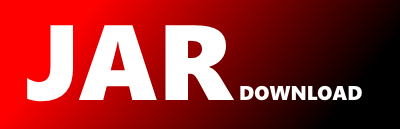
com.scudata.dm.ResourceManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.dm;
import java.util.ArrayList;
import com.scudata.parallel.IProxy;
public final class ResourceManager {
private ArrayList resources = new ArrayList();
private ArrayList proxys = new ArrayList();
public void add(IResource resource) {
synchronized(resources) {
resources.add(resource);
}
}
public void remove(IResource resource) {
synchronized(resources) {
resources.remove(resource);
}
}
public void closeResource() {
ArrayList resources = this.resources;
synchronized(resources) {
for (int i = resources.size() - 1; i >= 0; --i) {
try {
IResource resource = resources.get(i);
resource.close();
} catch (Exception e) {
}
}
resources.clear();
}
}
public void addProxy(IProxy proxy) {
synchronized(proxys) {
proxys.add(proxy);
}
}
public IProxy getProxy(int proxyId) {
ArrayList proxys = this.proxys;
synchronized(proxys) {
for (IProxy proxy : proxys) {
if (proxy.getProxyId() == proxyId) {
return proxy;
}
}
}
return null;
}
public void closeProxy() {
ArrayList proxys = this.proxys;
synchronized(proxys) {
for (IProxy proxy : proxys) {
proxy.close();
}
proxys.clear();
}
}
public boolean closeProxy(int proxyId) {
ArrayList proxys = this.proxys;
synchronized(proxys) {
for (int i = proxys.size() - 1; i >= 0; --i) {
IProxy proxy = proxys.get(i);
if (proxy.getProxyId() == proxyId) {
proxy.close();
proxys.remove(i);
return true;
}
}
}
return false;
}
public void close() {
closeProxy();
closeResource();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy