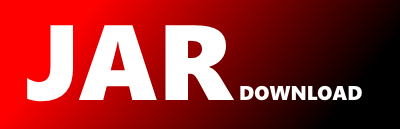
com.sap.hana.datalake.files.utils.http.RepeatableInputStreamEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sap-hdlfs Show documentation
Show all versions of sap-hdlfs Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting SAP HANA Data Lake Files.
// © 2023 SAP SE or an SAP affiliate company. All rights reserved.
package com.sap.hana.datalake.files.utils.http;
import com.sap.hana.datalake.files.classification.InterfaceAudience;
import org.apache.commons.io.IOUtils;
import org.apache.hadoop.thirdparty.com.google.common.base.Preconditions;
import org.apache.http.entity.InputStreamEntity;
import org.apache.http.util.Args;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
@InterfaceAudience.Private
public class RepeatableInputStreamEntity extends InputStreamEntity {
public RepeatableInputStreamEntity(final InputStream inStream) {
this(inStream, -1L);
}
public RepeatableInputStreamEntity(final InputStream inputStream, final long length) {
super(inputStream, length);
Preconditions.checkState(inputStream.markSupported());
Preconditions.checkState(length <= Integer.MAX_VALUE);
inputStream.mark(length == -1L ? Integer.MAX_VALUE : (int) length);
}
@Override
public boolean isRepeatable() {
return true;
}
@Override
public InputStream getContent() throws IOException {
final InputStream inputStream = super.getContent();
inputStream.reset();
return inputStream;
}
@Override
public void writeTo(final OutputStream outputStream) throws IOException {
Args.notNull(outputStream, "outputStream");
final InputStream inputStream = this.getContent();
final long length = this.getContentLength();
// Copy bytes to the OutputStream without closing the InputStream
IOUtils.copyLarge(inputStream, outputStream, /* inputOffset */ 0L, length);
}
}
// © 2023 SAP SE or an SAP affiliate company. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy