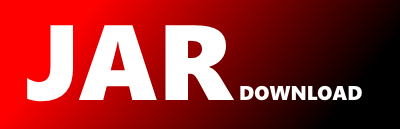
com.sap.cloud.yaas.servicesdk.auditbase.event.PersonalDataChangeAuditEvent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of service-sdk-audit-base Show documentation
Show all versions of service-sdk-audit-base Show documentation
Contains base utility classes for audit event logging
The newest version!
/*
* © 2017 SAP SE or an SAP affiliate company.
* All rights reserved.
* Please see http://www.sap.com/corporate-en/legal/copyright/index.epx for additional trademark information and
* notices.
*/
package com.sap.cloud.yaas.servicesdk.auditbase.event;
import javax.xml.bind.annotation.XmlRootElement;
import com.fasterxml.jackson.annotation.JsonAutoDetect;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.sap.cloud.yaas.servicesdk.auditbase.validation.Mandatory;
import com.sap.cloud.yaas.servicesdk.auditbase.validation.Validated;
import java.util.ArrayList;
import java.util.Collections;
import java.util.Date;
import java.util.List;
/**
* Changes to personal data as defined in the data protection agreements. For example: change of billing address of a
* customer, change of profile picture of a Yaas user.
*/
@XmlRootElement
@JsonAutoDetect(isGetterVisibility = JsonAutoDetect.Visibility.NONE, getterVisibility = JsonAutoDetect.Visibility.NONE, //
setterVisibility = JsonAutoDetect.Visibility.NONE, creatorVisibility = JsonAutoDetect.Visibility.NONE, //
fieldVisibility = JsonAutoDetect.Visibility.NONE)
@Validated
public final class PersonalDataChangeAuditEvent extends AuditEvent
{
/**
* The type of the event as used in Audit Ingestion Service.
*/
private static final String TYPE = "personal-data-changes";
@Mandatory
@JsonProperty(value = "attributes")
private final List attributes;
@Mandatory
@JsonProperty(value = "objectId")
private final String objectId;
@Mandatory
@JsonProperty(value = "objectType")
private final String objectType;
@Mandatory
@JsonProperty(value = "dataSubjectId")
private final String dataSubjectId;
@Mandatory
@JsonProperty(value = "dataSubjectType")
private final String dataSubjectType;
// CHECKSTYLE IGNORE ParameterNumberCheck NEXT 10
@SuppressWarnings("PMD.ExcessiveParameterList")
private PersonalDataChangeAuditEvent(final String serviceBasePath, final String serviceRegion, final String source,
final SourceType sourceType, final String userId, final Date timestamp, final List attributes,
final String objectId, final String objectType, final String dataSubjectId, final String dataSubjectType)
{
super(serviceBasePath, serviceRegion, source, sourceType, userId, timestamp);
this.attributes = attributes;
this.dataSubjectId = dataSubjectId;
this.dataSubjectType = dataSubjectType;
this.objectId = objectId;
this.objectType = objectType;
}
/**
* Creates a builder for personal data change audit event, see {@link AuditEventBuilderFactory#personalDataChange()}.
*
* @return a new instance of {@link PersonalDataChangeAuditEventBuilder}
*/
public static PersonalDataChangeAuditEventBuilder builder()
{
return new PersonalDataChangeAuditEventBuilder();
}
@Override
public String getType()
{
return TYPE;
}
public List getPersonalDataChangeAttrs()
{
return Collections.unmodifiableList(attributes);
}
/**
* See {@link PersonalDataChangeAuditEventBuilder#objectId(String)}.
*
* @return the object id
*/
public String getObjectId()
{
return objectId;
}
/**
* See {@link PersonalDataChangeAuditEventBuilder#objectType(String)}.
*
* @return the object type
*/
public String getObjectType()
{
return objectType;
}
/**
* See {@link PersonalDataChangeAuditEventBuilder#dataSubjectId(String)}.
*
* @return the data subject id
*/
public String getDataSubjectId()
{
return dataSubjectId;
}
/**
* See {@link PersonalDataChangeAuditEventBuilder#dataSubjectType(String)}.
*
* @return the data subject type
*/
public String getDataSubjectType()
{
return dataSubjectType;
}
@Override
public String toString()
{
return String
.format("%s, objectId: '%s', objectType: '%s', attributes: '%s'", super.toString(), objectId, objectType, attributes);
}
/**
* Builder for {@link PersonalDataChangeAuditEvent}.
*/
@SuppressWarnings("PMD.AvoidFieldNameMatchingMethodName")
public static class PersonalDataChangeAuditEventBuilder
extends AbstractAuditEventBuilder
{
private List personalDataChangeAttrs;
private String objectId;
private String objectType;
private String dataSubjectId;
private String dataSubjectType;
/**
* The identifier of the object containing the personal data that is
* being changed. The triplet (serviceBasePath, objectType, objectId) should
* uniquely identify an object within the client owner (tenant) scope."
* Could by a YaaSa Resource Name, for example:
* "urn:yaas:hybris:product:product:tempfbf21f6;57bd96ab9ef6c9001d9b73e4".
*
* @param newObjectId the object identifier
* @return {@code this}, for chaining
*/
public PersonalDataChangeAuditEventBuilder objectId(final String newObjectId)
{
if (newObjectId != null)
{
this.objectId = newObjectId;
}
return this;
}
/**
* The type of the object containing the personal data as defined by the
* service. The triplet (serviceBasePath, objectType, objectId) should
* uniquely identify an object within the client owner (tenant) scope.
*
* @param newObjectType the type of the object
* @return {@code this}, for chaining
*/
public PersonalDataChangeAuditEventBuilder objectType(final String newObjectType)
{
if (newObjectType != null)
{
this.objectType = newObjectType;
}
return this;
}
/**
* The identifier of the individual to which the personal data relates
* to.
*
* @param newDataSubjectId the ID of the personal data owner
* @return {@code this}, for chaining
*/
public PersonalDataChangeAuditEventBuilder dataSubjectId(final String newDataSubjectId)
{
if (newDataSubjectId != null)
{
this.dataSubjectId = newDataSubjectId;
}
return this;
}
/**
* The provider that manages the individual identified by the
* dataSubjectId. (Example: yaas-account, caas-customer).
*
* @param newDataSubjectType the data subject type
* @return {@code this}, for chaining
*/
public PersonalDataChangeAuditEventBuilder dataSubjectType(final String newDataSubjectType)
{
if (newDataSubjectType != null)
{
this.dataSubjectType = newDataSubjectType;
}
return this;
}
/**
* Adds information about the changed attributes of the object and associated old and new values.
*
* @param name attribute name; for nested values use dots to separate the path
* @param oldValue the previous value of the attribute if available; should never contain passwords
* @param newValue the new value of the attribute; should never contain passwords
* @param operation the operation made to the attribute
* @return {@code this}, for chaining
*/
public PersonalDataChangeAuditEventBuilder addPersonalDataChange(final String name, final String oldValue,
final String newValue, final AuditEventOperation operation)
{
if (personalDataChangeAttrs == null)
{
personalDataChangeAttrs = new ArrayList<>();
}
final AuditEventAttribute attr = new AuditEventAttribute();
attr.setName(name);
attr.setValue(newValue);
attr.setOldValue(oldValue);
attr.setOperation(operation);
personalDataChangeAttrs.add(attr);
return this;
}
@Override
public PersonalDataChangeAuditEvent build()
{
return new PersonalDataChangeAuditEvent(resolveServiceBasePath(), resolveServiceRegion(), resolveSource(),
resolveSourceType(), resolveUserId(),
resolveTimestamp(), personalDataChangeAttrs, objectId, objectType, dataSubjectId, dataSubjectType);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy