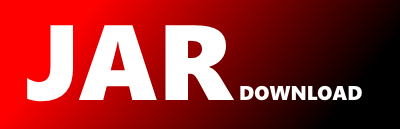
com.scalar.dl.client.tool.SmallbankLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scalardl-java-client-sdk Show documentation
Show all versions of scalardl-java-client-sdk Show documentation
A client-side Java library to interact with Scalar DL network.
package com.scalar.dl.client.tool;
import com.scalar.dl.client.config.ClientConfig;
import com.scalar.dl.client.exception.ClientException;
import com.scalar.dl.client.service.ClientService;
import com.scalar.dl.client.service.ClientServiceFactory;
import java.io.File;
import java.util.Optional;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
import javax.json.Json;
import javax.json.JsonObject;
import picocli.CommandLine;
import picocli.CommandLine.Command;
@Command(name = "smallbank-loader", description = "Create accounts for smallbank workload.")
public class SmallbankLoader implements Callable {
@CommandLine.Option(
names = {"--properties", "--config"},
required = true,
paramLabel = "PROPERTIES_FILE",
description = "A configuration file in properties format.")
private String properties;
@CommandLine.Option(
names = {"--num-accounts"},
required = false,
paramLabel = "NUM_ACCOUNTS",
description = "The number of target accounts.")
private int numAccounts = 10000;
@CommandLine.Option(
names = {"--num-threads"},
required = false,
paramLabel = "NUM_THREADS",
description = "The number of threads to run.")
private int numThreads = 1;
@CommandLine.Option(
names = {"-h", "--help"},
usageHelp = true,
description = "display the help message.")
boolean helpRequested;
private static final AtomicInteger counter = new AtomicInteger(0);
private static final String contractId = "create_account";
private static final int DEFAULT_BALANCE = 100000;
public static void main(String[] args) {
int exitCode = new CommandLine(new SmallbankLoader()).execute(args);
System.exit(exitCode);
}
@Override
public Integer call() throws Exception {
ClientServiceFactory factory = new ClientServiceFactory(new ClientConfig(new File(properties)));
ClientService service = factory.getClientService();
ExecutorService executor = Executors.newFixedThreadPool(numThreads);
for (int i = 0; i < numThreads; ++i) {
executor.execute(
() -> {
while (true) {
int id = counter.getAndIncrement();
JsonObject jsonArgument =
Json.createObjectBuilder()
.add("customer_id", id)
.add("customer_name", "Name " + id)
.add("initial_checking_balance", DEFAULT_BALANCE)
.add("initial_savings_balance", DEFAULT_BALANCE)
.build();
try {
if (counter.get() > numAccounts) {
break;
}
service.executeContract(contractId, jsonArgument, Optional.empty());
} catch (ClientException e) {
e.printStackTrace();
}
}
});
}
while (counter.get() <= numAccounts) {
System.out.println(counter.get() + " assets are loaded.");
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
// ignore
}
}
executor.shutdown();
executor.awaitTermination(10, TimeUnit.SECONDS);
return 0;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy