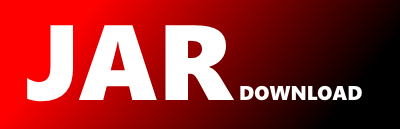
com.scalar.dl.client.tool.Common Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scalardl-java-client-sdk Show documentation
Show all versions of scalardl-java-client-sdk Show documentation
A client-side Java library to interact with Scalar DL network.
package com.scalar.dl.client.tool;
import com.scalar.dl.client.exception.ClientException;
import com.scalar.dl.ledger.service.StatusCode;
import java.io.StringWriter;
import java.util.HashMap;
import java.util.Map;
import javax.json.Json;
import javax.json.JsonObject;
import javax.json.JsonValue;
import javax.json.JsonWriter;
import javax.json.JsonWriterFactory;
import javax.json.stream.JsonGenerator;
public class Common {
static String STATUS_CODE_KEY = "status_code";
static String OUTPUT_KEY = "output";
static String ERROR_MESSAGE_KEY = "error_message";
static void printOutput(JsonValue value) {
printOutput(StatusCode.OK, value);
}
static void printOutput(StatusCode code, JsonValue value) {
JsonObject json =
Json.createObjectBuilder()
.add(Common.STATUS_CODE_KEY, code.toString())
.add(Common.OUTPUT_KEY, value)
.add(Common.ERROR_MESSAGE_KEY, JsonValue.NULL)
.build();
printJson(json);
}
static void printError(ClientException e) {
JsonObject json =
Json.createObjectBuilder()
.add(Common.STATUS_CODE_KEY, e.getStatusCode().toString())
.add(Common.OUTPUT_KEY, JsonValue.NULL)
.add(Common.ERROR_MESSAGE_KEY, e.getMessage())
.build();
printJson(json);
}
static void printJson(JsonObject json) {
Map properties = new HashMap<>();
properties.put(JsonGenerator.PRETTY_PRINTING, true);
JsonWriterFactory writerFactory = Json.createWriterFactory(properties);
StringWriter stringWriter = new StringWriter();
JsonWriter jsonWriter = writerFactory.createWriter(stringWriter);
jsonWriter.writeObject(json);
System.out.println(stringWriter.toString().trim());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy