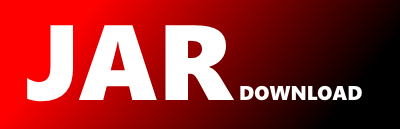
com.sap.psr.vulas.java.sign.ASTSignatureChange Maven / Gradle / Ivy
/**
* This file is part of Eclipse Steady.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* SPDX-License-Identifier: Apache-2.0
*
* Copyright (c) 2018 SAP SE or an SAP affiliate company. All rights reserved.
*/
package com.sap.psr.vulas.java.sign;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.fasterxml.jackson.databind.ser.std.StdSerializer;
import com.sap.psr.vulas.java.sign.gson.ASTSignatureChangeSerializer;
import com.sap.psr.vulas.shared.json.JacksonUtil;
import com.sap.psr.vulas.sign.Signature;
import com.sap.psr.vulas.sign.SignatureChange;
import ch.uzh.ifi.seal.changedistiller.distilling.Distiller;
import ch.uzh.ifi.seal.changedistiller.distilling.DistillerFactory;
import ch.uzh.ifi.seal.changedistiller.model.classifiers.java.JavaEntityType;
import ch.uzh.ifi.seal.changedistiller.model.entities.SourceCodeChange;
import ch.uzh.ifi.seal.changedistiller.model.entities.StructureEntityVersion;
import ch.uzh.ifi.seal.changedistiller.model.entities.Update;
import ch.uzh.ifi.seal.changedistiller.treedifferencing.Node;
/**
* ASTSignatureChange class.
*
*/
public class ASTSignatureChange extends DistillerUtil implements SignatureChange {
public static enum OperationType { Insert, Update, Move, Delete };
private Signature mDefSignature = null;
private Signature mFixSignature = null;
private Set listOfChanges = null;
private String mMethodName;
//TODO : (Something fishy here, needs an improvement - see the sourcecode of changedistiller)
//A StructureEntityVersion has all the SourceCodeChange's
private StructureEntityVersion structureEntity = null;
/**
* addChange.
*
* @param scc a {@link ch.uzh.ifi.seal.changedistiller.model.entities.SourceCodeChange} object.
*/
public void addChange(SourceCodeChange scc) {
this.listOfChanges.add(scc);
}
/**
* removeChange.
*
* @param scc a {@link ch.uzh.ifi.seal.changedistiller.model.entities.SourceCodeChange} object.
*/
public void removeChange(SourceCodeChange scc){
this.listOfChanges.remove(scc);
}
/**
* toSourceCodeChanges.
*
* @param _objects a {@link java.util.Set} object.
* @return a {@link java.util.Set} object.
*/
public static Set toSourceCodeChanges(Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy