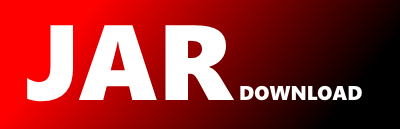
com.scalar.dl.rpc.GatewayGrpc Maven / Gradle / Ivy
The newest version!
package com.scalar.dl.rpc;
import static io.grpc.MethodDescriptor.generateFullMethodName;
/**
*/
@javax.annotation.Generated(
value = "by gRPC proto compiler (version 1.68.1)",
comments = "Source: scalar.proto")
@io.grpc.stub.annotations.GrpcGenerated
public final class GatewayGrpc {
private GatewayGrpc() {}
public static final java.lang.String SERVICE_NAME = "rpc.Gateway";
// Static method descriptors that strictly reflect the proto.
private static volatile io.grpc.MethodDescriptor getRegisterContractMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "RegisterContract",
requestType = com.scalar.dl.rpc.ContractRegistrationRequest.class,
responseType = com.google.protobuf.Empty.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getRegisterContractMethod() {
io.grpc.MethodDescriptor getRegisterContractMethod;
if ((getRegisterContractMethod = GatewayGrpc.getRegisterContractMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getRegisterContractMethod = GatewayGrpc.getRegisterContractMethod) == null) {
GatewayGrpc.getRegisterContractMethod = getRegisterContractMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "RegisterContract"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.scalar.dl.rpc.ContractRegistrationRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.google.protobuf.Empty.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("RegisterContract"))
.build();
}
}
}
return getRegisterContractMethod;
}
private static volatile io.grpc.MethodDescriptor getListContractsMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ListContracts",
requestType = com.scalar.dl.rpc.ContractsListingRequest.class,
responseType = com.scalar.dl.rpc.ContractsListingResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getListContractsMethod() {
io.grpc.MethodDescriptor getListContractsMethod;
if ((getListContractsMethod = GatewayGrpc.getListContractsMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getListContractsMethod = GatewayGrpc.getListContractsMethod) == null) {
GatewayGrpc.getListContractsMethod = getListContractsMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ListContracts"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.scalar.dl.rpc.ContractsListingRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.scalar.dl.rpc.ContractsListingResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("ListContracts"))
.build();
}
}
}
return getListContractsMethod;
}
private static volatile io.grpc.MethodDescriptor getExecuteContractMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ExecuteContract",
requestType = com.scalar.dl.rpc.ContractExecutionRequest.class,
responseType = com.scalar.dl.rpc.ContractExecutionResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getExecuteContractMethod() {
io.grpc.MethodDescriptor getExecuteContractMethod;
if ((getExecuteContractMethod = GatewayGrpc.getExecuteContractMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getExecuteContractMethod = GatewayGrpc.getExecuteContractMethod) == null) {
GatewayGrpc.getExecuteContractMethod = getExecuteContractMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ExecuteContract"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.scalar.dl.rpc.ContractExecutionRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.scalar.dl.rpc.ContractExecutionResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("ExecuteContract"))
.build();
}
}
}
return getExecuteContractMethod;
}
private static volatile io.grpc.MethodDescriptor getValidateLedgerMethod;
@io.grpc.stub.annotations.RpcMethod(
fullMethodName = SERVICE_NAME + '/' + "ValidateLedger",
requestType = com.scalar.dl.rpc.LedgerValidationRequest.class,
responseType = com.scalar.dl.rpc.LedgerValidationResponse.class,
methodType = io.grpc.MethodDescriptor.MethodType.UNARY)
public static io.grpc.MethodDescriptor getValidateLedgerMethod() {
io.grpc.MethodDescriptor getValidateLedgerMethod;
if ((getValidateLedgerMethod = GatewayGrpc.getValidateLedgerMethod) == null) {
synchronized (GatewayGrpc.class) {
if ((getValidateLedgerMethod = GatewayGrpc.getValidateLedgerMethod) == null) {
GatewayGrpc.getValidateLedgerMethod = getValidateLedgerMethod =
io.grpc.MethodDescriptor.newBuilder()
.setType(io.grpc.MethodDescriptor.MethodType.UNARY)
.setFullMethodName(generateFullMethodName(SERVICE_NAME, "ValidateLedger"))
.setSampledToLocalTracing(true)
.setRequestMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.scalar.dl.rpc.LedgerValidationRequest.getDefaultInstance()))
.setResponseMarshaller(io.grpc.protobuf.ProtoUtils.marshaller(
com.scalar.dl.rpc.LedgerValidationResponse.getDefaultInstance()))
.setSchemaDescriptor(new GatewayMethodDescriptorSupplier("ValidateLedger"))
.build();
}
}
}
return getValidateLedgerMethod;
}
/**
* Creates a new async stub that supports all call types for the service
*/
public static GatewayStub newStub(io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GatewayStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayStub(channel, callOptions);
}
};
return GatewayStub.newStub(factory, channel);
}
/**
* Creates a new blocking-style stub that supports unary and streaming output calls on the service
*/
public static GatewayBlockingStub newBlockingStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GatewayBlockingStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayBlockingStub(channel, callOptions);
}
};
return GatewayBlockingStub.newStub(factory, channel);
}
/**
* Creates a new ListenableFuture-style stub that supports unary calls on the service
*/
public static GatewayFutureStub newFutureStub(
io.grpc.Channel channel) {
io.grpc.stub.AbstractStub.StubFactory factory =
new io.grpc.stub.AbstractStub.StubFactory() {
@java.lang.Override
public GatewayFutureStub newStub(io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayFutureStub(channel, callOptions);
}
};
return GatewayFutureStub.newStub(factory, channel);
}
/**
*/
public interface AsyncService {
/**
*/
default void registerContract(com.scalar.dl.rpc.ContractRegistrationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getRegisterContractMethod(), responseObserver);
}
/**
*/
default void listContracts(com.scalar.dl.rpc.ContractsListingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getListContractsMethod(), responseObserver);
}
/**
*/
default void executeContract(com.scalar.dl.rpc.ContractExecutionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getExecuteContractMethod(), responseObserver);
}
/**
*/
default void validateLedger(com.scalar.dl.rpc.LedgerValidationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ServerCalls.asyncUnimplementedUnaryCall(getValidateLedgerMethod(), responseObserver);
}
}
/**
* Base class for the server implementation of the service Gateway.
*/
public static abstract class GatewayImplBase
implements io.grpc.BindableService, AsyncService {
@java.lang.Override public final io.grpc.ServerServiceDefinition bindService() {
return GatewayGrpc.bindService(this);
}
}
/**
* A stub to allow clients to do asynchronous rpc calls to service Gateway.
*/
public static final class GatewayStub
extends io.grpc.stub.AbstractAsyncStub {
private GatewayStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GatewayStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayStub(channel, callOptions);
}
/**
*/
public void registerContract(com.scalar.dl.rpc.ContractRegistrationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getRegisterContractMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void listContracts(com.scalar.dl.rpc.ContractsListingRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getListContractsMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void executeContract(com.scalar.dl.rpc.ContractExecutionRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getExecuteContractMethod(), getCallOptions()), request, responseObserver);
}
/**
*/
public void validateLedger(com.scalar.dl.rpc.LedgerValidationRequest request,
io.grpc.stub.StreamObserver responseObserver) {
io.grpc.stub.ClientCalls.asyncUnaryCall(
getChannel().newCall(getValidateLedgerMethod(), getCallOptions()), request, responseObserver);
}
}
/**
* A stub to allow clients to do synchronous rpc calls to service Gateway.
*/
public static final class GatewayBlockingStub
extends io.grpc.stub.AbstractBlockingStub {
private GatewayBlockingStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GatewayBlockingStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayBlockingStub(channel, callOptions);
}
/**
*/
public com.google.protobuf.Empty registerContract(com.scalar.dl.rpc.ContractRegistrationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getRegisterContractMethod(), getCallOptions(), request);
}
/**
*/
public com.scalar.dl.rpc.ContractsListingResponse listContracts(com.scalar.dl.rpc.ContractsListingRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getListContractsMethod(), getCallOptions(), request);
}
/**
*/
public com.scalar.dl.rpc.ContractExecutionResponse executeContract(com.scalar.dl.rpc.ContractExecutionRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getExecuteContractMethod(), getCallOptions(), request);
}
/**
*/
public com.scalar.dl.rpc.LedgerValidationResponse validateLedger(com.scalar.dl.rpc.LedgerValidationRequest request) {
return io.grpc.stub.ClientCalls.blockingUnaryCall(
getChannel(), getValidateLedgerMethod(), getCallOptions(), request);
}
}
/**
* A stub to allow clients to do ListenableFuture-style rpc calls to service Gateway.
*/
public static final class GatewayFutureStub
extends io.grpc.stub.AbstractFutureStub {
private GatewayFutureStub(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
super(channel, callOptions);
}
@java.lang.Override
protected GatewayFutureStub build(
io.grpc.Channel channel, io.grpc.CallOptions callOptions) {
return new GatewayFutureStub(channel, callOptions);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture registerContract(
com.scalar.dl.rpc.ContractRegistrationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getRegisterContractMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture listContracts(
com.scalar.dl.rpc.ContractsListingRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getListContractsMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture executeContract(
com.scalar.dl.rpc.ContractExecutionRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getExecuteContractMethod(), getCallOptions()), request);
}
/**
*/
public com.google.common.util.concurrent.ListenableFuture validateLedger(
com.scalar.dl.rpc.LedgerValidationRequest request) {
return io.grpc.stub.ClientCalls.futureUnaryCall(
getChannel().newCall(getValidateLedgerMethod(), getCallOptions()), request);
}
}
private static final int METHODID_REGISTER_CONTRACT = 0;
private static final int METHODID_LIST_CONTRACTS = 1;
private static final int METHODID_EXECUTE_CONTRACT = 2;
private static final int METHODID_VALIDATE_LEDGER = 3;
private static final class MethodHandlers implements
io.grpc.stub.ServerCalls.UnaryMethod,
io.grpc.stub.ServerCalls.ServerStreamingMethod,
io.grpc.stub.ServerCalls.ClientStreamingMethod,
io.grpc.stub.ServerCalls.BidiStreamingMethod {
private final AsyncService serviceImpl;
private final int methodId;
MethodHandlers(AsyncService serviceImpl, int methodId) {
this.serviceImpl = serviceImpl;
this.methodId = methodId;
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public void invoke(Req request, io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
case METHODID_REGISTER_CONTRACT:
serviceImpl.registerContract((com.scalar.dl.rpc.ContractRegistrationRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_LIST_CONTRACTS:
serviceImpl.listContracts((com.scalar.dl.rpc.ContractsListingRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_EXECUTE_CONTRACT:
serviceImpl.executeContract((com.scalar.dl.rpc.ContractExecutionRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
case METHODID_VALIDATE_LEDGER:
serviceImpl.validateLedger((com.scalar.dl.rpc.LedgerValidationRequest) request,
(io.grpc.stub.StreamObserver) responseObserver);
break;
default:
throw new AssertionError();
}
}
@java.lang.Override
@java.lang.SuppressWarnings("unchecked")
public io.grpc.stub.StreamObserver invoke(
io.grpc.stub.StreamObserver responseObserver) {
switch (methodId) {
default:
throw new AssertionError();
}
}
}
public static final io.grpc.ServerServiceDefinition bindService(AsyncService service) {
return io.grpc.ServerServiceDefinition.builder(getServiceDescriptor())
.addMethod(
getRegisterContractMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.scalar.dl.rpc.ContractRegistrationRequest,
com.google.protobuf.Empty>(
service, METHODID_REGISTER_CONTRACT)))
.addMethod(
getListContractsMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.scalar.dl.rpc.ContractsListingRequest,
com.scalar.dl.rpc.ContractsListingResponse>(
service, METHODID_LIST_CONTRACTS)))
.addMethod(
getExecuteContractMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.scalar.dl.rpc.ContractExecutionRequest,
com.scalar.dl.rpc.ContractExecutionResponse>(
service, METHODID_EXECUTE_CONTRACT)))
.addMethod(
getValidateLedgerMethod(),
io.grpc.stub.ServerCalls.asyncUnaryCall(
new MethodHandlers<
com.scalar.dl.rpc.LedgerValidationRequest,
com.scalar.dl.rpc.LedgerValidationResponse>(
service, METHODID_VALIDATE_LEDGER)))
.build();
}
private static abstract class GatewayBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoFileDescriptorSupplier, io.grpc.protobuf.ProtoServiceDescriptorSupplier {
GatewayBaseDescriptorSupplier() {}
@java.lang.Override
public com.google.protobuf.Descriptors.FileDescriptor getFileDescriptor() {
return com.scalar.dl.rpc.ScalarProto.getDescriptor();
}
@java.lang.Override
public com.google.protobuf.Descriptors.ServiceDescriptor getServiceDescriptor() {
return getFileDescriptor().findServiceByName("Gateway");
}
}
private static final class GatewayFileDescriptorSupplier
extends GatewayBaseDescriptorSupplier {
GatewayFileDescriptorSupplier() {}
}
private static final class GatewayMethodDescriptorSupplier
extends GatewayBaseDescriptorSupplier
implements io.grpc.protobuf.ProtoMethodDescriptorSupplier {
private final java.lang.String methodName;
GatewayMethodDescriptorSupplier(java.lang.String methodName) {
this.methodName = methodName;
}
@java.lang.Override
public com.google.protobuf.Descriptors.MethodDescriptor getMethodDescriptor() {
return getServiceDescriptor().findMethodByName(methodName);
}
}
private static volatile io.grpc.ServiceDescriptor serviceDescriptor;
public static io.grpc.ServiceDescriptor getServiceDescriptor() {
io.grpc.ServiceDescriptor result = serviceDescriptor;
if (result == null) {
synchronized (GatewayGrpc.class) {
result = serviceDescriptor;
if (result == null) {
serviceDescriptor = result = io.grpc.ServiceDescriptor.newBuilder(SERVICE_NAME)
.setSchemaDescriptor(new GatewayFileDescriptorSupplier())
.addMethod(getRegisterContractMethod())
.addMethod(getListContractsMethod())
.addMethod(getExecuteContractMethod())
.addMethod(getValidateLedgerMethod())
.build();
}
}
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy