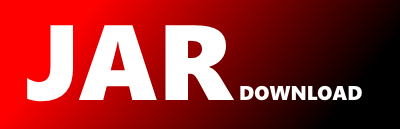
com.scudata.dw.pseudo.Pseudo Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.dw.pseudo;
import java.util.ArrayList;
import java.util.List;
import com.scudata.common.MessageManager;
import com.scudata.common.RQException;
import com.scudata.dm.BaseRecord;
import com.scudata.dm.Context;
import com.scudata.dm.DataStruct;
import com.scudata.dm.IndexTable;
import com.scudata.dm.Sequence;
import com.scudata.dm.cursor.ICursor;
import com.scudata.dm.op.Operable;
import com.scudata.dm.op.Operation;
import com.scudata.dm.op.Select;
import com.scudata.dm.op.Switch;
import com.scudata.expression.Expression;
import com.scudata.expression.operator.And;
import com.scudata.resources.EngineMessage;
import com.scudata.util.Variant;
public class Pseudo extends IPseudo {
protected PseudoDefination pd;//ʵ???Ķ???
protected Sequence cache = null;//??import?Ľ????cache
//?????α???Ҫ?IJ???
protected String []names;
protected Expression []exps;
protected Context ctx;
protected ArrayList opList;
protected Expression filter;
protected ArrayList fkNameList;
protected ArrayList codeList;
protected ArrayList optList;
protected ArrayList extraNameList;//??Ϊ???˻?α?ֶζ???Ҫ????ȡ?????ֶ???
protected ArrayList allNameList;//ʵ?????????ֶ?
protected String []deriveNames;//??derive???ӵ?
protected Expression []deriveExps;
public void addColName(String name) {
throw new RQException("never run to here");
}
public void addPKeyNames() {
throw new RQException("never run to here");
}
public Operable addOperation(Operation op, Context ctx) {
IPseudo newObj = null;
try {
newObj = (IPseudo) ((IPseudo)this).clone(ctx);
((Pseudo) newObj).addOpt(op, ctx);
} catch (CloneNotSupportedException e) {
throw new RQException(e);
}
return (Operable) newObj;
}
public void addOpt(Operation op, Context ctx) {
if (opList == null) {
opList = new ArrayList();
}
if (op != null) {
ArrayList tempList = new ArrayList();
new Expression(op.getFunction()).getUsedFields(ctx, tempList);
boolean isBFile = pd.isBFile();
if (!isBFile && op instanceof Select && op.getFunction() != null) {
Expression exp = ((Select)op).getFilterExpression();
boolean flag = true;//true??ʾ???DZ??????ֶΡ? ????news??new??derive
for (String name : tempList) {
if (!isColumn(name)) {
flag = false;
break;
}
}
if (flag) {
if (filter == null) {
filter = exp;
} else {
And and = new And();
and.setLeft(filter.getHome());
and.setRight(exp.getHome());
filter = new Expression(and);
}
} else {
for (String name : tempList) {
addColName(name);
}
opList.add(op);
}
} else if (!isBFile && op instanceof Switch && ((Switch) op).isIsect()) {
//??switch@iת??ΪF:K
//ת???????????ֶΡ??????ֶδ?????????????code??????????codeû????????
String names[] = ((Switch) op).getFkNames();
Sequence codes[] = ((Switch) op).getCodes();
//????Ƿ?????????????
boolean flag = true;
while (true) {
if (1 != names.length) break;//???ǵ??ֶ?
String keyName;
if (((Switch) op).getExps()[0] == null) {
keyName = codes[0].dataStruct().getPrimary()[0];
} else {
keyName = ((Switch) op).getExps()[0].getIdentifierName();
}
Object obj = codes[0].ifn();
if (obj instanceof BaseRecord) {
DataStruct ds = ((BaseRecord)obj).dataStruct();
if (-1 == ds.getFieldIndex(keyName)) {
//code?ﲻ???ڸ??ֶ?
MessageManager mm = EngineMessage.get();
throw new RQException(keyName + mm.getMessage("ds.fieldNotExist"));
}
int []fields = ds.getPKIndex();
if (fields == null) {
break;//??????????
} else {
if (! ds.getPrimary()[0].equals(keyName)) {
break;//???ֶβ???code??????
}
}
} else {
MessageManager mm = EngineMessage.get();
throw new RQException(mm.getMessage("engine.needPmt"));
}
IndexTable it = codes[0].getIndexTable();
if (it != null) {
break;//??????????Ҳ??ת??
}
flag = false;
if (codeList == null) {
codeList = new ArrayList();
}
if (fkNameList == null) {
fkNameList = new ArrayList();
}
for (String name : names) {
fkNameList.add(name);
}
for (Sequence seq : codes) {
codeList.add(seq);
}
break;
}
if (flag) {
//??????ȡΪF:T
for (String name : tempList) {
addColName(name);
}
opList.add(op);
}
} else {
for (String name : tempList) {
addColName(name);
}
opList.add(op);
}
}
this.ctx = ctx;
}
//????news??new??derive
protected void setFetchInfo(ICursor cursor, Expression []exps, String []names) {
if (this.exps != null) return;
if (exps == null && extraNameList.size() == 0) {
//???û??ָ??ȡ???ֶΣ?????????֯
names = cursor.getDataStruct().getFieldNames();
for (String name : names) {
if (!extraNameList.contains(name)) {
extraNameList.add(name);
}
}
int size = extraNameList.size();
names = new String[size];
extraNameList.toArray(names);
exps = new Expression[size];
for (int i = 0; i < size; i++) {
exps[i] = new Expression(names[i]);
}
this.exps = exps;
this.names = names;
} else {
//???ָ????ȡ???ֶΣ?ҲҪ?Ѷ????õ????ֶμ???
//???extraNameList???Ƿ????exps????ֶ?
//????У???ȥ??
ArrayList tempList = new ArrayList();
for (String name : extraNameList) {
if (!tempList.contains(name)) {
tempList.add(name);
}
}
if (exps != null) {
for (Expression exp : exps) {
String expName = exp.getIdentifierName();
if (tempList.contains(expName)) {
tempList.remove(expName);
}
}
}
ArrayList tempNameList = new ArrayList();
ArrayList tempExpList = new ArrayList();
if (exps != null) {
for (Expression exp : exps) {
tempExpList.add(exp);
}
if (names == null) {
for (Expression exp : exps) {
tempNameList.add(exp.getIdentifierName());
}
} else {
for (String name : names) {
tempNameList.add(name);
}
}
}
for (String name : tempList) {
tempExpList.add(new Expression(name));
tempNameList.add(name);
}
int size = tempExpList.size();
this.exps = new Expression[size];
tempExpList.toArray(this.exps);
this.names = new String[size];
tempNameList.toArray(this.names);
}
// //?????derive??????exps??names
// if (deriveExps != null) {
// int oldSize = this.exps.length;
// int newSize = deriveExps.length + oldSize;
//
// Expression []newExps = new Expression[newSize];
// String []newNames = new String[newSize];
// System.arraycopy(this.exps, 0, newExps, 0, oldSize);
// System.arraycopy(deriveExps, 0, newExps, oldSize, deriveExps.length);
// System.arraycopy(this.names, 0, newNames, 0, oldSize);
// System.arraycopy(deriveNames, 0, newNames, oldSize, deriveExps.length);
//
// this.exps = newExps;
// this.names = newNames;
// }
}
public boolean isColumn(String col) {
return allNameList.contains(col);
}
public Context getContext() {
return ctx;
}
public void cloneField(Pseudo obj) {
//obj.table = table;
obj.pd = new PseudoDefination(getPd());
obj.ctx = ctx;
obj.names = names == null ? null : names.clone();
obj.exps = exps == null ? null : exps.clone();
obj.filter = filter == null ? null : filter.newExpression(ctx);
if (opList != null) {
obj.opList = new ArrayList();
for (Operation op : opList) {
obj.opList.add(op.duplicate(ctx));
}
}
if (fkNameList != null) {
obj.fkNameList = new ArrayList();
for (String str : fkNameList) {
obj.fkNameList.add(str);
}
}
if (codeList != null) {
obj.codeList = new ArrayList();
for (Sequence seq : codeList) {
obj.codeList.add(seq);
}
}
if (optList != null) {
obj.optList = new ArrayList();
for (String str : optList) {
obj.optList.add(str);
}
}
if (extraNameList != null) {
obj.extraNameList = new ArrayList();
for (String str : extraNameList) {
obj.extraNameList.add(str);
}
}
if (allNameList != null) {
obj.allNameList = new ArrayList();
for (String str : allNameList) {
obj.allNameList.add(str);
}
}
obj.deriveNames = deriveNames == null ? null : deriveNames.clone();
obj.deriveExps = deriveExps == null ? null : deriveExps.clone();
}
public PseudoDefination getPd() {
return pd;
}
public void append(ICursor cursor, String option) {
throw new RQException("never run to here");
}
public Sequence update(Sequence data, String opt) {
throw new RQException("never run to here");
}
public Sequence delete(Sequence data, String opt) {
throw new RQException("never run to here");
}
public void addColNames(String[] nameArray) {
throw new RQException("never run to here");
}
public ICursor cursor(Expression[] exps, String[] names) {
throw new RQException("never run to here");
}
public ICursor cursor(Expression[] exps, String[] names, boolean isColumn) {
throw new RQException("never run to here");
}
public Object clone(Context ctx) throws CloneNotSupportedException {
throw new RQException("never run to here");
}
public void setCache(Sequence cache) {
this.cache = cache;
}
public Sequence getCache() {
return cache;
}
// ?ж??Ƿ???????ָ?????Ƶ????
public boolean hasForeignKey(String fkName) {
PseudoColumn column = pd.findColumnByName(fkName);
if (column != null && column.getDim() != null)
return true;
else
return false;
}
// ȡ???????
public String[] getPrimaryKey() {
return getPd().getAllSortedColNames();
}
//?????ֶ?????ά??
public PseudoColumn getFieldSwitchColumnByName(String fieldName) {
List columns = pd.getColumns();
if (columns == null) {
return null;
}
for (PseudoColumn column : columns) {
if (column.getDim() != null) {
if (column.getName() != null && column.getName().equals(fieldName)) {
return column;
}
}
}
return null;
}
// ȡ?ֶ?ָ???Column
public PseudoColumn getFieldSwitchColumn(String fieldName) {
List columns = pd.getColumns();
if (columns == null) {
return null;
}
for (PseudoColumn column : columns) {
if (column.getDim() != null) {
if (column.getFkey() == null && column.getName().equals(fieldName)) {
return column;
} else if (column.getFkey() != null
&& column.getFkey().length == 1
&& column.getFkey()[0].equals(fieldName)) {
return column;
}
}
}
return null;
}
public List getFieldSwitchColumns(String[] fieldNames) {
List list = new ArrayList();
if (fieldNames == null) {
List columns = pd.getColumns();
if (columns == null) {
return null;
}
for (PseudoColumn column : columns) {
if (column.getDim() != null) {
list.add(column);
}
}
} else {
for (String fieldName : fieldNames) {
PseudoColumn column = getFieldSwitchColumn(fieldName);
if (column != null) {
list.add(column);
}
}
}
if (list.size() == 0)
return null;
else
return list;
}
// ȡ?ֶ???switchָ???????????û???ؿ?
public Pseudo getFieldSwitchTable(String fieldName) {
List columns = pd.getColumns();
if (columns == null) {
return null;
}
for (PseudoColumn column : columns) {
if (column.getDim() != null) {
if (column.getFkey() == null && column.getName().equals(fieldName)) {
return (PseudoTable) column.getDim();
}
// else if (column.getFkey() != null
// && column.getFkey().length > 0
// && column.getFkey()[0].equals(fieldName)) {
// return (PseudoTable) column.getDim();
// }
}
}
return null;
}
/**
* ???????
* @param fkName ?????
* @param fieldNames ????ֶ?
* @param code ???
* @param clone ????һ???????
* @return
*/
public Pseudo addForeignKeys(String fkName, String []fieldNames, Object code, String[] codeKeys, boolean clone) {
throw new RQException("Never run to here.");
}
public Pseudo setPathCount(int pathCount) {
throw new RQException("Never run to here.");
}
public Pseudo setMcsTable(Pseudo mcsTable) {
throw new RQException("Never run to here.");
}
public Sequence Import(Expression[] exps, String[] names) {
return cursor(exps, names).fetch();
}
/**
* ?ж??Ƿ??????????
* @param fkName
* @param fieldNames
* @param code
* @param codeKeys
* @return
*/
public PseudoColumn isForeignKey(String fkName, String []fieldNames, Object code, String[] codeKeys) {
List columns = pd.getColumns();
if (columns == null) {
return null;
}
for (PseudoColumn column : columns) {
if (column.getDim() != null) {
if (column.getName() != null && column.getName().equals(fkName)) {
return column;
}
if (column.getFkey() != null
&& column.getFkey().length == 1
&& column.getFkey()[0].equals(fkName)) {
return column;
}
if ((column.getFkey() != null)
&& Variant.compareArrays(fieldNames, column.getFkey()) == 0) {
return column;
}
if ((column.getDimKey() != null)
&& Variant.compareArrays(codeKeys, column.getDimKey()) == 0) {
return column;
}
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy