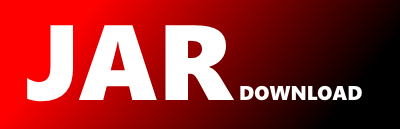
sirius.search.properties.StringListMapProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sirius-search Show documentation
Show all versions of sirius-search Show documentation
Provides a thin layer above Elasticsearch (Fluent Query API, Automatic Mapping, Utilities)
The newest version!
/*
* Made with all the love in the world
* by scireum in Remshalden, Germany
*
* Copyright by scireum GmbH
* http://www.scireum.de - [email protected]
*/
package sirius.search.properties;
import sirius.kernel.commons.Context;
import sirius.kernel.di.std.Register;
import sirius.search.annotations.MapType;
import java.lang.reflect.Field;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Represents a property which contains a map of strings to strings. Such fields must wear a {@link
* sirius.search.annotations.ListType} annotation with String as their value.
*/
public class StringListMapProperty extends StringMapProperty {
/**
* Factory for generating properties based on their field type
*/
@Register
public static class Factory implements PropertyFactory {
@Override
public boolean accepts(Field field) {
return Map.class.equals(field.getType()) && field.isAnnotationPresent(MapType.class) && List.class.equals(
field.getAnnotation(MapType.class).value());
}
@Override
public Property create(Field field) {
return new StringListMapProperty(field);
}
}
/*
* Instances are only created by the factory
*/
private StringListMapProperty(Field field) {
super(field);
}
@Override
@SuppressWarnings("unchecked")
protected Object transformFromSource(Object value) {
Map> result = new HashMap<>();
if (value instanceof Collection) {
((Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy