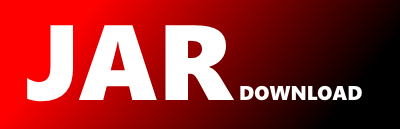
com.sap.cloud.yaas.servicesdk.authorization.AccessToken Maven / Gradle / Ivy
/*
* © 2016 SAP SE or an SAP affiliate company.
* All rights reserved.
* Please see http://www.sap.com/corporate-en/legal/copyright/index.epx for additional trademark information and
* notices.
*/
package com.sap.cloud.yaas.servicesdk.authorization;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.List;
import java.util.concurrent.TimeUnit;
import com.google.common.base.Joiner;
/**
* Represents an OAuth 2.0 Access Token and associated information, such as granted scopes and
* expiration.
*
* Instances of this class are immutable.
*
* @see http://tools.ietf.org/html/rfc6749#section-1.4
*/
public class AccessToken
{
private final String value;
private final Integer expiresIn;
private final String type;
private final List scopes;
private final long acquiredAt;
/**
* Creates a new token instance based on given field values.
*
* @param value The string value that represents the token. Opaque to the client, but passed to subsequent requests
* to a YaaS service.
* @param acquiredAt a timestamp that denotes the time when the token was acquired
* @param expiresIn the duration in seconds in which the token will expire, if {@code null} or negative it will
* expire immediately
* @param type the type of the access token, like 'Bearer', as specified by OAuth 2.0
* (see http://tools.ietf.org/html/rfc6749#section-7.1 for details)
* @param scopes list of scopes the token is granted for
*/
public AccessToken(
final String value,
final long acquiredAt,
final Integer expiresIn,
final String type,
final Collection scopes)
{
this.value = value;
this.acquiredAt = acquiredAt;
this.expiresIn = expiresIn;
this.type = type;
if (scopes == null)
{
this.scopes = null;
}
else
{
this.scopes = Collections.unmodifiableList(new ArrayList<>(scopes));
}
}
@Override
public String toString()
{
return AccessToken.class.getSimpleName()
+ "[type=" + type
+ (scopes != null ? ", scope=" + Joiner.on(' ').join(scopes) : "")
+ ", expiresIn=" + expiresIn
+ ", acquiredAt=" + acquiredAt + "]";
}
/**
* Converts the token to a String format that is suitable for use in an {@code Authorization} header.
*
* @return the value for an {@code Authorization} header
* @see http://tools.ietf.org/html/rfc6749#section-7
*/
public String toAuthorizationHeaderValue()
{
return type + " " + value;
}
/**
* Checks if the token has passed it's expiration period.
*
* @return true if the difference between creation time and current time is bigger than expiration period
*/
public boolean isExpired()
{
if (expiresIn == null || expiresIn <= 0)
{
return true;
}
final long diff = System.currentTimeMillis() - acquiredAt;
return diff >= TimeUnit.SECONDS.toMillis(expiresIn);
}
public String getValue()
{
return value;
}
public long getAcquiredAt()
{
return acquiredAt;
}
public Integer getExpiresIn()
{
return expiresIn;
}
public String getType()
{
return type;
}
public List getScopes()
{
return scopes;
}
}