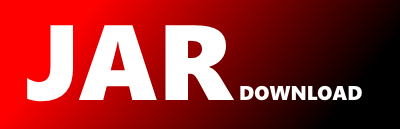
sirius.kernel.cache.Cache Maven / Gradle / Ivy
/*
* Made with all the love in the world
* by scireum in Remshalden, Germany
*
* Copyright by scireum GmbH
* http://www.scireum.de - [email protected]
*/
package sirius.kernel.cache;
import sirius.kernel.commons.Callback;
import sirius.kernel.commons.Tuple;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import java.util.Date;
import java.util.Iterator;
import java.util.List;
import java.util.function.Predicate;
/**
* Provides a cache which can be used to store and access values.
*
* In contrast to a simple Map, the cache has a maximum size. If the cache is full, old (least recently used)
* entries are evicted to make room for newer ones. Also this cache will be automatically evicted from time to
* time so that only values which are really used remain in the cache. Therefore system resources (most essentially
* heap storage) is released if no longer required.
*
* A new Cache is created by invoking {@link CacheManager#createCache(String)}. The maximal size as well as the
* time to live value for each entry is set via the cache.[cacheName] extension. Additionally
* {@link CacheManager#createCache(String, ValueComputer, ValueVerifier)} can be used to supply a
* ValueComputer as well as a ValueVerifier. Those classes are responsible for creating non-
* existent cache values or to verify that cached values are still up to date, before they are returned to a
* user of the cache.
*
* @param the key type determining the type of the lookup values in the cache
* @param the value type determining the type of values stored in the cache
* @see CacheManager
* @see ValueComputer
* @see ValueVerifier
*/
public interface Cache {
/**
* Returns the name of the cache, which is also used to load the configuration.
*
* @return the name of the cache
*/
String getName();
/**
* Returns the max size of the cache
*
* @return the maximal number of cached entries
*/
int getMaxSize();
/**
* Returns the number of entries in the cache
*
* @return the number of cached entries
*/
int getSize();
/**
* Returns the number of reads since the last eviction
*
* @return the number of uses of this cache since the last eviction run
*/
long getUses();
/**
* Returns the statistical values of "uses" for the last some eviction
* intervals.
*
* @return a list of uses for the last N eviction intervals
*/
List getUseHistory();
/**
* Returns the cache hit-rate (in percent)
*
* @return the cache hit-rate (successful gets) of this cache since the last eviction
*/
Long getHitRate();
/**
* Returns the statistical values of "hit rate" for the last some eviction
* intervals.
*
* @return a list of hit-rates for the last N eviction intervals
*/
List getHitRateHistory();
/**
* Returns the date of the last eviction run.
*
* @return the timestamp of the last eviction
*/
Date getLastEvictionRun();
/**
* Clears the complete cache
*/
void clear();
/**
* Returns the value associated with the given key.
*
* @param key the key used to retrieve the value in the cache
* @return the cached value or null if neither a valid value was found, nor one could be
* computed.
*/
@Nullable
V get(@Nonnull K key);
/**
* Returns the value associated with the given key. If the value is not
* found, the {@link ValueComputer} is invoked.
*
* @param key the key used to retrieve the value in the cache
* @param computer the computer used to generate a value if absent in the cache
* @return the cached value or null if neither a valid value was found, nor one could be
* computed.
*/
@Nullable
V get(@Nonnull K key, @Nullable ValueComputer computer);
/**
* Stores the given key value mapping in the cache
*
* @param key the key used to store this entry
* @param value the value to be stored in the entry
*/
void put(@Nonnull K key, @Nullable V value);
/**
* Removes the given item from the cache
*
* @param key the key which should be removed from the cache
*/
void remove(@Nonnull K key);
/**
* Removes all cached values for which the predicate returns true.
*
* @param predicate the predicate used to determine if a value should be removed from the cache.
*/
void removeIf(@Nonnull Predicate> predicate);
/**
* Provides access to the keys stored in this cache
*
* @return an Iterator for all keys in the cache
*/
Iterator keySet();
/**
* Provides access to the contents of this cache
*
* @return a list of entries which provide detailed information about each entry in the cache
*/
List> getContents();
/**
* Sets the remove callback which is invoked once a value is removed from the cache.
*
* Only one handler can be set at a time.
*
* @param onRemoveCallback the callback to call when an element is removed from the cache. Can be null
* null to remove the last handler.
* @return the original instance of the cache.
*/
Cache onRemove(Callback> onRemoveCallback);
}