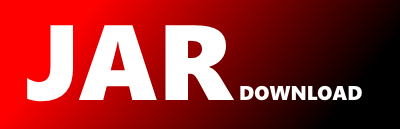
com.sap.cloud.spring.boot.mt.cds.MultiTenantSingleModelConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-boot-cds4j Show documentation
Show all versions of spring-boot-cds4j Show documentation
Enables cds4j for Spring Boot
The newest version!
/******************************************************************************
* © 2020 SAP SE or an SAP affiliate company. All rights reserved. *
******************************************************************************/
package com.sap.cloud.spring.boot.mt.cds;
import com.sap.cds.CdsDataStoreConnector;
import com.sap.cds.mt.CdsDataStoreLookup;
import com.sap.cds.mt.TenantAwareCdsDataStoreConnector;
import com.sap.cds.mtx.CdsDataStoreConnectorCreator;
import com.sap.cds.mtx.MetaDataAccessor;
import com.sap.cds.mtx.impl.CacheParams;
import com.sap.cds.mtx.impl.CdsDataStoreConnectorCreatorImpl;
import com.sap.cds.mtx.impl.MetaDataAccessorSingleModelImpl;
import com.sap.cds.mtx.impl.ModelOutDatedChecker;
import com.sap.cloud.mt.runtime.TenantAwareDataSource;
import com.sap.cloud.mt.runtime.TenantProvider;
import com.sap.cloud.spring.boot.mt.lib.InvocationHandlerForTenant;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Conditional;
import org.springframework.context.annotation.Configuration;
import org.springframework.util.Assert;
import javax.sql.DataSource;
import java.io.IOException;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Proxy;
import java.util.concurrent.TimeUnit;
import java.util.function.Supplier;
@Configuration("comSapMtMultiTenantSingleModelConfig")
@Conditional(MultiTenantSingleModelCondition.class)
@ConfigurationProperties(Const.CSN_MODEL_PREFIX)
public class MultiTenantSingleModelConfig {
private static final String CSN_JSON = "csn.json";
private String path = CSN_JSON;
@Bean("comSapMtMetaDataAccessor")
MetaDataAccessor
© 2015 - 2025 Weber Informatics LLC | Privacy Policy