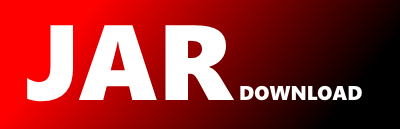
com.sap.hana.datalake.files.utils.filewatcher.DirectoryWatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sap-hdlfs Show documentation
Show all versions of sap-hdlfs Show documentation
An implementation of org.apache.hadoop.fs.FileSystem targeting SAP HANA Data Lake Files.
// © 2023-2024 SAP SE or an SAP affiliate company. All rights reserved.
package com.sap.hana.datalake.files.utils.filewatcher;
import com.sap.hana.datalake.files.classification.InterfaceAudience;
import org.slf4j.Logger;
import java.io.IOException;
import java.nio.file.FileSystems;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardWatchEventKinds;
import java.nio.file.WatchEvent;
import java.nio.file.WatchKey;
import java.nio.file.WatchService;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
@InterfaceAudience.Private
public class DirectoryWatcher implements Runnable {
@FunctionalInterface
public interface EventHandler {
void handleModifyEvent(final String fileName) throws FileWatcherException;
}
protected final EventHandler eventHandler;
private final List directoriesPath;
private final Logger log;
private final WatchService watchService;
public DirectoryWatcher(final String directoryPath, final Logger log, final EventHandler eventHandler) throws FileWatcherException {
this(Collections.singletonList(directoryPath), log, eventHandler);
}
public DirectoryWatcher(final List directoriesPath, final Logger log, final EventHandler eventHandler) throws FileWatcherException {
this.directoriesPath = directoriesPath;
this.log = log;
this.eventHandler = eventHandler;
this.watchService = this.setupWatchService();
}
@Override
public void run() {
this.watchModifyEvents();
}
protected WatchService setupWatchService() throws FileWatcherException {
try {
final WatchService watchService = FileSystems.getDefault().newWatchService();
final WatchEvent.Kind event = StandardWatchEventKinds.ENTRY_MODIFY;
for (final String directoryPath : this.directoriesPath) {
final Path dirPath = Paths.get(directoryPath).toAbsolutePath();
dirPath.register(watchService, event);
this.log.info("Registered the directory [{}] with the watch service for event [{}]", dirPath, event);
}
return watchService;
} catch (final IOException ex) {
final String errMsg = String.format("Error setting up the watch service for directories [%s]", this.directoriesPath);
this.log.error(errMsg, ex);
throw new FileWatcherException(errMsg);
}
}
private void watchModifyEvents() {
try {
WatchKey key;
while ((key = this.watchService.take()) != null) {
this.handleModifyEvent(key);
if (!key.reset()) {
break;
}
}
} catch (final InterruptedException ex) {
this.log.error("Thread interrupt while watching events", ex);
Thread.currentThread().interrupt();
}
}
private void handleModifyEvent(final WatchKey key) {
final Optional> modifyEvent = key.pollEvents()
.stream()
.filter(event -> event.kind() == StandardWatchEventKinds.ENTRY_MODIFY)
.findFirst();
if (!modifyEvent.isPresent()) {
return;
}
final String fileName = modifyEvent.get().context().toString();
try {
this.eventHandler.handleModifyEvent(fileName);
} catch (final FileWatcherException ex) {
this.log.error(String.format("Error in handling the modify event for the file: [%s]", fileName), ex);
}
}
}
// © 2023-2024 SAP SE or an SAP affiliate company. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy