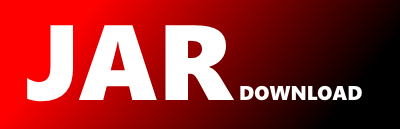
com.scudata.chart.Engine Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.chart;
import org.w3c.dom.*;
import com.scudata.app.common.*;
import com.scudata.chart.edit.*;
import com.scudata.chart.element.*;
import com.scudata.common.*;
import com.scudata.dm.*;
import com.scudata.expression.*;
import java.lang.reflect.*;
import java.io.*;
import java.util.*;
import java.awt.*;
import java.awt.image.*;
import java.awt.geom.*;
/**
* ??ͼ????
*/
public class Engine {
private ArrayList elements;
private ArrayList axisList = new ArrayList(); // ??
private ArrayList coorList = new ArrayList(); // ????ϵ
private ArrayList dataList = new ArrayList(); // ????ͼԪ
private ArrayList timeList = new ArrayList(); // ʱ????
private transient ArrayList allShapes = new ArrayList();
private transient ArrayList allLinks = new ArrayList();
private transient ArrayList allTitles = new ArrayList();
private transient ArrayList allTargets = new ArrayList();
private transient int w, h;
private transient Graphics2D g;
private transient ArrayList textAreas = new ArrayList(); // ?ı??????Ѿ?ռ?õĿռ?
private transient StringBuffer html;
private transient double t_maxDate = 0, t_minDate = Long.MAX_VALUE;// ????ʱ??????????Сʱ???
/**
* ?????ͼ????
*/
public Engine() {
}
/**
* ????ͼ??Ԫ???б?
*
* @param ies
* ??ͼԪ??
*/
public void setElements(ArrayList ies) {
elements = ies;
}
/**
* ???ÿ̶????б?
*
* @param tas
* ?̶????б?
*/
public void setAxisList(ArrayList tas) {
axisList = tas;
}
/**
* ????????ϵ?б?
*
* @param ics
* ????ϵ?б?
*/
public void setCoorList(ArrayList ics) {
coorList = ics;
}
/**
* ????????ͼԪ?б?
*
* @param des
* ????ͼԪ
*/
public void setDataList(ArrayList des) {
dataList = des;
}
/**
* ????ʱ????
*
* @param tas
* ʱ?????б?
*/
public void setTimeList(ArrayList tas) {
timeList = tas;
}
/**
* ??ȡʱ????
*
* @param name
* ??????
* @return ʱ???????
*/
public TimeAxis getTimeAxis(String name) {
for (int i = 0; i < timeList.size(); i++) {
TimeAxis axis = timeList.get(i);
if (axis.getName().equals(name)) {
return axis;
}
}
return null;
}
/**
* ??ȡ????ͼԪ??Ӧ????״?б?
*
* @return
*/
public ArrayList getShapes() {
return allShapes;
}
/**
* ??ȡ??????֡??frameCount?ĵ?frameIndex֡ͼ??ļ???????
* @param frameCount;??֡??
* @param frameIndex;?ڼ?֡????0??ʼ
* @return
*/
public Engine getFrameEngine(int frameCount, int frameIndex){
Engine e = clone();
ArrayList des = new ArrayList();
double timeSlice = (t_maxDate-t_minDate)*1f/frameCount;//ʱ???Ȱ?????֡???з?
double frameTime = t_minDate+ timeSlice*frameIndex;//???????ǰ֡????ʱ???
for(DataElement de:dataList){
String timeAxis = de.getAxisTimeName();
TimeAxis ta = e.getTimeAxis(timeAxis);
if(ta.displayMark){
// ????ʱ???
des.add( ta.getMarkElement(frameTime) );
}
if( StringUtils.isValidString(timeAxis) ){
e.elements.remove(de);//elements?Ѿ?????????ͼԪ????ʱ?????µ?????ͼԪ????Ҫ???ϵ?????ͼԪ??
des.add(de.getFrame( frameTime ) );
}else{
des.add(de);
}
}
e.setDataList(des);
return e;
}
/**
* ͼԪ?г????ӵĻ?????ȡ???????б?
*
* @return
*/
public ArrayList getLinks() {
return allLinks;
}
/**
* ????????ͼ?ε?HTML?????Ӵ???
*
* @return
*/
public String getHtmlLinks() {
return generateHyperLinks(true);
}
/**
* ??ȡ??????״shape?ı߽?????
*
* @param shape
* @return ????ε??????
*/
private ArrayList getShapeOutline(Shape shape) {
// ??ȡ??״shape??ƽ??·??
PathIterator iter = new FlatteningPathIterator(
shape.getPathIterator(new AffineTransform()), 1);
ArrayList points = new ArrayList();
float[] coords = new float[6];
while (!iter.isDone()) {
iter.currentSegment(coords);
int x = (int) coords[0];
int y = (int) coords[1];
points.add(new Point(x, y));
iter.next();
}
return points;
}
private String getRectCoords(Rectangle rect) {
int x, y, w, h;
x = rect.x;
y = rect.y;
w = rect.width;
h = rect.height;
// ?????Ӵ???
int minimum = 10;
if (w < minimum) {
w = minimum;
}
if (h < minimum) {
h = minimum;
}
String coords = x + "," + y + "," + (x + w) + "," + (y + h);
return coords;
}
private String getPolyCoords(Shape shape) {
StringBuffer buf = new StringBuffer();
ArrayList polyPoints = getShapeOutline(shape);
for (int i = 0; i < polyPoints.size(); i++) {
Point p = polyPoints.get(i);
if (i > 0) {
buf.append(",");
}
buf.append(p.getX() + "," + p.getY());
}
return buf.toString();
}
private String dealSpecialChar(String str) {
// ??????Ÿ?Ϊ?ϲ㴦?????˴????ٴ???
return str;
}
private String getLinkHtml(String link, String shape, String coords,
String title, Object target) {
StringBuffer sb = new StringBuffer(128);
sb.append("\n");
return sb.toString();
}
// svgû????ʾ??Ϣ???????????title??????Ч??
private String getLinkSvg(String link, String shape, String coords,
String title, Object target) {
StringBuffer sb = new StringBuffer(128);
link = dealSpecialChar(link);
sb.append("\n");
sb.append("<");
sb.append(shape);
sb.append(" ");
sb.append(coords);
sb.append("/>\n");
sb.append("");
return sb.toString();
}
/**
* ????isHtml????Html?Ļ???svg?ij?????
*
* @param isHtml
* ???淵??html?ij????Ӵ???????Ϊsvgͼ?ε????Ӵ?
* @return
*/
private String generateHyperLinks(boolean isHtml) {
if (allLinks.isEmpty())
return null;
StringBuffer buf = new StringBuffer();
String link, shape, coords, target, title;
for (int i = 0; i < allShapes.size(); i++) {
link = allLinks.get(i);
Shape s = allShapes.get(i);
target = allTargets.get(i);
title = allTitles.get(i);
if (isHtml) {
if (s instanceof Rectangle) {
shape = "rect";
coords = getRectCoords((Rectangle) s);
} else {// if(s instanceof Polygon){
shape = "poly";
coords = getPolyCoords(s);
}
buf.append(getLinkHtml(link, shape, coords, title, target));
} else {// svg
String style = " style=\"fill-opacity:0;stroke-width:0\"";
if (s instanceof Rectangle) {
Rectangle r = (Rectangle) s;
shape = "rect";
coords = "x=\"" + r.x + "\" y=\"" + r.y + "\" width=\""
+ r.width + "\" height=\"" + r.height + "\""
+ style;
} else {// if(s instanceof Polygon){
shape = "polygon";
coords = "points=\"" + getPolyCoords(s) + "\"" + style;
}
buf.append(getLinkSvg(link, shape, coords, title, target));
}
}
return buf.toString();
}
/**
* Ϊ?˷?ֹͼԪ?ص??????ƹ?????ÿ???????ͼԪλ?ö??Ỻ?? ?÷????????ж?ָ???ľ???λ??rect?Ƿ???Ѿ??????ͼ??Ԫ?????ཻ
*
* @param rect
* ָ???ľ???λ??
* @return ??????ཻʱ????true??????false
*/
public boolean intersectTextArea(Rectangle rect) {
int size = textAreas.size();
for (int i = 0; i < size; i++) {
Rectangle tmp = (Rectangle) textAreas.get(i);
if (tmp.intersects(rect)) {
return true;
}
}
return false;
}
/**
* ÿ????һ??ͼԪ????Ҫ????ǰͼԪ??????λ????Ϣ???ӵ????滺??
*
* @param rect
* ͼԪ??Ӧ?ľ???????λ??
*/
public void addTextArea(Rectangle rect) {
textAreas.add(rect);
}
private IElement getElement(Sequence chartParams) {
ChartParam cp = (ChartParam) chartParams.get(1);
ElementInfo ei = ElementLib.getElementInfo(cp.getName());
if (ei == null) {
throw new RuntimeException("Unknown chart element: " + cp.getName());
}
ObjectElement oe = ei.getInstance();
oe.loadProperties(chartParams);
return oe;
}
/**
* ?ö???õ?ͼԪ???й????ͼ????
*
* @param chartElements
* ͼ??Ԫ??????
*/
public Engine(Sequence chartElements) {
int size = chartElements.length();
this.elements = new ArrayList();
for (int i = 1; i <= size; i++) {
Sequence chartParams = (Sequence) chartElements.get(i);
IElement e = getElement(chartParams);
this.elements.add(e);
}
prepare();
}
/**
* ??ȡ?̶????б?
*
* @return ?̶???
*/
public ArrayList getAxisList() {
return axisList;
}
/**
* ??ȡ????ͼԪ?б?
*
* @return ????ͼԪ
*/
public ArrayList getDataList() {
return dataList;
}
/**
* ??ȡ????ϵ?б???һ??????????Բ??ö??????ϵ???Ա???????ͼ??
*
* @return ????ϵ
*/
public ArrayList getCoorList() {
return coorList;
}
/**
* ???ݿ̶???????ƻ?ȡ??Ӧ?Ŀ̶??????
*
* @param name
* ?̶???????
* @return ?̶??????
*/
public TickAxis getAxisByName(String name) {
for (int i = 0; i < axisList.size(); i++) {
TickAxis axis = axisList.get(i);
if (axis.getName().equals(name)) {
return axis;
}
}
return null;
}
public IMapAxis getMapAxisByName(String name) {
for (int i = 0; i < elements.size(); i++) {
IElement e = elements.get(i);
if (e instanceof IMapAxis) {
IMapAxis ma = (IMapAxis) e;
if (ma.getName().equals(name)) {
return ma;
}
}
}
return null;
}
private ArrayList getDataElementsOnAxis(String axis) {
ArrayList al = new ArrayList();
int size = dataList.size();
for (int i = 0; i < size; i++) {
DataElement de = dataList.get(i);
if (de.isPhysicalCoor()) {
continue;
}
if (de.getAxis1Name().equals(axis)
|| de.getAxis2Name().equals(axis)) {
al.add(de);
}
}
return al;
}
private ArrayList getDataElementsOnTime(String axis) {
ArrayList al = new ArrayList();
int size = dataList.size();
for (int i = 0; i < size; i++) {
DataElement de = dataList.get(i);
if (de.getAxisTimeName().equals(axis)) {
al.add(de);
}
}
return al;
}
private void prepare() {
// ֻ???Ƿ????ͼʱ??ֻ??һ??ͼ??????????????ͼ??ֵ???????Զ???ö?????ȡͼ??ֵ??
ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy