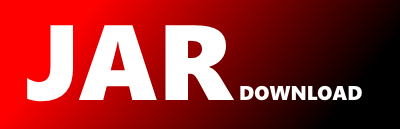
com.sap.hana.datalake.files.directaccess.gcs.GcsDirectAccessInputStream Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sap-hdlfs Show documentation
Show all versions of sap-hdlfs Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting SAP HANA Data Lake Files.
// © 2023 SAP SE or an SAP affiliate company. All rights reserved.
package com.sap.hana.datalake.files.directaccess.gcs;
import com.sap.hana.datalake.files.HdlfsFileSystemCapabilities;
import com.sap.hana.datalake.files.directaccess.BaseDirectAccessInputStream;
import com.sap.hana.datalake.files.directaccess.BaseSignedUrl;
import com.sap.hana.datalake.files.utils.http.HttpClientUtils;
import org.apache.hadoop.fs.Path;
import com.sap.hana.datalake.files.shaded.org.apache.hadoop.hdfs.web.WebHdfsFileSystem;
import org.apache.http.Header;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import java.io.IOException;
/* package-private */ class GcsDirectAccessInputStream extends BaseDirectAccessInputStream {
private static final String STORED_CONTENT_LENGTH_HEADER = "X-Goog-Stored-Content-Length";
private GcsSignedUrl signedUrl;
/* package-private */ GcsDirectAccessInputStream(final Path path,
final int chunkSize,
final int signedUrlExpirationSafetyMargin,
final WebHdfsFileSystem webHdfsFileSystem,
final HttpClient httpClient) {
super(path, chunkSize, signedUrlExpirationSafetyMargin, webHdfsFileSystem, httpClient);
}
@Override
protected synchronized BaseSignedUrl getSignedUrl() throws IOException {
if (this.signedUrl != null && !this.signedUrl.isExpired()) {
return this.signedUrl;
}
final HdlfsFileSystemCapabilities.DirectAccessResponse directAccessResponse = this.webHdfsFileSystem.openDirectAccess(this.path);
final HdlfsFileSystemCapabilities.GcsOpenDirectAccessProperties gcsDirectAccessProperties =
(HdlfsFileSystemCapabilities.GcsOpenDirectAccessProperties) directAccessResponse.getProperties();
this.signedUrl = GcsSignedUrl.from(gcsDirectAccessProperties.getSignedUrl(), this.signedUrlExpirationSafetyMargin);
return this.signedUrl;
}
@Override
protected long getBackendStoredContentLength(final HttpResponse response) {
return Long.parseLong(HttpClientUtils.getRequiredHeaderValue(response, STORED_CONTENT_LENGTH_HEADER));
}
}
// © 2023 SAP SE or an SAP affiliate company. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy