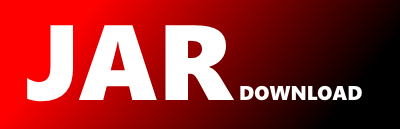
sirius.biz.storage.BucketInfo Maven / Gradle / Ivy
Show all versions of sirius-biz Show documentation
/*
* Made with all the love in the world
* by scireum in Remshalden, Germany
*
* Copyright by scireum GmbH
* http://www.scireum.de - [email protected]
*/
package sirius.biz.storage;
import sirius.biz.tenants.Tenant;
import sirius.db.mixing.OMA;
import sirius.kernel.di.GlobalContext;
import sirius.kernel.di.std.Part;
import sirius.kernel.nls.NLS;
import sirius.kernel.settings.Extension;
import sirius.web.security.UserContext;
/**
* Represents metadata available for a bucket.
*
* Most of this is taken from storage.buckets.[bucketName] from the system config.
*/
public class BucketInfo {
private String name;
private String description;
private String permission;
private boolean canCreate;
private boolean canEdit;
private boolean canDelete;
private int deleteFilesAfterDays;
private PhysicalStorageEngine engine;
@Part
private static OMA oma;
@Part
private static GlobalContext context;
/**
* Creates a new bucket info based on the given config section.
*
* @param extension the config section holding the bucket infos
*/
protected BucketInfo(Extension extension) {
this.name = extension.getId();
this.description = NLS.getIfExists("Storage.bucket." + this.name, NLS.getCurrentLang()).orElse("");
this.permission = extension.get("permission").asString();
this.canCreate = extension.get("canCreate").asBoolean();
this.canEdit = extension.get("canEdit").asBoolean();
this.canDelete = extension.get("canDelete").asBoolean();
this.deleteFilesAfterDays = extension.get("deleteFilesAfterDays").asInt(0);
this.engine = context.findPart(extension.get("engine").asString(), PhysicalStorageEngine.class);
}
/**
* Counts the total number of objects in this bucket.
*
* @return the total number of objects in this bucket
*/
public long getNumberOfObjects() {
return oma.select(VirtualObject.class)
.eq(VirtualObject.TENANT, UserContext.getCurrentUser().as(Tenant.class))
.eq(VirtualObject.BUCKET, name)
.count();
}
/**
* Returns the (technical) name of the bucket.
*
* @return the name of the bucket
*/
public String getName() {
return name;
}
/**
* Returns the description of the bucket.
*
* @return the description of the bucket
*/
public String getDescription() {
return description;
}
/**
* Returns the permission required to access the contents of this bucket.
*
* @return the permission required to access this bucket or null if no special rights are needed
*/
public String getPermission() {
return permission;
}
/**
* Determines if a user can create new objects in this bucket.
*
* @return true if a user can create net objects, falefalse otherwise
*/
public boolean isCanCreate() {
return canCreate;
}
/**
* Determines if a user can edit objects within thi bucket.
*
* @return true if a user can modify an object within this bucket, false otherwise
*/
public boolean isCanEdit() {
return canEdit;
}
/**
* Determines if a user can delete objects within thi bucket.
*
* @return true if a user can delete an object within this bucket, false otherwise
*/
public boolean isCanDelete() {
return canDelete;
}
/**
* Determines the number of days after which objects are automatically deleted.
*
* This is used for "self organizing" buckets, which automatically drain old data.
*
* @return the number of days after which objects are deleted or 0 to disable auto-deletion
*/
public int getDeleteFilesAfterDays() {
return deleteFilesAfterDays;
}
/**
* Determines the {@link PhysicalStorageEngine} used to store objects within this bucket.
*
* @return the storage engine used by this bucket
*/
public PhysicalStorageEngine getEngine() {
return engine;
}
}