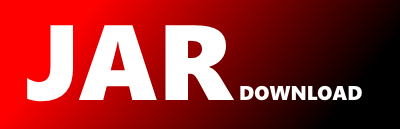
com.sap.cloud.yaas.servicesdk.springboot.jersey.NotFoundExceptionPassThroughMapper Maven / Gradle / Ivy
/*
* © 2017 SAP SE or an SAP affiliate company.
* All rights reserved.
* Please see http://www.sap.com/corporate-en/legal/copyright/index.epx for additional trademark information and
* notices.
*/
package com.sap.cloud.yaas.servicesdk.springboot.jersey;
import javax.annotation.Priority;
import javax.ws.rs.NotFoundException;
import javax.ws.rs.Produces;
import javax.ws.rs.core.MediaType;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.Response.Status;
import javax.ws.rs.ext.ExceptionMapper;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Serializes a {@link NotFoundException} to a 404 response with an empty body.
*
* This is useful, if further processing outside of JAX-RS is intended.
* In particular, when Jersey runs as a Filter and passes requests that it cannot handle itself up the Filter chain.
*
* @see org.glassfish.jersey.servlet.ServletProperties#FILTER_FORWARD_ON_404
*/
@Produces({MediaType.APPLICATION_JSON})
@Priority(NotFoundExceptionPassThroughMapper.THIS_PRIORITY)
public class NotFoundExceptionPassThroughMapper implements ExceptionMapper
{
/**
* Defines the priority of the {@link ExceptionMapper}.
*/
protected static final int THIS_PRIORITY = 10;
/** The logger used for this class. */
private static final Logger LOG = LoggerFactory.getLogger(NotFoundExceptionPassThroughMapper.class);
/**
* {@inheritDoc}
*
* This implementation creates an empty {@link Response}.
*/
@Override
public Response toResponse(final NotFoundException exception)
{
LOG.trace(
NotFoundException.class.getSimpleName()
+ " occured in JAX-RS;"
+ " not sending an error response body yet, so that other components get a chance to handle the request.",
exception);
return Response
.status(Status.NOT_FOUND)
.type(MediaType.APPLICATION_JSON_TYPE)
.build();
}
}