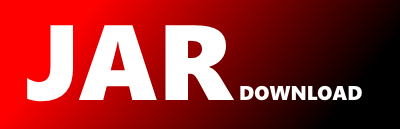
com.scalar.db.sql.statement.CreateTableStatement Maven / Gradle / Ivy
The newest version!
package com.scalar.db.sql.statement;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.collect.ImmutableSet;
import com.scalar.db.sql.ClusteringOrder;
import com.scalar.db.sql.DataType;
import com.scalar.db.sql.TableRef;
import java.util.Map.Entry;
import java.util.Objects;
import javax.annotation.concurrent.Immutable;
@Immutable
public class CreateTableStatement
implements DdlStatement, NamespaceNameOmittable {
public final TableRef table;
public final boolean ifNotExists;
public final ImmutableList columns;
public final ImmutableSet partitionKeyColumnNames;
public final ImmutableSet clusteringKeyColumnNames;
public final ImmutableMap clusteringOrders;
public final ImmutableMap options;
private CreateTableStatement(
TableRef table,
boolean ifNotExists,
ImmutableList columns,
ImmutableSet partitionKeyColumnNames,
ImmutableSet clusteringKeyColumnNames,
ImmutableMap clusteringOrders,
ImmutableMap options) {
this.table = table;
this.ifNotExists = ifNotExists;
this.columns = Objects.requireNonNull(columns);
this.partitionKeyColumnNames = Objects.requireNonNull(partitionKeyColumnNames);
this.clusteringKeyColumnNames = Objects.requireNonNull(clusteringKeyColumnNames);
this.clusteringOrders = Objects.requireNonNull(clusteringOrders);
this.options = Objects.requireNonNull(options);
}
@Override
public String toSql() {
StringBuilder builder = new StringBuilder("CREATE TABLE ");
if (ifNotExists) {
builder.append("IF NOT EXISTS ");
}
StatementUtils.appendTable(builder, table);
builder.append('(');
boolean first = true;
for (ColumnDef column : columns) {
if (!first) {
builder.append(',');
} else {
first = false;
}
StatementUtils.appendObjectName(builder, column.name);
builder.append(' ').append(column.dataType);
if (column.encrypted) {
builder.append(" ENCRYPTED");
}
}
builder.append(",PRIMARY KEY((");
StatementUtils.appendObjectNames(builder, partitionKeyColumnNames);
builder.append(')');
for (String clusteringKeyColumnName : clusteringKeyColumnNames) {
builder.append(',');
StatementUtils.appendObjectName(builder, clusteringKeyColumnName);
}
builder.append("))");
if (!clusteringOrders.isEmpty() || !options.isEmpty()) {
builder.append(" WITH ");
if (!clusteringOrders.isEmpty()) {
builder.append("CLUSTERING ORDER BY(");
first = true;
for (Entry entry : clusteringOrders.entrySet()) {
if (!first) {
builder.append(',');
} else {
first = false;
}
StatementUtils.appendObjectName(builder, entry.getKey());
builder.append(' ').append(entry.getValue());
}
builder.append(')');
}
if (!options.isEmpty()) {
if (!clusteringOrders.isEmpty()) {
builder.append(" AND ");
}
StatementUtils.appendOptions(builder, options);
}
}
return builder.toString();
}
@Override
public R accept(StatementVisitor visitor, C context) {
return visitor.visit(this, context);
}
@Override
public R accept(DdlStatementVisitor visitor, C context) {
return visitor.visit(this, context);
}
@Override
public boolean namespaceNameOmitted() {
return table.namespaceName == null;
}
@Override
public CreateTableStatement setNamespaceNameIfOmitted(String namespaceName) {
if (namespaceNameOmitted()) {
return create(
TableRef.of(namespaceName, table.tableName),
ifNotExists,
columns,
partitionKeyColumnNames,
clusteringKeyColumnNames,
clusteringOrders,
options);
}
return this;
}
@Override
public String toString() {
return toSql();
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof CreateTableStatement)) {
return false;
}
CreateTableStatement that = (CreateTableStatement) o;
return ifNotExists == that.ifNotExists
&& Objects.equals(table, that.table)
&& Objects.equals(columns, that.columns)
&& Objects.equals(partitionKeyColumnNames, that.partitionKeyColumnNames)
&& Objects.equals(clusteringKeyColumnNames, that.clusteringKeyColumnNames)
&& Objects.equals(clusteringOrders, that.clusteringOrders)
&& Objects.equals(options, that.options);
}
@Override
public int hashCode() {
return Objects.hash(
table,
ifNotExists,
columns,
partitionKeyColumnNames,
clusteringKeyColumnNames,
clusteringOrders,
options);
}
public static CreateTableStatement create(
TableRef table,
boolean ifNotExists,
ImmutableList columns,
ImmutableSet partitionKeyColumnNames,
ImmutableSet clusteringKeyColumnNames,
ImmutableMap clusteringOrders,
ImmutableMap options) {
return new CreateTableStatement(
table,
ifNotExists,
columns,
partitionKeyColumnNames,
clusteringKeyColumnNames,
clusteringOrders,
options);
}
public static class ColumnDef {
public final String name;
public final DataType dataType;
public final boolean encrypted;
private ColumnDef(String name, DataType dataType, boolean encrypted) {
this.name = name;
this.dataType = dataType;
this.encrypted = encrypted;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (!(o instanceof ColumnDef)) {
return false;
}
ColumnDef columnDef = (ColumnDef) o;
return encrypted == columnDef.encrypted
&& Objects.equals(name, columnDef.name)
&& dataType == columnDef.dataType;
}
@Override
public int hashCode() {
return Objects.hash(name, dataType, encrypted);
}
public static ColumnDef create(String name, DataType dataType, boolean encrypted) {
return new ColumnDef(name, dataType, encrypted);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy