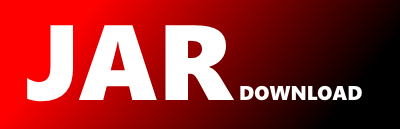
com.sap.cloud.sdk.hana.connectivity.handler.CDSDataSourceHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of hana-connectivity-cds Show documentation
Show all versions of hana-connectivity-cds Show documentation
SAP Cloud Platform SDK for service development
/*******************************************************************************
* (c) 201X SAP SE or an SAP affiliate company. All rights reserved.
******************************************************************************/
package com.sap.cloud.sdk.hana.connectivity.handler;
import java.sql.Connection;
import java.sql.SQLException;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.sap.cloud.sdk.hana.connectivity.cds.CDSException;
import com.sap.cloud.sdk.service.prov.rt.cds.CDSHandler;
/**
* API used by CDS Component.
*/
public abstract class CDSDataSourceHandler extends CDSHandler {
private static Logger log = LoggerFactory.getLogger(CDSDataSourceHandler.class);
Connection conn;
CDSDataSourceHandler(Connection conn, String ns) {
super(conn, ns);
this.conn = conn;
}
/**
* Start a transaction
* @return - true if start transaction is successful
* @throws CDSException - throws CDS exception
*/
public boolean startTransaction() throws CDSException {
try {
conn.setAutoCommit(false);
return true;
} catch (SQLException e) {
log.error("Exception while starting Transaction: " + e.getMessage(), e);
throw new CDSException(null, getExceptionCause(e));
}
}
/**
* Commit a transaction
* @return - true if commit is successful
* @throws CDSException - throws CDS exception
*/
public boolean commit() throws CDSException {
try {
conn.commit();
conn.setAutoCommit(true);
return true;
} catch (SQLException e) {
log.error("Exception while executing commit: " + e.getMessage(), e);
throw new CDSException(null, getExceptionCause(e));
}
}
/**
* Rollback a transaction
* @return - true if rollback is successful
* @throws CDSException - throws CDS exception
*/
public boolean rollback() throws CDSException {
try {
conn.rollback();
conn.setAutoCommit(true);
return true;
} catch (SQLException e) {
log.error("Exception while executing rollback: " + e.getMessage(), e);
throw new CDSException(null, getExceptionCause(e));
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy