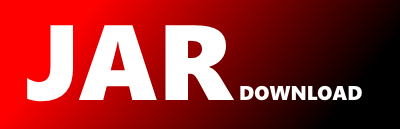
com.sap.cloud.yaas.servicesdk.springboot.clients.jersey.OAuth2ClientProperties Maven / Gradle / Ivy
/*
* © 2017 SAP SE or an SAP affiliate company.
* All rights reserved.
* Please see http://www.sap.com/corporate-en/legal/copyright/index.epx for additional trademark information and
* notices.
*/
package com.sap.cloud.yaas.servicesdk.springboot.clients.jersey;
import com.sap.cloud.yaas.servicesdk.authorization.AuthorizationScope;
import org.springframework.boot.context.properties.ConfigurationProperties;
import java.net.URI;
/**
* Properties used to configure an OAuth 2.0 client that uses the Client Credentials grant to obtain Access Tokens.
* Those can be used to make authorized requests to other services.
*/
@ConfigurationProperties(prefix = "yaas.clients.oauth2")
public class OAuth2ClientProperties
{
/**
* The URI of the Token Endpoint of the OAuth 2.0 Authorization server to use.
* Defaults to the Token Endpoint of the YaaS Oauth2 service, currently in v1.
*/
private URI tokenEndpointUri = URI.create("https://api.stage.yaas.io/hybris/oauth2/v1/token");
/**
* The OAuth 2.0 client id to use for authentication against the Authorization server.
* Leave it empty in order to disable OAuth 2.0 authorization.
*/
private String clientId;
/**
* The OAuth 2.0 client secret to use for authentication against the Authorization server.
* Note: It is strongly advised to externalize this configuration property (e.g. by means of CLI arguments or
* environment variables) and not make it part of the executable of an application (e.g. inside an
* application.properties file).
*/
private String clientSecret;
/**
* Should the OAuth 2.0 validate whether the Authorization server granted all the scopes that have been requested?
* Set to false to silently ignore situations when the Authorization server only granted part of the requested
* scopes.
*/
private boolean scopeValidationEnabled = true;
/**
* A list of OAuth 2.0 scopes that a Jersey client will obtain, when nothing else is specified for the particular
* request.
*/
private AuthorizationScope defaultScope;
/**
* Number of retries that a Jersey client should attempt in case of authorization failures (HTTP status 401) when
* using a previously obtained Access Token.
* That usually happens if an Access Token expires earlier than previously announced by the Authorization server.
* The default of 1 should be suitable for almost all scenarios. Set to 0 in order to disable automatic retries.
*/
private int tokenRequestRetries = 1;
public URI getTokenEndpointUri()
{
return tokenEndpointUri;
}
public void setTokenEndpointUri(final URI tokenEndpointUri)
{
this.tokenEndpointUri = tokenEndpointUri;
}
public String getClientId()
{
return clientId;
}
public void setClientId(final String clientId)
{
this.clientId = clientId;
}
public String getClientSecret()
{
return clientSecret;
}
public void setClientSecret(final String clientSecret)
{
this.clientSecret = clientSecret;
}
public Boolean getScopeValidationEnabled()
{
return scopeValidationEnabled;
}
public void setScopeValidationEnabled(final Boolean scopeValidationEnabled)
{
this.scopeValidationEnabled = scopeValidationEnabled;
}
public AuthorizationScope getDefaultScope()
{
return defaultScope;
}
public void setDefaultScope(final AuthorizationScope defaultScope)
{
this.defaultScope = defaultScope;
}
public Integer getTokenRequestRetries()
{
return tokenRequestRetries;
}
public void setTokenRequestRetries(final Integer tokenRequestRetries)
{
this.tokenRequestRetries = tokenRequestRetries;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy