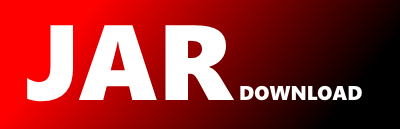
com.scudata.cellset.graph.draw.DrawArea Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.cellset.graph.draw;
import java.awt.*;
import java.awt.geom.Point2D;
import java.awt.geom.Rectangle2D;
import java.util.ArrayList;
import com.scudata.cellset.graph.*;
import com.scudata.chart.Consts;
import com.scudata.chart.Utils;
/**
* ???ͼ??ʵ??
* @author Joancy
*
*/
public class DrawArea extends DrawBase {
/**
* ʵ?ֻ?ͼ????
*/
public void draw(StringBuffer htmlLink) {
drawing(this, htmlLink);
}
/**
* ???ݻ?ͼ????db??ͼ????????ͼ??ij????Ӵ???htmlLink
* @param db ????Ļ?ͼ????
* @param htmlLink ?????ӻ???
*/
public static void drawing(DrawBase db,StringBuffer htmlLink) {
//?ٸĶ????룬ͬ??????Ҫ?õ???ʵ??
GraphParam gp = db.gp;
ExtGraphProperty egp = db.egp;
Graphics2D g = db.g;
ArrayList labelList = db.labelList;
int VALUE_RADIUS = db.VALUE_RADIUS;
double seriesWidth;
double coorWidth;
double categorySpan;
double dely;
double tmpInt;
double x, y;
gp.coorWidth = 0;
Point2D.Double headPoint[];
db.initGraphInset();
db.createCoorValue();
db.drawLegend(htmlLink);
db.drawTitle();
db.drawLabel();
db.keepGraphSpace();
db.adjustCoorInset();
gp.graphRect = new Rectangle2D.Double(gp.leftInset, gp.topInset, gp.graphWidth
- gp.leftInset - gp.rightInset, gp.graphHeight - gp.topInset
- gp.bottomInset);
if (gp.graphRect.width < 10 || gp.graphRect.height < 10) {
return;
}
if (gp.coorWidth < 0 || gp.coorWidth > 10000) {
gp.coorWidth = 0;
}
seriesWidth = gp.graphRect.width
/ (((gp.catNum + 1) * gp.categorySpan / 100.0) + gp.coorWidth
/ 200.0 + gp.catNum * gp.serNum);
coorWidth = seriesWidth * (gp.coorWidth / 200.0);
categorySpan = seriesWidth * (gp.categorySpan / 100.0);
tmpInt = (gp.catNum + 1) * categorySpan + coorWidth + gp.catNum
* gp.serNum * seriesWidth;
gp.graphRect.x += (gp.graphRect.width - tmpInt) / 2;
gp.graphRect.width = tmpInt;
dely = (gp.graphRect.height - coorWidth) / gp.tickNum;
tmpInt = dely * gp.tickNum + coorWidth;
gp.graphRect.y += (gp.graphRect.height - tmpInt) / 2;
gp.graphRect.height = tmpInt;
gp.gRect1 = (Rectangle2D.Double)gp.graphRect.clone();
gp.gRect2 = (Rectangle2D.Double)gp.graphRect.clone();
gp.gRect1.y += coorWidth;
gp.gRect1.width -= coorWidth;
gp.gRect1.height -= coorWidth;
gp.gRect2.x += coorWidth;
gp.gRect2.width -= coorWidth;
gp.gRect2.height -= coorWidth;
/* ???????? */
db.drawGraphRect();
/* ??Y?? */
for (int i = 0; i <= gp.tickNum; i++) {
db.drawGridLine(dely, i);
Number coory = (Number) gp.coorValue.get(i);
String scoory = db.getFormattedValue(coory.doubleValue());
x = gp.gRect1.x - gp.tickLen; // - TR.width
y = gp.gRect1.y + gp.gRect1.height - i * dely;
gp.GFV_YLABEL.outText(x, y, scoory);
// ???û???
if (coory.doubleValue() == gp.baseValue + gp.minValue) {
gp.valueBaseLine = gp.gRect1.y + gp.gRect1.height - i
* dely;
}
}
// ????????
db.drawWarnLine();
if (gp.graphTransparent) {
g.setComposite(AlphaComposite.getInstance(AlphaComposite.SRC_OVER,
0.60F));
}
/* ??X?? */
headPoint = new Point2D.Double[gp.serNum];
ArrayList cats = egp.getCategories();
int cc = cats.size();
Color c;
for (int i = 0; i < cc; i++) {
ExtGraphCategory egc = (ExtGraphCategory) cats.get(i);
double posx = DrawLine.getPosX(gp,i,cc,categorySpan,seriesWidth);
boolean valvis = (i % (gp.graphXInterval + 1) == 0);//?????Ƿ???ʾֵ????Table?ֿ?
boolean vis = valvis && !gp.isDrawTable;
if (vis) {
c = egp.getAxisColor(GraphProperty.AXIS_BOTTOM);
Utils.setStroke(g, c, Consts.LINE_SOLID, 1.0f);
db.drawLine(posx, gp.gRect1.y + gp.gRect1.height,
posx, gp.gRect1.y + gp.gRect1.height
+ gp.tickLen,c);
// ??????????
db.drawGridLineCategoryV(posx);
}
String value = egc.getNameString();
x = posx;
y = gp.gRect1.y + gp.gRect1.height + gp.tickLen; // + TR.height;
gp.GFV_XLABEL.outText(x, y, value, vis);
for (int j = 0; j < gp.serNum; j++) {
ExtGraphSery egs = egc.getExtGraphSery(gp.serNames.get(j));
if (egs.isNull()) {
continue;
}
double val = egs.getValue();
double tmp = val - gp.baseValue;
double len = dely * gp.tickNum * (tmp - gp.minValue) / (gp.maxValue * gp.coorScale);
if( gp.isDrawTable ){
posx = db.getDataTableX( i );
}
Point2D.Double endPoint;
if (egs.isNull()) {
endPoint = null;
} else {
endPoint = new Point2D.Double(posx, gp.valueBaseLine - len);
}
// ??????
if (headPoint[j] != null && endPoint != null) {
double ptx1[] = { headPoint[j].x, headPoint[j].x, endPoint.x,
endPoint.x };
double pty1[] = { headPoint[j].y, headPoint[j].y, endPoint.y,
endPoint.y };
g.setColor(db.getColor(j));
fillPolygon(db,ptx1, pty1, 4);
db.drawPolygon(ptx1, pty1, 4,
egp.getAxisColor(GraphProperty.AXIS_COLBORDER));
// ??????
double ptx2[] = { headPoint[j].x, headPoint[j].x, endPoint.x,
endPoint.x };
double pty2[] = { headPoint[j].y, gp.valueBaseLine,
gp.valueBaseLine, endPoint.y };
db.setPaint(headPoint[j].x, headPoint[j].y, endPoint.x
- headPoint[j].x, endPoint.y - gp.valueBaseLine, j,
true);
fillPolygon(db,ptx2, pty2, 4);
if (seriesWidth > 3) {
db.drawPolygon(ptx2, pty2, 4,
egp.getAxisColor(GraphProperty.AXIS_COLBORDER));
}
}
// ???????
if (gp.dispValueOntop && !egs.isNull() && valvis) {
String sval = db.getDispValue(egc,egs,gp.serNum); // getFormattedValue(val);
x = endPoint.x;
y = endPoint.y;
if (!gp.isMultiSeries) {
c = db.getColor(i);
} else {
c = db.getColor(j);
}
ValueLabel vl = new ValueLabel(sval, new Point2D.Double(x, y
- VALUE_RADIUS), c);
labelList.add(vl);
}
boolean vis2 = (i % (gp.graphXInterval + 1) == 0);
if (!egs.isNull() && gp.drawLineDot && vis2) {
double xx, yy, ww, hh;
xx = endPoint.x - VALUE_RADIUS;
yy = endPoint.y - VALUE_RADIUS;
ww = 2 * VALUE_RADIUS;
hh = ww;
if (!gp.isMultiSeries) {
db.setPaint(xx, yy, ww, hh, db.getColor(i), true);
} else {
db.setPaint(xx, yy, ww, hh, db.getColor(j), true);
}
db.fillRect(xx, yy, ww, hh);
db.drawRect(xx, yy, ww, hh,
egp.getAxisColor(GraphProperty.AXIS_COLBORDER));
db.htmlLink(xx, yy, ww, hh, htmlLink, egc.getNameString(), egs);
} // ?????ϵ?С????
headPoint[j] = endPoint;
}
}
// ??????ֵ??ǩ???????ö?
db.outLabels();
/* ?ػ?һ?»??? */
db.drawLine(gp.gRect1.x, gp.valueBaseLine, gp.gRect1.x + gp.gRect1.width,
gp.valueBaseLine, egp.getAxisColor(GraphProperty.AXIS_BOTTOM));
db.drawLine(gp.gRect1.x + gp.gRect1.width, gp.valueBaseLine,
gp.gRect1.x + gp.gRect1.width + coorWidth,
gp.valueBaseLine - coorWidth,
egp.getAxisColor(GraphProperty.AXIS_BOTTOM));
}
private static void fillPolygon(DrawBase db,double[] x, double[] y, int n) {
//?ٸĶ????룬ͬ??????Ҫ?õ???ʵ??
ExtGraphProperty egp = db.egp;
Graphics2D g = db.g;
if (egp.isDrawShade()) {
Color c = g.getColor();
Paint p = g.getPaint();
double[] xx = new double[n];
double[] yy = new double[n];
for (int i = 0; i < n; i++) {
xx[i] = x[i] + db.SHADE_SPAN;
yy[i] = y[i] + db.SHADE_SPAN;
}
g.setColor(Color.lightGray);
Utils.fillPolygon(g, xx, yy);
g.setColor(c);
g.setPaint(p);
}
Utils.fillPolygon(g,x, y);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy