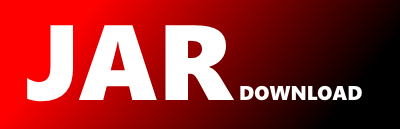
com.sap.xs.hdb.utils.DigestUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of xs-hdb-conn-options Show documentation
Show all versions of xs-hdb-conn-options Show documentation
Use this library to handle SAP HANA DB connection options
package com.sap.xs.hdb.utils;
import java.nio.charset.StandardCharsets;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public final class DigestUtils {
private static final char[] DIGITS_UPPER = { '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', 'A', 'B', 'C', 'D', 'E', 'F' };
private DigestUtils() {}
/**
* @param string
* string to encode
* @return the encoded string
* @throws NoSuchAlgorithmException
* NoSuchAlgorithmException
*/
public static char[] getSha256Hex(String string) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hash = digest.digest(string.getBytes(StandardCharsets.UTF_8));
return encodeHex(hash);
}
/**
* @param string
* to encode
* @return the encoded string
* @throws NoSuchAlgorithmException
* NoSuchAlgorithmException
*/
public static String getSha256HexString(String string) throws NoSuchAlgorithmException {
return new String(getSha256Hex(string));
}
private static char[] encodeHex(final byte[] data) {
final int l = data.length;
final char[] out = new char[l << 1];
// two characters form the hex value.
int j = 0;
for (int i = 0; i < l; i++) {
out[j++] = DIGITS_UPPER[(0xF0 & data[i]) >>> 4];
out[j++] = DIGITS_UPPER[0x0F & data[i]];
}
return out;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy