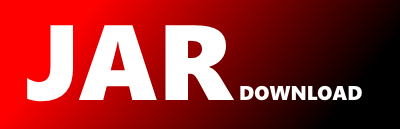
com.sap.cloud.spring.boot.mt.cds.SingleTenantConfig Maven / Gradle / Ivy
/******************************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
******************************************************************************/
package com.sap.cloud.spring.boot.mt.cds;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
import java.util.function.Supplier;
import javax.sql.DataSource;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Conditional;
import org.springframework.context.annotation.Configuration;
import com.sap.cds.CdsDataStoreConnector;
import com.sap.cds.reflect.CdsModel;
import com.sap.cds.reflect.impl.CdsModelReader;
@Configuration("comSapMtSingleTenantConfig")
@ConfigurationProperties(Const.CSN_MODEL_PREFIX)
@Conditional(SingleTenantCondition.class)
public class SingleTenantConfig {
private static final String CSN_JSON = "csn.json";
private String path = CSN_JSON;
public CdsModel getCdsModel() throws IOException {
try (InputStream is = SingleTenantConfig.class.getClassLoader().getResourceAsStream(path)) {
if (is == null) {
throw new IOException("Model " + path + " not found");
}
return CdsModelReader.read(is);
}
}
@Bean("comSapMtCdsDataStoreConnector")
public CdsDataStoreConnector cdsDataStoreConnector(DataSource ds, CdsTransactionManager transactionManager) throws IOException {
return CdsDataStoreConnector.createJdbcConnector(getCdsModel(), transactionManager).datasource(getDataSourceProxy(ds)).build();
}
private DataSource getDataSourceProxy(DataSource ds) {
// The data source proxy needs a new connection invocation handler for each getConnection call. This is
// achieved by calling this supplier.
Supplier connectionInvocationHandlerSupplier = () -> new ConnectionInvocationHandler(ds);
InvocationHandler dataSourceInvocationHandler = new DataSourceInvocationHandler(ds, connectionInvocationHandlerSupplier);
return (javax.sql.DataSource) Proxy.newProxyInstance(SingleTenantConfig.class.getClassLoader(),
new Class>[]{javax.sql.DataSource.class},
dataSourceInvocationHandler);
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy