> list = AnnotationRepository.getInstance().getClassMethodListForAnnotation(CHANGESET);
AnnotatedClassMethod> annotatedMethodToBeInvoked = null;
if (list != null) {
Method method = null;
boolean isDefaultPresent = false;
String annotOperServiceName = null;
ChangeSet changeSetAnno = null;
for (AnnotatedClassMethod> annotatedClassMethod : list) {
method = annotatedClassMethod.getMethod();
changeSetAnno = method.getAnnotation(ChangeSet.class);
annotOperServiceName = changeSetAnno.serviceName();
if (annotOperServiceName.equals("") && !isPresent) {
if (isDefaultPresent) {
isDuplicate = true;
}
isDefaultPresent =true;
annotatedMethodToBeInvoked = annotatedClassMethod;
log.debug("Found default annoted method: " + method.getName() + " for operation " + CHANGESET);
}
if (annotOperServiceName.equals(serviceName)) {
if (isPresent) {
log.error("Duplicate custom method "+ method.getName() + " found for annoted operation: " + CHANGESET + " service name: " + serviceName);
throw new ODataApplicationException(GENERIC_ENDUSER_ERROR,
HttpStatusCode.INTERNAL_SERVER_ERROR.getStatusCode(), Locale.ENGLISH);
}
isPresent = true;
annotatedMethodToBeInvoked = annotatedClassMethod;
log.debug("Found annoted method: " + method.getName() + " for operation: " + CHANGESET + " for service name: " + serviceName);
}
}
}
if (!isPresent && isDuplicate) {
log.error("Duplicate custom method found for annoted operation: " + CHANGESET + " service name: " + serviceName);
throw new ODataApplicationException(GENERIC_ENDUSER_ERROR,
HttpStatusCode.INTERNAL_SERVER_ERROR.getStatusCode(), Locale.ENGLISH);
}
if (annotatedMethodToBeInvoked == null) {
log.error("No match found for annoted operation: " + CHANGESET + " service name: " + serviceName);
throw new ODataApplicationException("Method not implemented",
HttpStatusCode.NOT_IMPLEMENTED.getStatusCode(), Locale.ENGLISH);
}
try {
Class clazz = (Class)annotatedMethodToBeInvoked.getUserClass();
Method method = annotatedMethodToBeInvoked.getMethod();
method.invoke(clazz.newInstance(), changeSet);
} catch (IllegalAccessException | InvocationTargetException | InstantiationException e) {
log.error(e.getMessage());
throw new ODataApplicationException(e.getMessage(),
HttpStatusCode.NOT_IMPLEMENTED.getStatusCode(), Locale.ENGLISH, e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy