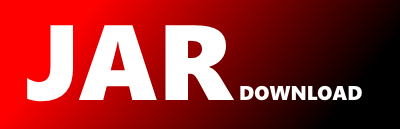
com.sap.xs.hdb.options.ConnectionPropertiesBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xs-hdb-conn-options Show documentation
Show all versions of xs-hdb-conn-options Show documentation
Use this library to handle SAP HANA DB connection options
package com.sap.xs.hdb.options;
import static java.lang.String.format;
import static java.util.stream.Collectors.joining;
import java.util.ArrayList;
import java.util.List;
import java.util.Properties;
import java.util.function.Consumer;
public class ConnectionPropertiesBuilder {
protected final List> propertyModifiers = new ArrayList<>();
/**
* Sets the autocommit connection property. For more information @see
* JDBC
* Connection Properties
*
* @param autoCommit
* autoCommit
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withAutoCommit(boolean autoCommit) {
withProperty("autocommit", Boolean.toString(autoCommit));
return this;
}
/**
* Sets the closeHandlesByCleaner connection property. For more
* information @see JDBC
* Connection Properties
*
* @param closeHandlesByCleaner
* closeHandlesByCleaner
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withCloseHandlesByCleaner(boolean closeHandlesByCleaner) {
withProperty("closeHandlesByCleaner", Boolean.toString(closeHandlesByCleaner));
return this;
}
/**
* Sets the closeHandlesOnFinalize connection property. For more
* information @see JDBC
* Connection Properties
*
* @param closeHandlesOnFinalize
* closeHandlesOnFinalize
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withCloseHandlesOnFinalize(boolean closeHandlesOnFinalize) {
withProperty("closeHandlesOnFinalize", Boolean.toString(closeHandlesOnFinalize));
return this;
}
/**
* Sets the communicationTimeout connection property. For more
* information @see JDBC
* Connection Properties
*
* @param communicationTimeout
* communicationTimeout
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withCommunicationTimeout(long communicationTimeout) {
withProperty("communicationTimeout", Long.toString(communicationTimeout));
return this;
}
/**
* Sets the compress connection property. For more information @see JDBC
* Connection Properties
*
* @param compress
* compress
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withCompress(boolean compress) {
withProperty("compress", Boolean.toString(compress));
return this;
}
/**
* Sets the connectTimeout connection property. For more information @see
* JDBC
* Connection Properties
*
* @param connectTimeout
* connectTimeout
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withConnectTimeout(long connectTimeout) {
withProperty("connectTimeout", Long.toString(connectTimeout));
return this;
}
/**
* Sets the currentSchema connection property. For more information @see
* JDBC
* Connection Properties
*
* @param currentSchema
* currentSchema
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withCurrentSchema(String currentSchema) {
withProperty("currentSchema", currentSchema);
return this;
}
/**
* Sets the databaseName connection property. For more information @see
* JDBC
* Connection Properties
*
* @param databaseName
* databaseName
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withDatabaseName(String databaseName) {
withProperty("databaseName", databaseName);
return this;
}
/**
* Sets the distribution connection property. For more information @see
* JDBC
* Connection Properties
*
* @param distribution
* distribution
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withDistribution(Distribution distribution) {
withProperty("distribution", distribution.name());
return this;
}
/**
* Sets the emptyTimestampIsNull connection property. For more
* information @see JDBC
* Connection Properties
*
* @param emptyTimestampIsNull
* emptyTimestampIsNull
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withEmptyTimestampIsNull(boolean emptyTimestampIsNull) {
withProperty("emptyTimestampIsNull", Boolean.toString(emptyTimestampIsNull));
return this;
}
/**
* Sets the ignoreTopology connection property. For more information @see
* JDBC
* Connection Properties
*
* @param ignoreTopology
* ignoreTopology
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withIgnoreTopology(int ignoreTopology) {
if (ignoreTopology != 0 && ignoreTopology != 1) {
throw new IllegalArgumentException("ignoreTopology can only be 0 or 1");
}
withProperty("ignoreTopology", Integer.toString(ignoreTopology));
return this;
}
/**
* Sets the instanceNumber connection property. For more information @see
* JDBC
* Connection Properties
*
* @param instanceNumber
* instanceNumber
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withInstanceNumber(int instanceNumber) {
withProperty("instanceNumber", Integer.toString(instanceNumber));
return this;
}
/**
* Sets the autoCommit connection property. For more information @see
* JDBC
* Connection Properties
*
* @param isolation
* isolation
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withIsolation(Isolation isolation) {
withProperty("isolation", isolation.name());
return this;
}
/**
* Sets the key connection property. For more information @see JDBC
* Connection Properties
*
* @param key
* key
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withKey(String key) {
withProperty("key", key);
return this;
}
/**
* Sets the latency connection property. For more information @see JDBC
* Connection Properties
*
* @param latency
* latency
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withLatency(long latency) {
withProperty("latency", Long.toString(latency));
return this;
}
/**
* Sets the locale connection property. For more information @see JDBC
* Connection Properties
*
* @param localeCode
* localeCode
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withLocale(String localeCode) {
withProperty("locale", localeCode);
return this;
}
/**
* Sets the autoCommit connection property. For more information @see
* JDBC
* Connection Properties
*
* @param packetSize
* packetSize
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withPacketsize(long packetSize) {
withProperty("packetsize", Long.toString(packetSize));
return this;
}
/**
* Sets the password connection property. For more information @see JDBC
* Connection Properties
*
* @param password
* password
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withPassword(String password) {
withProperty("password", password);
return this;
}
/**
* Sets the prefetch connection property. For more information @see JDBC
* Connection Properties
*
* @param prefetch
* prefetch
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withPrefetch(boolean prefetch) {
withProperty("prefetch", Boolean.toString(prefetch));
return this;
}
/**
* Sets the proxyHostname connection property. For more information @see
* JDBC
* Connection Properties
*
* @param proxyHostname
* proxyHostname
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withProxyHostname(String proxyHostname) {
withProperty("proxyHostname", proxyHostname);
return this;
}
/**
* Sets the proxyPassword connection property. For more information @see
* JDBC
* Connection Properties
*
* @param proxyPassword
* proxyPassword
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withProxyPassword(String proxyPassword) {
withProperty("proxyPassword", proxyPassword);
return this;
}
/**
* Sets the proxyPort connection property. For more information @see
* JDBC
* Connection Properties
*
* @param proxyPort
* proxyPort
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withProxyPort(int proxyPort) {
withProperty("proxyPort", Integer.toString(proxyPort));
return this;
}
/**
* Sets the proxyScpAccount connection property. For more information @see
* JDBC
* Connection Properties
*
* @param proxyScpAccount
* proxyScpAccount
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withProxyScpAccount(String proxyScpAccount) {
withProperty("proxyScpAccount", proxyScpAccount);
return this;
}
/**
* Sets the proxyUserName connection property. For more information @see
* JDBC
* Connection Properties
*
* @param proxyUserName
* proxyUserName
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withProxyUserName(String proxyUserName) {
withProperty("proxyUserName", proxyUserName);
return this;
}
/**
* Sets the readOnly connection property. For more information @see JDBC
* Connection Properties
*
* @param readOnly
* readOnly
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withReadOnly(boolean readOnly) {
withProperty("readOnly", Boolean.toString(readOnly));
return this;
}
/**
* Sets the reconnect connection property. For more information @see
* JDBC
* Connection Properties
*
* @param reconnect
* reconnect
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withReconnect(boolean reconnect) {
withProperty("reconnect", Boolean.toString(reconnect));
return this;
}
/**
* Sets the sessionVariable connection property. For more information @see
* JDBC
* Connection Properties
*
* @param key
* key
* @param value
* value
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withSessionVariable(String key, String value) {
withProperty("sessionVariable:" + key, value);
return this;
}
/**
* Sets the siteType connection property. For more information @see JDBC
* Connection Properties
*
* @param siteType
* siteType
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withSiteType(SiteType siteType) {
withProperty("siteType", siteType.name());
return this;
}
/**
* Sets the splitBatchCommands connection property. For more
* information @see JDBC
* Connection Properties
*
* @param splitBatchCommands
* splitBatchCommands
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withSplitBatchCommands(boolean splitBatchCommands) {
withProperty("splitBatchCommands", Boolean.toString(splitBatchCommands));
return this;
}
/**
* Sets the statementCacheSize connection property. For more
* information @see JDBC
* Connection Properties
*
* @param statementCacheSize
* statementCacheSize
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withStatementCacheSize(int statementCacheSize) {
withProperty("statementCacheSize", Integer.toString(statementCacheSize));
return this;
}
/**
* Sets the trace connection property. For more information @see JDBC
* Connection Properties
*
* @param traceFileName
* traceFileName
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withTrace(String traceFileName) {
withProperty("trace", traceFileName);
return this;
}
/**
* Sets the transactionalLobs connection property. For more information @see
* JDBC
* Connection Properties
*
* @param transactionalLobs
* transactionalLobs
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withTransactionalLobs(boolean transactionalLobs) {
withProperty("transactionalLobs", Boolean.toString(transactionalLobs));
return this;
}
/**
* Sets the user connection property. For more information @see JDBC
* Connection Properties
*
* @param user
* user
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withUser(String user) {
withProperty("user", user);
return this;
}
/**
* Sets the virtualHostName connection property. For more information @see
* JDBC
* Connection Properties
*
* @param virtualHostName
* virtualHostName
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withVirtualHostName(String virtualHostName) {
withProperty("virtualHostName", virtualHostName);
return this;
}
/**
* Sets the autoCommit connection property. For more information @see
* JDBC
* Connection Properties
*
* @param websocketURL
* websocketURL
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withWebsocketURL(String websocketURL) {
withProperty("websocketURL", websocketURL);
return this;
}
/**
* Sets the cseKeyStorePassword connection property. For more
* information @see JDBC
* Connection Properties
*
* @param cseKeyStorePassword
* cseKeyStorePassword
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withCseKeyStorePassword(String cseKeyStorePassword) {
withProperty("cseKeyStorePassword", cseKeyStorePassword);
return this;
}
/**
* Sets the encrypt connection property. For more information @see JDBC
* Connection Properties
*
* @param encrypt
* encrypt
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withEncrypt(boolean encrypt) {
withProperty("encrypt", Boolean.toString(encrypt));
return this;
}
/**
* Sets the hostNameInCertificate connection property. For more
* information @see JDBC
* Connection Properties
*
* @param hostname
* host name in certificate
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withHostNameInCertificate(String hostname) {
withProperty("hostNameInCertificate", hostname);
return this;
}
/**
* Sets the keyStore connection property. For more information @see JDBC
* Connection Properties
*
* @param keyStore
* keyStore
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withKeyStore(String keyStore) {
withProperty("keyStore", keyStore);
return this;
}
/**
* Sets the keyStorePassword connection property. For more information @see
* JDBC
* Connection Properties
*
* @param keyStorePassword
* keyStorePassword
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withKeyStorePassword(String keyStorePassword) {
withProperty("keyStorePassword", keyStorePassword);
return this;
}
/**
* Sets the keyStoreType connection property. For more information @see
* JDBC
* Connection Properties
*
* @param keyStoreType
* keyStoreType
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withKeyStoreType(String keyStoreType) {
withProperty("keyStoreType", keyStoreType);
return this;
}
/**
* Sets the sniHostname connection property. For more information @see
* JDBC
* Connection Properties
*
* @param sniHostname
* sniHostname
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withSNIHostname(String sniHostname) {
withProperty("sniHostname", sniHostname);
return this;
}
/**
* Sets the trustStore connection property. For more information @see
* JDBC
* Connection Properties
*
* @param trustStore
* trustStore
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withTrustStore(String trustStore) {
withProperty("trustStore", trustStore);
return this;
}
/**
* Sets the trustStorePassword connection property. For more
* information @see JDBC
* Connection Properties
*
* @param trustStorePassword
* trustStorePassword
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withTrustStorePassword(String trustStorePassword) {
withProperty("trustStorePassword", trustStorePassword);
return this;
}
/**
* Sets the trustStoreType connection property. For more information @see
* JDBC
* Connection Properties
*
* @param trustStoreType
* trustStoreType
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withTrustStoreType(String trustStoreType) {
withProperty("trustStoreType", trustStoreType);
return this;
}
/**
* Sets the validateCertificate connection property. For more
* information @see JDBC
* Connection Properties
*
* @param validateCertificate
* validateCertificate
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withValidateCertificate(boolean validateCertificate) {
withProperty("validateCertificate", Boolean.toString(validateCertificate));
return this;
}
/**
* Sets an arbitrary connection property. For a list of supported connection
* properties @see JDBC
* Connection Properties
*
* @param name
* name
* @param value
* value
* @return {@link ConnectionPropertiesBuilder}
*/
public ConnectionPropertiesBuilder withProperty(final String name, final String value) {
propertyModifiers.add(p -> p.setProperty(name, value));
return this;
}
/**
* @return connection options
*/
public Properties build() {
Properties connOptions = new Properties();
propertyModifiers.forEach(modifier -> modifier.accept(connOptions));
return connOptions;
}
/**
* Builds the property map and returns a String representation in the format
* key1=value1separator
key2=value2
*
* @param separator
* separator
* @return the connection properties map as a String
*/
public String buildString(String separator) {
return build().entrySet().stream().map(e -> format("%s=%s", e.getKey(), e.getValue()))
.collect(joining(separator));
}
public enum Distribution {
OFF, CONNECTION, STATEMENT, ALL
}
public enum Isolation {
TRANSACTION_READ_COMMITTED, TRANSACTION_REPEATABLE_READ, TRANSACTION_SERIALIZABLE
}
public enum SiteType {
PRIMARY, SECONDARY
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy