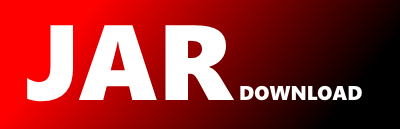
com.sap.hana.datalake.files.utils.HdlfsRetryUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sap-hdlfs Show documentation
Show all versions of sap-hdlfs Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting SAP HANA Data Lake Files.
// © 2022 SAP SE or an SAP affiliate company. All rights reserved.
package com.sap.hana.datalake.files.utils;
import org.apache.hadoop.io.retry.RetryPolicy;
import org.apache.hadoop.util.functional.CallableRaisingIOE;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
public class HdlfsRetryUtils {
private static final Logger LOG = LoggerFactory.getLogger(HdlfsRetryUtils.class);
public static T execWithRetry(final String operationTitle, final RetryPolicy retryPolicy,
final boolean idempotent, final CallableRaisingIOE operation) throws IOException {
if (retryPolicy != null) {
int retryCount = 0;
boolean shouldRetry;
IOException lastException;
do {
try {
LOG.debug("Executing [{}] - try [{}]", operationTitle, retryCount + 1);
return operation.apply();
} catch (final IOException ioEx) {
lastException = ioEx;
}
try {
final RetryPolicy.RetryAction retryAction = retryPolicy.shouldRetry(lastException, retryCount, /* failovers */ 0, idempotent);
shouldRetry = retryAction.action == RetryPolicy.RetryAction.RetryDecision.RETRY;
if (shouldRetry) {
LOG.warn("Error in [{}] during try [{}]; retrying in [{}] ms", operationTitle, retryCount + 1, retryAction.delayMillis, lastException);
Thread.sleep(retryAction.delayMillis);
}
retryCount++;
} catch (final InterruptedException intEx) {
lastException = new IOException("Thread interrupted while waiting to retry", intEx);
shouldRetry = false;
Thread.currentThread().interrupt();
} catch (final Exception ex) {
LOG.warn("Error in [{}] during retry processing", operationTitle, ex);
// Stop retrying and fail with last execution exception
shouldRetry = false;
}
} while (shouldRetry);
LOG.error("Failed to execute [{}]; gave up after [{}] retries", operationTitle, retryCount, lastException);
throw lastException;
} else {
LOG.warn("No retry policy configured; executing [{}] without retry", operationTitle);
return operation.apply();
}
}
}
// © 2022 SAP SE or an SAP affiliate company. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy