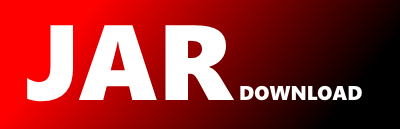
com.sap.cloud.yaas.servicesdk.springboot.jersey.JerseyServiceProperties Maven / Gradle / Ivy
/*
* © 2017 SAP SE or an SAP affiliate company.
* All rights reserved.
* Please see http://www.sap.com/corporate-en/legal/copyright/index.epx for additional trademark information and
* notices.
*/
package com.sap.cloud.yaas.servicesdk.springboot.jersey;
import javax.validation.Valid;
import javax.validation.constraints.Min;
import javax.validation.constraints.NotNull;
import org.springframework.boot.context.properties.ConfigurationProperties;
import java.util.HashMap;
import java.util.Map;
/**
* Properties used to configure a server-side Jersey ResourceConfiguration.
*/
@ConfigurationProperties(prefix = "yaas.service.jersey")
public class JerseyServiceProperties
{
/**
* String valued configuration properties to pass through to the server-side Jersey ResourceConfiguration.
* Properties given here will be prepended with "jersey.config", so Spring property "yaas.service.jersey.config.foo"
* maps to Jersey property "jersey.config.foo".
*/
@NotNull
private Map config = new HashMap<>();
/**
* Enable registration of a JAX-RS feature that registers a Jackson JSON ObjectMapper with Jersey.
* Will use the ObjectMapper provided by JacksonAutoConfiguration, if present on the classpath.
* Alternatively, a custom ObjectMapper defined in the Spring application context will be used.
*/
private boolean enableJsonFeature = true;
/**
* Enable registration of a JAX-RS feature that supports annotation based security based on YaaS haeders.
* See the SecurityFeature from the YaaS Service SDK for more information.
*/
private boolean enableSecurityFeature = true;
/**
* Enable registration of a JAX-RS feature that exposes a simple ping resource.
* The ping resource will be available at URI path "/ping" and will respond to GET requests with a status of to 200
* and a content body of "OK".
*/
private boolean enablePingResource = true;
/**
* Nested configuration properties that control logging of requests to the service, and the corresponding responses.
*/
@NotNull
@Valid
private RequestResponseLoggingProperties requestResponseLogging = new RequestResponseLoggingProperties();
public Map getConfig()
{
return config;
}
public void setConfig(final Map config)
{
this.config = config;
}
public boolean isEnableJsonFeature()
{
return enableJsonFeature;
}
public void setEnableJsonFeature(final boolean enableJsonFeature)
{
this.enableJsonFeature = enableJsonFeature;
}
public boolean isEnableSecurityFeature()
{
return enableSecurityFeature;
}
public void setEnableSecurityFeature(final boolean enableSecurityFeature)
{
this.enableSecurityFeature = enableSecurityFeature;
}
public boolean isEnablePingResource()
{
return enablePingResource;
}
public void setEnablePingResource(final boolean enablePingResource)
{
this.enablePingResource = enablePingResource;
}
public RequestResponseLoggingProperties getRequestResponseLogging()
{
return requestResponseLogging;
}
public void setRequestResponseLogging(final RequestResponseLoggingProperties requestResponseLogging)
{
this.requestResponseLogging = requestResponseLogging;
}
/**
* Nested configuration properties that control logging of requests and responses processed by Jersey.
*/
public static class RequestResponseLoggingProperties
{
/**
* The name of the Slf4j Logger to use for logging requests and responses.
* Set to the literal value "false" to disable request and response logging.
*/
@NotNull
private String loggerName = "";
/**
* The maximum number of bytes of both request and response entity to log.
* If set to zero, only headers will be logged.
*/
@Min(0)
private int maxEntitySize;
public String getLoggerName()
{
return loggerName;
}
public void setLoggerName(final String loggerName)
{
this.loggerName = loggerName;
}
public int getMaxEntitySize()
{
return maxEntitySize;
}
public void setMaxEntitySize(final int maxEntitySize)
{
this.maxEntitySize = maxEntitySize;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy