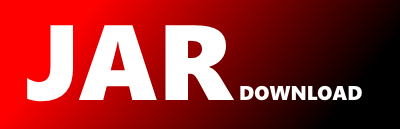
com.scalar.dl.ledger.function.FunctionBase Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scalardl-java-client-sdk Show documentation
Show all versions of scalardl-java-client-sdk Show documentation
A client-side Java library to interact with Scalar DL network.
package com.scalar.dl.ledger.function;
import static com.google.common.base.Preconditions.checkArgument;
import static com.google.common.base.Preconditions.checkNotNull;
import com.scalar.dl.ledger.database.Database;
import com.scalar.dl.ledger.exception.ContractContextException;
import javax.annotation.Nullable;
abstract class FunctionBase {
private FunctionManager manager;
protected T contractProperties;
protected T contractContext;
private boolean isRoot;
void initialize(FunctionManager manager) {
this.manager = checkNotNull(manager);
}
abstract void setContractProperties(@Nullable String contractProperties);
@Nullable
protected T getContractProperties() {
return contractProperties;
}
void setRoot(boolean isRoot) {
this.isRoot = isRoot;
}
public boolean isRoot() {
return isRoot;
}
@SuppressWarnings("unchecked")
void setContractContext(@Nullable Object contractContext) {
this.contractContext = (T) contractContext;
}
/**
* Returns a contract context given from a contract that is executed together with the function.
*
* @return contract context
*/
@Nullable
protected T getContractContext() {
return contractContext;
}
@Nullable
abstract String invokeRoot(
Database database, @Nullable String functionArgument, String contractArgument);
/**
* Invokes the function to {@link Database} with the specified argument and the corresponding root
* contract argument and properties. An implementation of the {@code FunctionBase} should throw
* {@link ContractContextException} if it faces application-level contextual error (such as lack
* of balance in payment application).
*
* @param database mutable database
* @param functionArgument function argument
* @param contractArgument the argument of the corresponding root contract
* @param contractProperties the pre-registered properties of the corresponding root contract
*/
@Nullable
public abstract T invoke(
Database database,
@Nullable T functionArgument,
T contractArgument,
@Nullable T contractProperties);
@SuppressWarnings("unchecked")
@Nullable
protected final T invoke(
String functionId,
Database database,
@Nullable T functionArgument,
T contractArgument) {
checkArgument(manager != null, "please call initialize() before this.");
FunctionBase function =
(FunctionBase) manager.getInstance(functionId).getFunctionBase();
function.setContractContext(contractContext); // context is propagated to all the functions
return function.invoke(database, functionArgument, contractArgument, contractProperties);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy