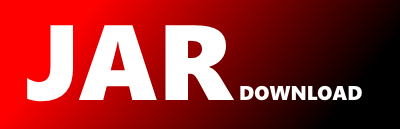
com.sap.ipe.ble.irp.utils.SecurityUtils Maven / Gradle / Ivy
package com.sap.ipe.ble.irp.utils;
import com.sap.ipe.ble.irp.handler.constants.Constants;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
/***
* SecurityUtils is used to check if response data has security vulnerability characters
*
*/
public class SecurityUtils {
private static final Pattern sanitizePattern = Pattern.compile(Constants.SANITISE_REGEX);
private static final Pattern vulnerablePattern = Pattern.compile(Constants.VULNERABLE_CHARAC_REGEX);
/***
* This method is the default constructor
*/
private SecurityUtils() {}
/**
* This method is used to perform input sanity test
* @param dataToBeSantized data to be sanitized
* @return if any match is found or not
*/
public static String santize(String dataToBeSantized) {
Matcher matcher = sanitizePattern.matcher(dataToBeSantized);
StringBuffer buf = new StringBuffer(dataToBeSantized.length());
while (matcher.find()) {
matcher.appendReplacement(buf, Matcher.quoteReplacement(" "));
}
matcher.appendTail(buf);
return buf.toString().replace("\n", "").replace("\r", "");
}
/***
* This method is used to validate the response for any unsafe characters passed
* @param dataToBeValidated data to be validated
* @return if any match is found or not
*/
public static boolean containUnsafeCharacters(String dataToBeValidated) {
Matcher matcher = vulnerablePattern.matcher(dataToBeValidated);
return matcher.find();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy