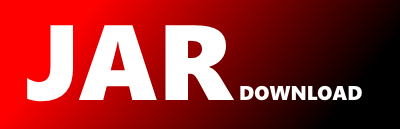
sirius.kernel.commons.Context Maven / Gradle / Ivy
Show all versions of sirius-kernel Show documentation
/*
* Made with all the love in the world
* by scireum in Remshalden, Germany
*
* Copyright by scireum GmbH
* http://www.scireum.de - [email protected]
*/
package sirius.kernel.commons;
import javax.annotation.Nonnull;
import javax.script.ScriptContext;
import java.util.Collection;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
/**
* Provides an execution context to scripts etc.
*
* This is basically a wrapper for {@code Map<String, Object>}
*/
public class Context implements Map {
protected Map data = new TreeMap<>();
@Override
public int size() {
return data.size();
}
@Override
public boolean isEmpty() {
return data.isEmpty();
}
@Override
public boolean containsKey(Object key) {
return data.containsKey(key);
}
@Override
public boolean containsValue(Object value) {
return data.containsValue(value);
}
@Override
public Object get(Object key) {
return data.get(key);
}
/**
* Provides the value associated with the given key as {@link Value}
*
* @param key the key for which the value should ne returned
* @return a value wrapping the internally associated value for the given key
*/
@Nonnull
public Value getValue(Object key) {
return Value.of(get(key));
}
@Override
public Object put(String key, Object value) {
set(key, value);
return value;
}
/**
* Sets the given value (its string representation), mit limits this to limit characters.
*
* @param key the key to which the value should be associated
* @param value the value which string representation should be put into the context
* @param limit the maximal number of characters to put into the map. Everything after that will be discarded
*/
public void putLimited(String key, Object value, int limit) {
set(key, Strings.limit(value, limit));
}
@Override
public Object remove(Object key) {
return data.remove(key);
}
@Override
public void putAll(Map extends String, ?> m) {
data.putAll(m);
}
@Override
public void clear() {
data.clear();
}
@Override
public Set keySet() {
return data.keySet();
}
@Override
public Collection