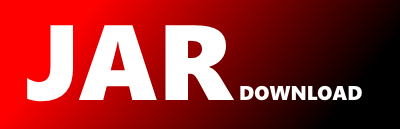
com.sappenin.utils.rest.v2.model.meta.impl.AbstractCollectionMeta Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-utils2 Show documentation
Show all versions of rest-utils2 Show documentation
Utilities, Helpers, and base-classes for assisting in server-side REST API implementations written in
Java.
The newest version!
/**
* Copyright (C) 2014 Sappenin Inc. ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except in compliance with
* the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License is distributed on
* an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the License for the
* specific language governing permissions and limitations under the License.
*/
package com.sappenin.utils.rest.v2.model.meta.impl;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.Setter;
import lombok.ToString;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.google.common.base.Optional;
import com.sappenin.utils.rest.v2.model.Link;
import com.sappenin.utils.rest.v2.model.meta.CollectionMeta;
/**
* A AbstractMeta object for storing read-only information about a Collection of resources.
*/
@JsonTypeName("meta")
@Getter
@Setter
@EqualsAndHashCode(callSuper = true)
@ToString
public abstract class AbstractCollectionMeta extends AbstractMeta implements CollectionMeta
{
private static final long serialVersionUID = 7323709012810641819L;
// An encoded value that represents the next starting index in the entire Collection of items to return. Omit to
// return results from the beginning of the Collection.
@JsonProperty("offset")
private final Optional optOffset;
// An array containing a listing of Objects of any object type. If used in combination with the url property, the
// items array can be used to provide a subset of the objects that may be found in the resource identified by the
// url. The maximum number of collection items N to return for a single request. Minimum value is 1. Max is 50.
// Default is 25.
@JsonProperty("limit")
private final Optional optLimit;
// An (Optional) total number as set by the server (this can be bigger than the size of the items array, which is
// merely a paged sub-collection).
@JsonProperty("totalItems")
private final Optional optTotalItems;
// TODO Links for previous, next, href, current.
/**
* Required-args Constructor
*
* @param selfLink A {@link Link} to this collection.
*/
public AbstractCollectionMeta(final Link selfLink)
{
super(selfLink);
this.optOffset = Optional.absent();
this.optLimit = OPT_DEFAULT_LIMIT;
this.optTotalItems = Optional.absent();
}
/**
* Required-args Constructor
*
* @param selfLink A {@link Link} to this collection.
* @param optOffset
* @param optLimit
* @param optTotalItems
*/
public AbstractCollectionMeta(@JsonProperty("@self") final Link selfLink,
@JsonProperty("offset") final Optional optOffset, @JsonProperty("limit") Optional optLimit,
@JsonProperty("optTotalItems") final Optional optTotalItems)
{
super(selfLink);
this.optOffset = optOffset;
this.optLimit = optLimit;
this.optTotalItems = optTotalItems;
}
/**
* Copy Constructor.
*
* @param collectionMeta
*/
public AbstractCollectionMeta(final CollectionMeta collectionMeta)
{
super(collectionMeta);
this.optOffset = collectionMeta.getOptOffset();
this.optLimit = collectionMeta.getOptLimit();
this.optTotalItems = collectionMeta.getOptTotalItems();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy