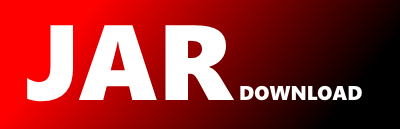
com.scalar.dl.client.config.ClientConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scalardl-java-client-sdk Show documentation
Show all versions of scalardl-java-client-sdk Show documentation
A client-side Java library to interact with Scalar DL network.
/*
* This file is part of the Scalar DL client SDK.
* Copyright (c) 2019 Scalar, Inc.
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
*
* You can be released from the requirements of the license by purchasing
* a commercial license. For more information, please contact Scalar, Inc.
*/
package com.scalar.dl.client.config;
import static com.google.common.base.Preconditions.checkArgument;
import com.scalar.dl.ledger.util.PropertiesUtil;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Optional;
import java.util.Properties;
import javax.annotation.concurrent.Immutable;
@Immutable
public class ClientConfig implements com.scalar.dl.ledger.config.ClientConfig {
public static final String PREFIX = "scalar.dl.client.";
public static final String SERVER_HOST = PREFIX + "server.host";
public static final String SERVER_PORT = PREFIX + "server.port";
public static final String SERVER_PRIVILEGED_PORT = PREFIX + "server.privileged_port";
public static final String CERT_HOLDER_ID = PREFIX + "cert_holder_id";
public static final String CERT_VERSION = PREFIX + "cert_version";
public static final String CERT_PATH = PREFIX + "cert_path";
public static final String CERT_PEM = PREFIX + "cert_pem";
public static final String PRIVATE_KEY_PATH = PREFIX + "private_key_path";
public static final String PRIVATE_KEY_PEM = PREFIX + "private_key_pem";
public static final String TLS_ENABLED = PREFIX + "tls.enabled";
public static final String TLS_CA_ROOT_CERT_PATH = PREFIX + "tls.ca_root_cert_path";
public static final String TLS_CA_ROOT_CERT_PEM = PREFIX + "tls.ca_root_cert_pem";
public static final String AUTHORIZATION_CREDENTIAL = PREFIX + "authorization.credential";
public static final String CLIENT_MODE = PREFIX + "mode";
public static final String PROXY_SERVER = PREFIX + "proxy.server";
public static final String AUDITOR_ENABLED = PREFIX + "auditor.enabled";
public static final String AUDITOR_HOST = PREFIX + "auditor.host";
public static final String AUDITOR_PORT = PREFIX + "auditor.port";
public static final String AUDITOR_PRIVILEGED_PORT = PREFIX + "auditor.privileged_port";
public static final String DEFAULT_SERVER_HOST = "localhost";
public static final int DEFAULT_SERVER_PORT = 50051;
public static final int DEFAULT_SERVER_PRIVILEGED_PORT = 50052;
public static final int DEFAULT_CERT_VERSION = 1;
public static final boolean DEFAULT_TLS_ENABLED = false;
public static final boolean DEFAULT_AUDITOR_ENABLED = false;
public static final String DEFAULT_AUDITOR_HOST = "localhost";
public static final int DEFAULT_AUDITOR_PORT = 40051;
public static final int DEFAULT_AUDITOR_PRIVILEGED_PORT = 40052;
public static final String AUDITOR_TLS_ENABLED = PREFIX + "auditor.tls.enabled";
public static final String AUDITOR_TLS_CA_ROOT_CERT_PATH =
PREFIX + "auditor.tls.ca_root_cert_path";
public static final String AUDITOR_TLS_CA_ROOT_CERT_PEM = PREFIX + "auditor.tls.ca_root_cert_pem";
public static final String AUDITOR_AUTHORIZATION_CREDENTIAL =
PREFIX + "auditor.authorization.credential";
public static final String AUDITOR_LINEARIZABLE_VALIDATION_ENABLED =
PREFIX + "auditor.linearizable_validation.enabled";
public static final String AUDITOR_LINEARIZABLE_VALIDATION_CONTRACT_ID =
PREFIX + "auditor.linearizable_validation.contract_id";
private final Properties props;
private String serverHost = DEFAULT_SERVER_HOST;
private int serverPort = DEFAULT_SERVER_PORT;
private int serverPrivilegedPort = DEFAULT_SERVER_PRIVILEGED_PORT;
private String certHolderId;
private int certVersion = DEFAULT_CERT_VERSION;
private String cert;
private String privateKey;
private boolean isTlsEnabled = DEFAULT_TLS_ENABLED;
private Optional tlsCaRootCert;
private Optional authorizationCredential;
private ClientMode clientMode = ClientMode.CLIENT;
private Server proxyServer;
private boolean isAuditorEnabled = DEFAULT_AUDITOR_ENABLED;
private String auditorHost = DEFAULT_AUDITOR_HOST;
private int auditorPort = DEFAULT_AUDITOR_PORT;
private int auditorPrivilegedPort = DEFAULT_AUDITOR_PRIVILEGED_PORT;
private boolean isAuditorTlsEnabled = DEFAULT_TLS_ENABLED;
private Optional auditorTlsCaRootCert;
private Optional auditorAuthorizationCredential;
private boolean isAuditorLinearizableValidationEnabled = false;
private String auditorLinearizableValidationContractId = "validate-ledger";
public ClientConfig(File propertiesFile) throws IOException {
this(new FileInputStream(propertiesFile));
}
public ClientConfig(InputStream stream) throws IOException {
props = new Properties();
props.load(stream);
load();
}
public ClientConfig(Properties properties) throws IOException {
props = new Properties();
properties.forEach((k, v) -> props.setProperty(k.toString(), v.toString()));
load();
}
public Properties getProperties() {
return props;
}
public String getServerHost() {
return serverHost;
}
@Override
public String getLedgerHost() {
return serverHost;
}
public int getServerPort() {
return serverPort;
}
@Override
public int getLedgerPort() {
return serverPort;
}
public int getServerPrivilegedPort() {
return serverPrivilegedPort;
}
@Override
public int getLedgerPrivilegedPort() {
return serverPrivilegedPort;
}
public String getCertHolderId() {
return certHolderId;
}
public int getCertVersion() {
return certVersion;
}
public String getCert() {
return cert;
}
public String getPrivateKey() {
return privateKey;
}
public boolean isTlsEnabled() {
return isTlsEnabled;
}
public Optional getTlsCaRootCert() {
return tlsCaRootCert;
}
public Optional getAuthorizationCredential() {
return authorizationCredential;
}
public ClientMode getClientMode() {
return clientMode;
}
public Server getProxyServer() {
return proxyServer;
}
public boolean isAuditorEnabled() {
return isAuditorEnabled;
}
public String getAuditorHost() {
return auditorHost;
}
public int getAuditorPort() {
return auditorPort;
}
public int getAuditorPrivilegedPort() {
return auditorPrivilegedPort;
}
public boolean isAuditorTlsEnabled() {
return isAuditorTlsEnabled;
}
public Optional getAuditorTlsCaRootCert() {
return auditorTlsCaRootCert;
}
public Optional getAuditorAuthorizationCredential() {
return auditorAuthorizationCredential;
}
public boolean isAuditorLinearizableValidationEnabled() {
return isAuditorLinearizableValidationEnabled;
}
public String getAuditorLinearizableValidationContractId() {
return auditorLinearizableValidationContractId;
}
private void load() throws IOException {
if (PropertiesUtil.isNonEmpty(props, SERVER_HOST)) {
serverHost = props.getProperty(SERVER_HOST);
}
if (PropertiesUtil.isNonEmpty(props, SERVER_PORT)) {
serverPort = Integer.parseInt(props.getProperty(SERVER_PORT));
}
if (PropertiesUtil.isNonEmpty(props, SERVER_PRIVILEGED_PORT)) {
serverPrivilegedPort = Integer.parseInt(props.getProperty(SERVER_PRIVILEGED_PORT));
}
if (PropertiesUtil.isNonEmpty(props, CLIENT_MODE)) {
clientMode = ClientMode.valueOf(props.getProperty(CLIENT_MODE));
}
if (clientMode.equals(ClientMode.CLIENT)) {
checkArgument(
PropertiesUtil.isNonEmpty(props, CERT_HOLDER_ID),
CERT_HOLDER_ID + " is missing but required.");
certHolderId = props.getProperty(CERT_HOLDER_ID);
if (PropertiesUtil.isNonEmpty(props, CERT_VERSION)) {
certVersion = Integer.parseInt(props.getProperty(CERT_VERSION));
}
cert = PropertiesUtil.readFromStringOrFile(props, CERT_PEM, CERT_PATH);
checkArgument(cert != null, "either " + CERT_PEM + " or " + CERT_PATH + " is required.");
privateKey = PropertiesUtil.readFromStringOrFile(props, PRIVATE_KEY_PEM, PRIVATE_KEY_PATH);
checkArgument(
privateKey != null,
"either " + PRIVATE_KEY_PEM + " or " + PRIVATE_KEY_PATH + " is required.");
}
if (PropertiesUtil.isNonEmpty(props, TLS_ENABLED)) {
isTlsEnabled = Boolean.parseBoolean(props.getProperty(TLS_ENABLED));
}
tlsCaRootCert =
Optional.ofNullable(
PropertiesUtil.readFromStringOrFile(
props, TLS_CA_ROOT_CERT_PEM, TLS_CA_ROOT_CERT_PATH));
authorizationCredential = Optional.ofNullable(props.getProperty(AUTHORIZATION_CREDENTIAL));
if (PropertiesUtil.isNonEmpty(props, PROXY_SERVER)) {
proxyServer = parseServers(props.getProperty(PROXY_SERVER)).get(0);
}
if (PropertiesUtil.isNonEmpty(props, AUDITOR_ENABLED)) {
isAuditorEnabled = Boolean.parseBoolean(props.getProperty(AUDITOR_ENABLED));
}
if (isAuditorEnabled) {
if (PropertiesUtil.isNonEmpty(props, AUDITOR_HOST)) {
auditorHost = props.getProperty(AUDITOR_HOST);
}
if (PropertiesUtil.isNonEmpty(props, AUDITOR_PORT)) {
auditorPort = Integer.parseInt(props.getProperty(AUDITOR_PORT));
}
if (PropertiesUtil.isNonEmpty(props, AUDITOR_PRIVILEGED_PORT)) {
auditorPrivilegedPort = Integer.parseInt(props.getProperty(AUDITOR_PRIVILEGED_PORT));
}
if (PropertiesUtil.isNonEmpty(props, AUDITOR_TLS_ENABLED)) {
isAuditorTlsEnabled = Boolean.parseBoolean(props.getProperty(AUDITOR_TLS_ENABLED));
}
auditorTlsCaRootCert =
Optional.ofNullable(
PropertiesUtil.readFromStringOrFile(
props, AUDITOR_TLS_CA_ROOT_CERT_PEM, AUDITOR_TLS_CA_ROOT_CERT_PATH));
auditorAuthorizationCredential =
Optional.ofNullable(props.getProperty(AUDITOR_AUTHORIZATION_CREDENTIAL));
if (PropertiesUtil.isNonEmpty(props, AUDITOR_LINEARIZABLE_VALIDATION_ENABLED)) {
isAuditorLinearizableValidationEnabled =
Boolean.parseBoolean(props.getProperty(AUDITOR_LINEARIZABLE_VALIDATION_ENABLED));
}
if (PropertiesUtil.isNonEmpty(props, AUDITOR_LINEARIZABLE_VALIDATION_CONTRACT_ID)) {
auditorLinearizableValidationContractId =
props.getProperty(AUDITOR_LINEARIZABLE_VALIDATION_CONTRACT_ID);
}
}
}
private List parseServers(String line) {
List list = new ArrayList<>();
for (String item : line.split(",")) {
String[] elements = item.split(":");
list.add(new Server(elements[0], Integer.parseInt(elements[1])));
}
return list;
}
public static class Server {
private final String host;
private final int port;
public Server(String host, int port) {
this.host = host;
this.port = port;
}
public String getHost() {
return host;
}
public int getPort() {
return port;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy