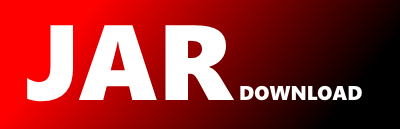
com.scudata.dm.DfxManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.dm;
import java.lang.ref.SoftReference;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import com.scudata.cellset.datamodel.PgmCellSet;
import com.scudata.expression.Expression;
/**
* dfx?????????
*/
public class DfxManager {
private static DfxManager dfxManager = new DfxManager();
private HashMap> dfxRefMap =
new HashMap>();
private HashMap>> expListMap =
new HashMap>>();
private DfxManager() {}
/**
* ȡdfx?????????ʵ??
* @return DfxManager
*/
public static DfxManager getInstance() {
return dfxManager;
}
/**
* ???????ij?????
*/
public void clear() {
synchronized(dfxRefMap) {
dfxRefMap.clear();
}
}
/**
* ʹ????dfx???????????????
* @param dfx PgmCellSet
*/
public void putDfx(PgmCellSet dfx) {
Context dfxCtx = dfx.getContext();
dfxCtx.setParent(null);
dfxCtx.setJobSpace(null);
dfx.reset();
synchronized(dfxRefMap) {
dfxRefMap.put(dfx.getName(), new SoftReference(dfx));
}
}
/**
* ?ӻ??????????ȡdfx??ʹ???????Ҫ????putDfx?????????????
* @param name dfx?ļ???
* @param ctx ??????????
* @return PgmCellSet
*/
public PgmCellSet removeDfx(String name, Context ctx) {
PgmCellSet dfx = null;
synchronized(dfxRefMap) {
SoftReference sr = dfxRefMap.remove(name);
if (sr != null) dfx = (PgmCellSet)sr.get();
}
if (dfx == null) {
return readDfx(name, ctx);
} else {
// ???ٹ???ctx?еı???
Context dfxCtx = dfx.getContext();
dfxCtx.setEnv(ctx);
return dfx;
}
}
/**
* ?ӻ??????????ȡdfx??ʹ???????Ҫ????putDfx?????????????
* @param fo dfx?ļ?????
* @param ctx ??????????
* @return PgmCellSet
*/
public PgmCellSet removeDfx(FileObject fo, Context ctx) {
PgmCellSet dfx = null;
String name = fo.getFileName();
synchronized(dfxRefMap) {
SoftReference sr = dfxRefMap.remove(name);
if (sr != null) dfx = (PgmCellSet)sr.get();
}
if (dfx == null) {
return readDfx(fo, ctx);
} else {
// ???ٹ???ctx?еı???
Context dfxCtx = dfx.getContext();
dfxCtx.setEnv(ctx);
return dfx;
}
}
/**
* ??ȡdfx??????ʹ?û???
* @param fo dfx?ļ?????
* @param ctx ??????????
* @return PgmCellSet
*/
public PgmCellSet readDfx(FileObject fo, Context ctx) {
PgmCellSet dfx = fo.readPgmCellSet();
dfx.resetParam();
// ???ٹ???ctx?еı???
Context dfxCtx = dfx.getContext();
dfxCtx.setEnv(ctx);
return dfx;
}
/**
* ??ȡdfx??????ʹ?û???
* @param name dfx?ļ???
* @param ctx ??????????
* @return PgmCellSet
*/
public PgmCellSet readDfx(String name, Context ctx) {
return readDfx(new FileObject(name, null, "s", ctx), ctx);
}
/**
* ȡ????ı???ʽ??????ʽ?????????Ҫ????putExpression?????黹????
* @param strExp ????ʽ??
* @param ctx ??????????
* @return Expression
*/
public Expression getExpression(String strExp, Context ctx) {
synchronized(expListMap) {
SoftReference> ref = expListMap.get(strExp);
if (ref != null) {
List expList = ref.get();
if (expList != null && expList.size() > 0) {
Expression exp = expList.remove(expList.size() - 1);
exp.reset();
return exp;
}
}
}
return new Expression(ctx, strExp);
}
/**
* ????ʽ??????ɺ?ѱ???ʽ????????
* @param strExp ????ʽ??
* @param exp ????ʽ
*/
public void putExpression(String strExp, Expression exp) {
synchronized(expListMap) {
SoftReference> ref = expListMap.get(strExp);
if (ref == null) {
List expList = new ArrayList();
expList.add(exp);
ref = new SoftReference>(expList);
expListMap.put(strExp, ref);
} else {
List expList = ref.get();
if (expList == null) {
expList = new ArrayList();
expList.add(exp);
ref = new SoftReference>(expList);
expListMap.put(strExp, ref);
} else {
expList.add(exp);
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy