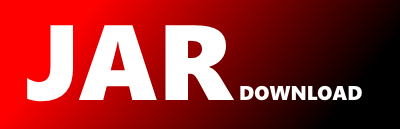
com.scudata.dw.pseudo.PseudoTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.dw.pseudo;
import java.io.IOException;
import java.util.ArrayList;
import java.util.List;
import com.scudata.common.RQException;
import com.scudata.common.Types;
import com.scudata.dm.BaseRecord;
import com.scudata.dm.Context;
import com.scudata.dm.DataStruct;
import com.scudata.dm.Sequence;
import com.scudata.dm.cursor.ConjxCursor;
import com.scudata.dm.cursor.ICursor;
import com.scudata.dm.cursor.MemoryCursor;
import com.scudata.dm.cursor.MergeCursor;
import com.scudata.dm.cursor.MultipathCursors;
import com.scudata.dm.op.Conj;
import com.scudata.dm.op.Group;
import com.scudata.dm.op.Join;
import com.scudata.dm.op.New;
import com.scudata.dm.op.Operable;
import com.scudata.dm.op.Operation;
import com.scudata.dm.op.Select;
import com.scudata.dm.op.Switch;
import com.scudata.dw.ColPhyTable;
import com.scudata.dw.Cursor;
import com.scudata.dw.IFilter;
import com.scudata.dw.IPhyTable;
import com.scudata.dw.RowCursor;
import com.scudata.dw.RowPhyTable;
import com.scudata.dw.PhyTable;
import com.scudata.expression.Constant;
import com.scudata.expression.Expression;
import com.scudata.expression.IParam;
import com.scudata.expression.Node;
import com.scudata.expression.ParamParser;
import com.scudata.expression.UnknownSymbol;
import com.scudata.expression.VarParam;
import com.scudata.expression.mfn.sequence.Contain;
import com.scudata.expression.operator.And;
import com.scudata.expression.operator.DotOperator;
import com.scudata.expression.operator.Equals;
import com.scudata.expression.operator.NotEquals;
import com.scudata.expression.operator.Or;
public class PseudoTable extends Pseudo {
//?????α???Ҫ?IJ???
protected String []fkNames;
protected Sequence []codes;
protected int pathCount;
protected ArrayList extraOpList = new ArrayList();//??????????????ӳټ??㣨????????????select???ӣ?
protected ArrayList joinColumnList = new ArrayList();//?????????
protected PseudoTable mcsTable;
protected boolean hasPseudoColumns = false;//?Ƿ???Ҫ????α?ֶ?ת????ö?١???ֵ??????ʽ
public PseudoTable() {
}
/**
* ???????????
* @param rec ?????¼
* @param hs ?ֻ?????
* @param n ??????
* @param ctx
*/
public PseudoTable(BaseRecord rec, int n, Context ctx) {
pd = new PseudoDefination(rec, ctx);
pathCount = n;
this.ctx = ctx;
extraNameList = new ArrayList();
init();
}
public PseudoTable(PseudoDefination pd, int n, Context ctx) {
this.pd = pd;
pathCount = n;
this.ctx = ctx;
extraNameList = new ArrayList();
init();
}
public PseudoTable(BaseRecord rec, PseudoTable mcs, Context ctx) {
this(rec, 0, ctx);
mcsTable = mcs;
}
public static PseudoTable create(BaseRecord rec, int n, Context ctx) {
PseudoDefination pd = new PseudoDefination(rec, ctx);
if (pd.isBFile()) {
return new PseudoBFile(pd, n, ctx);
} else {
return new PseudoTable(pd, n, ctx);
}
}
protected void init() {
if (getPd() != null) {
allNameList = new ArrayList();
String []names = getPd().getAllColNames();
for (String name : names) {
allNameList.add(name);
}
if (getPd().getColumns() != null) {
List columns = getPd().getColumns();
for (PseudoColumn column : columns) {
//???????ö??α?ֶκͶ?ֵα?ֶΣ?Ҫ??¼???????ڽ??????Ĵ????л??õ?
if (column.getPseudo() != null) {
hasPseudoColumns = true;
}
if (column.getBits() != null) {
hasPseudoColumns = true;
}
if (column.getDim() != null) {
if (column.getFkey() == null) {
addColName(column.getName());
} else {
for (String key : column.getFkey()) {
addColName(key);
}
}
Sequence dim = (Sequence) column.getDim();
if (column.getTkey() != null && dim.dataStruct().getTimeKeyCount() > 0) {
addColName(column.getTkey());
}
}
}
}
}
}
public void addPKeyNames() {
addColNames(getPd().getAllSortedColNames());
}
public void addColNames(String []nameArray) {
for (String name : nameArray) {
addColName(name);
}
}
public void addColName(String name) {
if (name == null) return;
if (allNameList.contains(name) && !extraNameList.contains(name)) {
extraNameList.add(name);
}
}
/**
* ????ȡ???ֶ?
* @param exps ȡ??????ʽ
* @param fields ȡ??????
*/
protected void setFetchInfo(Expression []exps, String []fields) {
this.exps = null;
this.names = null;
boolean needNew = extraNameList.size() > 0;
Expression newExps[] = null;
extraOpList.clear();
joinColumnList.clear();
//set FK codes info
if (fkNameList != null) {
int size = fkNameList.size();
fkNames = new String[size];
fkNameList.toArray(fkNames);
codes = new Sequence[size];
codeList.toArray(codes);
}
if (exps == null) {
if (fields == null) {
return;
} else {
int len = fields.length;
exps = new Expression[len];
for (int i = 0; i < len; i++) {
exps[i] = new Expression(fields[i]);
}
}
}
newExps = exps.clone();//????һ??
/**
* ??ȡ??????ʽҲ??ȡ???ֶ?,????extraNameList???Ƿ????exps????ֶ?
* ?????????ȥ??
*/
ArrayList tempList = new ArrayList();
for (String name : extraNameList) {
if (!tempList.contains(name)) {
tempList.add(name);
}
}
for (Expression exp : exps) {
String expName = exp.getIdentifierName();
if (tempList.contains(expName)) {
tempList.remove(expName);
}
}
ArrayList tempNameList = new ArrayList();
ArrayList tempExpList = new ArrayList();
int size = exps.length;
for (int i = 0; i < size; i++) {
Expression exp = exps[i];
String name = fields[i];
Node node = exp.getHome();
if (node instanceof UnknownSymbol || node instanceof VarParam) {
String expName = exp.getIdentifierName();
if (!allNameList.contains(expName)) {
/**
* ?????α?ֶ?????ת??
*/
PseudoColumn col = pd.findColumnByPseudoName(expName);
if (col != null) {
if (col.getDim() != null) {
if (col.getFkey() == null) {
String colName = col.getName();
if (!tempNameList.contains(colName)) {
tempExpList.add(new Expression(colName));
tempNameList.add(colName);
}
} else {
for (String colName : col.getFkey()) {
if (!tempNameList.contains(colName)) {
tempExpList.add(new Expression(colName));
tempNameList.add(colName);
}
}
}
joinColumnList.add(col);
} else if (col.getExp() != null) {
//?б???ʽ??α??
newExps[i] = new Expression(col.getExp());
needNew = true;
ArrayList list = new ArrayList();
newExps[i].getUsedFields(ctx, list);
for(String field : list) {
if (!tempNameList.contains(field)) {
tempExpList.add(new Expression(field));
tempNameList.add(field);
}
}
} else if (col.get_enum() != null) {
/**
* ö???ֶ???ת??
*/
String var = "pseudo_enum_value_" + i;
ctx.setParamValue(var, col.get_enum());
name = col.getName();
newExps[i] = new Expression(var + "(" + name + ")");
exp = new Expression(name);
needNew = true;
if (!tempNameList.contains(name)) {
tempExpList.add(exp);
tempNameList.add(name);
}
} else if (col.getBits() != null) {
/**
* ??ֵ?ֶ???ת??
*/
name = col.getName();
String pname = ((UnknownSymbol) node).getName();
Sequence seq;
seq = col.getBits();
int idx = seq.firstIndexOf(pname) - 1;
int bit = 1 << idx;
String str = "and(" + col.getName() + "," + bit + ")!=0";//??Ϊ???ֶε?λ????
newExps[i] = new Expression(str);
exp = new Expression(name);
needNew = true;
tempExpList.add(exp);
tempNameList.add(name);
}
}
} else {
if (!tempNameList.contains(name)) {
tempExpList.add(exp);
tempNameList.add(name);
}
}
// } else if (node instanceof DotOperator) {
// Node left = node.getLeft();
// if (left != null && left instanceof UnknownSymbol) {
// PseudoColumn col = getPd().findColumnByName( ((UnknownSymbol)left).getName());
// if (col != null) {
// Derive derive = new Derive(new Expression[] {exp}, new String[] {name}, null);
// extraOpList.add(derive);
// }
// }
}
}
//???????date?????ȡ?????ֶ?ugrp
String date = pd.getDate();
String ugrp = pd.getUgrp();
if (date != null && !tempNameList.contains(ugrp)) {
needNew = true;
tempList.add(ugrp);
}
for (String name : tempList) {
tempExpList.add(new Expression(name));
tempNameList.add(name);
}
size = tempExpList.size();
this.exps = new Expression[size];
tempExpList.toArray(this.exps);
this.names = new String[size];
tempNameList.toArray(this.names);
if (needNew) {
New _new = new New(newExps, fields, null);
extraOpList.add(_new);
}
return;
}
public String[] getFetchColNames(String []fields) {
ArrayList tempList = new ArrayList();
if (fields != null) {
for (String name : fields) {
tempList.add(name);
}
}
for (String name : extraNameList) {
if (!tempList.contains(name)) {
tempList.add(name);
}
}
int size = tempList.size();
if (size == 0) {
return null;
}
String []newFields = new String[size];
tempList.toArray(newFields);
return newFields;
}
/**
* ?õ??????ÿ??ʵ??????α깹?ɵ?????
* @return
*/
public ICursor[] getCursors(boolean isColumn) {
List tables = getPd().getTables();
int size = tables.size();
ICursor cursors[] = new ICursor[size];
for (int i = 0; i < size; i++) {
cursors[i] = getCursor(tables.get(i), null, true, isColumn);
}
return cursors;
}
/**
* ???ӿ????õ??????????
*/
protected void addJoin(ICursor cursor) {
List list = getFieldSwitchColumns(this.names);
if (list != null) {
for (PseudoColumn col : joinColumnList) {
list.add(col);
}
} else {
list = joinColumnList;
}
if (getPd() != null && list != null) {
for (PseudoColumn column : list) {
if (column.getDim() != null) {//??????????????????һ??switch???ӳټ???
Sequence dim;
if (column.getDim() instanceof Sequence) {
dim = (Sequence) column.getDim();
} else {
dim = ((IPseudo) column.getDim()).cursor(null, null, false).fetch();
}
boolean hasTimeKey = column.getTkey() != null && dim.dataStruct().getTimeKeyCount() == 1;
String fkey[] = column.getFkey();
if (fkey == null) {
/**
* ??ʱname????????ֶ?
*/
String[] fkNames = new String[] {column.getName()};
String[] timeFkNames =hasTimeKey ? new String[] {column.getTkey()} : null;
Sequence[] codes = new Sequence[] {dim};
Switch s = new Switch(
null,
fkNames,
timeFkNames,
codes,
null,
null,
null);
cursor.addOperation(s, ctx);
} else {
int size = fkey.length;
/**
* ?????????ʱ???ֶ?,?Ͱ?ʱ???ֶ?ƴ?ӵ?fkeyĩβ
*/
if (hasTimeKey) {
size++;
fkey = new String[size];
System.arraycopy(column.getFkey(), 0, fkey, 0, size - 1);
fkey[size - 1] = column.getTkey();
}
Expression[][] exps = new Expression[1][];
exps[0] = new Expression[size];
for (int i = 0; i < size; i++) {
exps[0][i] = new Expression(fkey[i]);
}
Expression[][] newExps = new Expression[1][];
newExps[0] = new Expression[] {new Expression("~")};
String newName = column.getName();
if (newName == null && column.getFkey() != null) {
newName = column.getFkey()[0];
}
String[][] newNames = new String[1][];
newNames[0] = new String[] {newName};
Expression[][] dimKeyExps = new Expression[1][];
String[] dimKey = column.getDimKey();
if (dimKey == null) {
dimKeyExps[0] = null;
} else {
Expression[] dimKeyExp = new Expression[size];
for (int i = 0; i < size; i++) {
dimKeyExp[i] = new Expression(dimKey[i]);
}
dimKeyExps[0] = dimKeyExp;
}
Join join = new Join(null, null, exps, new Sequence[] {dim}, dimKeyExps, newExps, newNames, null);
cursor.addOperation(join, ctx);
}
}
}
}
}
/**
* ?õ?table???α?
* @param table
* @param mcs
* @param addOpt ?Ƿ?Ѹ??Ӽ???????
* @param isColumn ?Ƿ???ʽ?α?
* @return
*/
private ICursor getCursor(IPhyTable table, ICursor mcs, boolean addOpt, boolean isColumn) {
ICursor cursor = null;
if (fkNames != null) {
if (mcs != null ) {
if (mcs instanceof MultipathCursors) {
cursor = table.cursor(null, this.names, filter, fkNames, codes, null, (MultipathCursors)mcs, null, ctx);
} else {
if (exps == null) {
cursor = table.cursor(null, this.names, filter, fkNames, codes, null, null, ctx);
} else {
cursor = table.cursor(this.exps, this.names, filter, fkNames, codes, null, null, ctx);
}
}
} else if (pathCount > 1) {
if (exps == null) {
cursor = table.cursor(null, this.names, filter, fkNames, codes, null, pathCount, null, ctx);
} else {
cursor = table.cursor(this.exps, this.names, filter, fkNames, codes, null, pathCount, null, ctx);
}
} else {
if (exps == null) {
cursor = table.cursor(null, this.names, filter, fkNames, codes, null, null, ctx);
} else {
cursor = table.cursor(this.exps, this.names, filter, fkNames, codes, null, null, ctx);
}
}
} else {
if (mcs != null ) {
if (mcs instanceof MultipathCursors) {
cursor = table.cursor(null, this.names, filter, null, null, null, (MultipathCursors)mcs, null, ctx);
} else {
if (exps == null) {
cursor = table.cursor(this.names, filter, ctx);
} else {
cursor = table.cursor(this.exps, this.names, filter, null, null, null, null, ctx);
}
}
} else if (pathCount > 1) {
if (exps == null) {
cursor = table.cursor(null, this.names, filter, null, null, null, pathCount, null, ctx);
} else {
cursor = table.cursor(this.exps, this.names, filter, null, null, null, pathCount, null, ctx);
}
} else {
if (exps == null) {
cursor = table.cursor(this.names, filter, ctx);
} else {
cursor = table.cursor(this.exps, this.names, filter, null, null, null, null, ctx);
}
}
}
addJoin(cursor);
if (addOpt) {
if (opList != null) {
for (Operation op : opList) {
cursor.addOperation(op, ctx);
}
}
if (extraOpList != null) {
for (Operation op : extraOpList) {
cursor.addOperation(op, ctx);
}
}
}
return cursor;
}
/**
* ??user???? ?????ص????α?ʱʹ?ã?
* @param cursors
* @param pd
* @param ctx
* @return
*/
static ICursor sortCursor(ICursor cursor, PseudoDefination pd, Context ctx) {
// if (pd.getDate() == null) {
// return cursor;
// }
String ugrp = pd.getUgrp();
String user = pd.getUser();
DataStruct ds = cursor.getDataStruct();
if (user == null || user.equals(ugrp) || ds.getFieldIndex(user) == -1) {
return cursor;
}
return new GroupSortCursor(cursor, ugrp, user, ctx);
}
/**
* ?鲢?????????α?
* @param cursors
* @return
*/
static ICursor mergeCursor(ICursor cursors[], PseudoDefination pd, Context ctx) {
if (pd.getDate() == null) {
return new ConjxCursor(cursors);
}
String ugrp = pd.getUgrp();
DataStruct ds = cursors[0].getDataStruct();
int index = ds.getFieldIndex(ugrp);
if (index == -1) {
return new ConjxCursor(cursors);
}
ICursor cs = new MergeCursor(cursors, new int[] {index}, null, ctx);
//???user?????ڻ????ugrp
String user = pd.getUser();
if (user == null || user.equals(ugrp) || ds.getFieldIndex(user) == -1) {
return cs;
}
//user???????ֶΣ??ڹ鲢??ֵͬ???ֶ??ڵ????ݰ?user????
return new GroupSortCursor(cs, ugrp, user, ctx);
}
static ICursor mergeCursor(ICursor cursors[], Context ctx) {
return new ConjxCursor(cursors);
}
/**
* ???????user???˻????ԣ???group(???ֶ?).conj(~.group(user)),
* ?????????user????ʹ?????ֶι鲢??
*/
static void groupByUser(ICursor cursor, String user, String ugrp, Context ctx) {
if (user != null && ugrp != null && user.equals(ugrp)) {
Expression[] exps = new Expression[1];
exps[0] = new Expression(ugrp);
cursor.addOperation(new Group(exps, null), ctx);
cursor.addOperation(new Conj(new Expression("~.group("+user+")")), ctx);
}
}
private ICursor addOptionToCursor(ICursor cursor) {
if (opList != null) {
for (Operation op : opList) {
cursor.addOperation(op, ctx);
}
}
if (extraOpList != null) {
for (Operation op : extraOpList) {
cursor.addOperation(op, ctx);
}
}
return cursor;
}
private List filterTables(List tables) {
if (filter != null && pd.getDate() != null) {
String dateName = pd.getDate();
PseudoColumn dateCol = pd.findColumnByPseudoName(dateName);
if (dateCol != null && dateCol.getExp() != null) {
dateName = dateCol.getName();
}
IPhyTable table = tables.get(0);
//?ж??Ƿ??й???date?Ĺ???
Object obj;
if (table instanceof ColPhyTable)
obj = Cursor.parseFilter((ColPhyTable) table, filter, ctx);
else
obj = RowCursor.parseFilter((RowPhyTable) table, filter.getHome(), ctx);
IFilter dateFilter = null;
if (obj instanceof IFilter) {
if (((IFilter)obj).getColumnName().equals(dateName)) {
dateFilter = (IFilter)obj;
}
} else if (obj instanceof ArrayList) {
@SuppressWarnings("unchecked")
ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy