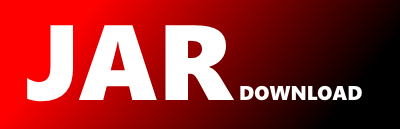
com.scalar.dl.client.tool.ContractsRegistration Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scalardl-java-client-sdk Show documentation
Show all versions of scalardl-java-client-sdk Show documentation
A client-side Java library to interact with Scalar DL network.
package com.scalar.dl.client.tool;
import com.fasterxml.jackson.databind.JsonNode;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.moandjiezana.toml.Toml;
import com.scalar.dl.client.config.ClientConfig;
import com.scalar.dl.client.exception.ClientException;
import com.scalar.dl.client.service.ClientService;
import com.scalar.dl.client.service.ClientServiceFactory;
import com.scalar.dl.ledger.service.StatusCode;
import com.scalar.dl.ledger.util.JacksonSerDe;
import java.io.File;
import java.util.concurrent.Callable;
import picocli.CommandLine;
import picocli.CommandLine.Command;
@Command(name = "register-contracts", description = "Register specified contracts.")
public class ContractsRegistration implements Callable {
@CommandLine.Option(
names = {"--properties", "--config"},
required = true,
paramLabel = "PROPERTIES_FILE",
description = "A configuration file in properties format.")
private String properties;
@CommandLine.Option(
names = {"--contracts-file"},
required = true,
paramLabel = "CONTRACTS_FILE",
description = "A file including contracts to register in TOML format.")
private String contractsFile;
@CommandLine.Option(
names = "--ignore-registered",
description = "Ignore an already registered contract.")
private boolean ignoreRegistered;
@CommandLine.Option(
names = {"-h", "--help"},
usageHelp = true,
description = "display the help message.")
boolean helpRequested;
public static void main(String[] args) {
int exitCode = new CommandLine(new ContractsRegistration()).execute(args);
System.exit(exitCode);
}
@Override
public Integer call() throws Exception {
ClientServiceFactory factory = new ClientServiceFactory();
ClientService service = factory.create(new ClientConfig(new File(properties)));
JacksonSerDe serde = new JacksonSerDe(new ObjectMapper());
try {
new Toml()
.read(new File(contractsFile))
.getTables("contracts")
.forEach(
each -> {
JsonNode properties =
each.contains("properties")
? serde.deserialize(each.getString("properties"))
: null;
try {
service.registerContract(
each.getString("contract-id"),
each.getString("contract-binary-name"),
each.getString("contract-class-file"),
properties);
} catch (ClientException e) {
if (!ignoreRegistered
|| e.getStatusCode() != StatusCode.CONTRACT_ALREADY_REGISTERED) {
throw e;
}
}
});
Common.printOutput(null);
return 0;
} catch (ClientException e) {
Common.printError(e);
return 1;
} finally {
factory.close();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy