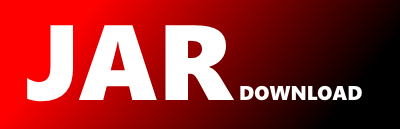
com.scalar.dl.client.config.IdentityConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scalardl-java-client-sdk Show documentation
Show all versions of scalardl-java-client-sdk Show documentation
A client-side Java library to interact with Scalar DL network.
package com.scalar.dl.client.config;
import static com.google.common.base.Preconditions.checkArgument;
import java.util.Objects;
import javax.annotation.concurrent.Immutable;
@Immutable
public class IdentityConfig {
private final String certHolderId;
private final int certVersion;
private final String cert;
private final String privateKey;
private IdentityConfig(IdentityConfig.Builder builder) {
this.certHolderId = builder.certHolderId;
this.certVersion = builder.certVersion;
this.cert = builder.cert;
this.privateKey = builder.privateKey;
}
public String getCertHolderId() {
return certHolderId;
}
public int getCertVersion() {
return certVersion;
}
public String getCert() {
return cert;
}
public String getPrivateKey() {
return privateKey;
}
@Override
public int hashCode() {
return Objects.hash(certHolderId, certVersion, cert, privateKey);
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (!(o instanceof IdentityConfig)) {
return false;
}
IdentityConfig other = (IdentityConfig) o;
return getCertHolderId().equals(other.getCertHolderId())
&& getCertVersion() == other.getCertVersion()
&& getCert().equals(other.getCert())
&& getPrivateKey().equals(other.getPrivateKey());
}
public static IdentityConfig.Builder newBuilder() {
return new IdentityConfig.Builder();
}
public static final class Builder {
private String certHolderId;
private int certVersion;
private String cert;
private String privateKey;
Builder() {
this.certHolderId = null;
this.certVersion = -1;
this.cert = null;
this.privateKey = null;
}
public IdentityConfig.Builder certHolderId(String certHolderId) {
checkArgument(certHolderId != null);
this.certHolderId = certHolderId;
return this;
}
public IdentityConfig.Builder certVersion(int certVersion) {
checkArgument(certVersion >= 0);
this.certVersion = certVersion;
return this;
}
public IdentityConfig.Builder cert(String cert) {
checkArgument(cert != null);
this.cert = cert;
return this;
}
public IdentityConfig.Builder privateKey(String privateKey) {
checkArgument(privateKey != null);
this.privateKey = privateKey;
return this;
}
public IdentityConfig build() {
if (certHolderId == null || certVersion < 0 || cert == null || privateKey == null) {
throw new IllegalArgumentException("Required fields are not given.");
}
return new IdentityConfig(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy