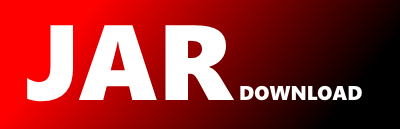
com.scudata.ide.spl.control.ContentPanel Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.ide.spl.control;
import java.awt.BasicStroke;
import java.awt.Color;
import java.awt.Component;
import java.awt.Container;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Graphics2D;
import java.awt.Point;
import java.awt.Rectangle;
import java.awt.Stroke;
import java.awt.event.FocusAdapter;
import java.awt.event.FocusEvent;
import java.awt.event.InputMethodEvent;
import java.awt.event.InputMethodListener;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.awt.font.TextHitInfo;
import java.awt.im.InputMethodRequests;
import java.text.AttributedCharacterIterator;
import java.text.AttributedCharacterIterator.Attribute;
import java.util.List;
import java.util.Vector;
import javax.swing.BorderFactory;
import javax.swing.JComponent;
import javax.swing.JInternalFrame;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JTextPane;
import javax.swing.text.Caret;
import javax.swing.text.DefaultCaret;
import javax.swing.text.JTextComponent;
import com.scudata.cellset.INormalCell;
import com.scudata.cellset.IStyle;
import com.scudata.cellset.datamodel.CellSet;
import com.scudata.cellset.datamodel.NormalCell;
import com.scudata.cellset.datamodel.PgmNormalCell;
import com.scudata.common.Area;
import com.scudata.common.CellLocation;
import com.scudata.common.IntArrayList;
import com.scudata.common.StringUtils;
import com.scudata.ide.common.ConfigOptions;
import com.scudata.ide.common.GC;
import com.scudata.ide.common.GM;
import com.scudata.ide.common.GV;
import com.scudata.ide.common.control.BorderStyle;
import com.scudata.ide.common.control.CellBorder;
import com.scudata.ide.common.control.CellRect;
import com.scudata.ide.common.swing.JComboBoxEx;
import com.scudata.ide.common.swing.JTextPaneEx;
import com.scudata.ide.spl.GVSpl;
import com.scudata.ide.spl.SheetSpl;
import com.scudata.ide.spl.ToolBarProperty;
/** ??????????? */
public class ContentPanel extends JPanel implements InputMethodListener,
InputMethodRequests {
private static final long serialVersionUID = 1L;
/**
* ?????ɫ
*/
public static Color XOR_COLOR = new Color(51, 0, 51);
/** ??????? */
CellSet cellSet;
/** ????????? */
protected CellSetParser parser;
/** ?????????Ƶ???ʼ?? */
protected int startRow;
/** ?????????ƵĽ????? */
protected int endRow;
/** ?????????Ƶ???ʼ?? */
protected int startCol;
/** ?????????ƵĽ????? */
protected int endCol;
/**
* ?Ƿ??ڱ༭״̬?????ֵ?Ԫ??ֵ??ʾ????ʽ??????ʾ??ֵ?õġ? ????Ӧ????û?õ??????ˣ?????????true??Ҳ????????ʾ?ĵ?Ԫ?????ʽ
**/
protected boolean isEditing;
/** ?Ƿ?ֻ????????ʾ???ڴ?С?ڵ????? */
protected boolean onlyDrawCellInWin;
/** ?Ƿ?ɱ༭ */
protected boolean editable;
/** ???????????Ĺ??????? */
JScrollPane jsp;
/** ????ؼ? */
protected SplControl control;
/** ??Ԫ???X???????? */
int[][] cellX;
/** ??Ԫ???Y???????? */
int[][] cellY;
/** ??Ԫ??Ŀ??????? */
int[][] cellW;
/** ??Ԫ??ĸ߶????? */
int[][] cellH;
/** ??ͼ??ʼ???С??У???ͼ???????С??? */
public int drawStartRow, drawStartCol, drawEndRow, drawEndCol;
/** ???б༭?? */
protected JTextPaneEx multiEditor;
/** ??ǰ?༭?ؼ? */
protected JComponent editor;
/** ?÷?????ı䵱ǰ??Ԫ??ʱ????¼?ĵ?ǰ????к? */
public int rememberedRow = 0;
/** ?÷?????ı䵱ǰ??Ԫ??ʱ????¼?ĵ?ǰ????к? */
public int rememberedCol = 0;
/** ???б༭?????? */
public static final int MULTI_EDITOR = 2;
/**
* ???б༭???Ĺ??????
*/
protected JScrollPane spEditor;
/**
* ?Ƿ???ֹ?仯
*/
protected boolean preventChange = false;
/**
* ?߿???ʽ
*/
protected BorderStyle borderStyle = new BorderStyle();
/** ͼ????????Ⱦ???????߷??4f */
public static BasicStroke bs1 = new BasicStroke(2.0f, BasicStroke.CAP_BUTT,
BasicStroke.JOIN_MITER, 1f, new float[] { 4f }, 0f);
/** ͼ????????Ⱦ???????߷??5f */
public static BasicStroke bs2 = new BasicStroke(2.0f, BasicStroke.CAP_BUTT,
BasicStroke.JOIN_MITER, 1f, new float[] { 5f }, 0f);
/**
* ͼ????????Ⱦ??
*/
protected BasicStroke bs = null;
/**
* ???ڱ༭?ĵ?Ԫ??????
*/
protected CellLocation editPos = null;
/**
* ???ڻ?ԭ?༭?ı?
*/
protected String undoExp = null;
protected SheetSpl sheet;
/**
* ???캯??
*
* @param cellSet
* ???????
* @param startRow
* ?????????ʼ??
* @param endRow
* ????????????
* @param startCol
* ?????????ʼ??
* @param endCol
* ????????????
* @param isEditing
* ????Ƿ?λ?ڱ༭?ؼ???
* @param onlyDrawCellInWin
* ?Ƿ?ֻ????ʾ???ڴ?С?ڵ????
* @param jsp
* ???????Ĺ???????
*/
public ContentPanel(CellSet cellSet, int startRow, int endRow,
int startCol, int endCol, boolean isEditing,
boolean onlyDrawCellInWin, JScrollPane jsp) {
this(cellSet, startRow, endRow, startCol, endCol, isEditing,
onlyDrawCellInWin, jsp, null);
}
/**
* ???캯??
*
* @param cellSet
* ???????
* @param startRow
* ?????????ʼ??
* @param endRow
* ????????????
* @param startCol
* ?????????ʼ??
* @param endCol
* ????????????
* @param isEditing
* ????Ƿ?λ?ڱ༭?ؼ???
* @param onlyDrawCellInWin
* ?Ƿ?ֻ????ʾ???ڴ?С?ڵ????
* @param jsp
* ???????Ĺ???????
* @param sheet
* ҳ?????
*/
public ContentPanel(CellSet cellSet, int startRow, int endRow,
int startCol, int endCol, boolean isEditing,
boolean onlyDrawCellInWin, JScrollPane jsp, SheetSpl sheet) {
this.sheet = sheet;
this.cellSet = cellSet;
this.parser = newCellSetParser(cellSet);
this.startRow = startRow;
this.endRow = endRow;
this.startCol = startCol;
this.endCol = endCol;
this.isEditing = isEditing;
this.onlyDrawCellInWin = onlyDrawCellInWin;
this.jsp = jsp;
if (jsp instanceof SplControl) {
control = (SplControl) jsp;
}
setDoubleBuffered(true);
initCellLocations();
setLayout(null);
newEditor();
spEditor = new JScrollPane(multiEditor);
spEditor.setHorizontalScrollBarPolicy(JScrollPane.HORIZONTAL_SCROLLBAR_NEVER);
spEditor.setVerticalScrollBarPolicy(JScrollPane.VERTICAL_SCROLLBAR_NEVER);
multiEditor.setVisible(false);
spEditor.setVisible(false);
add(spEditor);
if (!isEditing) {
addMouseListener(new ShowEditorListener(this));
} else {
enableInputMethods(true);
addInputMethodListener(this);
addEditorFocusListener(multiEditor);
addCellEditingListener(control, multiEditor);
EditorRightClicked erc = new EditorRightClicked(control);
multiEditor.addMouseListener(erc);
MouseAdapter ma = new MouseAdapter() {
public void mouseClicked(MouseEvent e) {
if (e.getClickCount() == 2) {
MouseListener[] ml = getMouseListeners();
if (ml != null) {
for (int i = 0; i < ml.length; i++) {
ml[i].mouseClicked(e);
}
}
}
}
};
multiEditor.addMouseListener(ma);
}
}
/**
* ?????༭?ؼ?
*/
protected void newEditor() {
multiEditor = new JTextPaneEx() {
private static final long serialVersionUID = 1L;
public Point getToolTipLocation(MouseEvent e) {
if (control.getActiveCell() == null) {
return new Point(-9999, -9999);
}
int r = control.getActiveCell().getRow();
int c = control.getActiveCell().getCol();
Point p = getTipPos(1 + cellX[r][c], 1 + cellY[r][c]);
return new Point(p.x - cellX[r][c], p.y - cellY[r][c]);
}
protected void docUpdate() {
if (preventChange || super.preventChanged)
return;
super.docUpdate();
try {
GV.toolBarProperty.setTextEditorText(multiEditor.getText());
} catch (Throwable t) {
}
try {
resetEditorBounds();
} catch (Throwable e) {
e.printStackTrace();
}
}
};
}
/**
* ?????????????
*
* @param cellSet
* ????
* @return CellSetParser
*/
protected CellSetParser newCellSetParser(CellSet cellSet) {
return new CellSetParser(cellSet);
}
/**
* ????????༭??????
*
* @return
*/
protected void addCellEditingListener(SplControl control,
JTextComponent jtext) {
CellEditingListener listener = new CellEditingListener(control, this);
jtext.addKeyListener(listener);
}
/**
* ???ӱ༭?ؼ??????¼?
*
* @param jtext
*/
protected void addEditorFocusListener(JTextComponent jtext) {
jtext.addFocusListener(new FocusAdapter() {
public void focusGained(FocusEvent e) {
GVSpl.isCellEditing = true;
}
});
}
/**
* ?????Ƿ???Ա༭
*
* @param editable
*/
public void setEditable(boolean editable) {
this.editable = editable;
repaint();
}
/**
* ȡ?Ƿ???Ա༭
*
* @return
*/
public boolean isEditable() {
return editable;
}
protected boolean isCellEditable(int row, int col) {
return editable;
}
/**
* ???????????
*
* @param newCellSet
* ???????
*/
public void setCellSet(CellSet newCellSet) {
this.cellSet = newCellSet;
this.parser = new CellSetParser(newCellSet);
repaint();
}
/**
* ȡ????ؼ?
*
* @return
*/
public SplControl getControl() {
return control;
}
/**
* ??????ijߴ?
*
* @return ???ijߴ?
*/
public Dimension getPreferredSize() {
float scale = 1.0f;
if (control != null) {
scale = control.scale;
}
/* Undoʱû?????¹?????????endCol??ʵ???п??ܲ??? */
if (endCol > cellSet.getColCount()) {
endCol = cellSet.getColCount();
}
if (endRow > cellSet.getRowCount()) {
endRow = cellSet.getRowCount();
}
/* ????End */
int width = 0;
for (int col = startCol; col <= cellSet.getColCount(); col++) {
if (!parser.isColVisible(col)) {
continue;
}
width += parser.getColWidth(col, scale);
}
int height = 0;
for (int row = startRow; row <= cellSet.getRowCount(); row++) {
if (!parser.isRowVisible(row)) {
continue;
}
height += parser.getRowHeight(row, scale);
}
return new Dimension(width + 2, height + 2);
}
/**
* ??ȡ?к?????ڶ??ߵ????ظ߶ȣ????ڹ???????λ
*
* @param row
* int
* @return int
*/
public int getRowOffset(int row, float scale) {
int h = 0;
for (int r = 1; r < row; r++) {
if (!parser.isRowVisible(r)) {
continue;
}
h += parser.getRowHeight(r, scale);
}
return h;
}
/**
* ??ȡ?к????????ߵ????ظ߶ȣ????ڹ???????λ
*
* @param col
* @return
*/
public int getColOffset(int col, float scale) {
int w = 0;
for (int c = 1; c < col; c++) {
if (!parser.isColVisible(c)) {
continue;
}
w += parser.getColWidth(c, scale);
}
return w;
}
/**
* ???????
*
* @param g
* ????
*/
public void paintComponent(Graphics g) {
super.paintComponent(g);
ControlUtils.setGraphicsRenderingHints(g);
float scale = 1.0f;
if (control != null) {
scale = control.scale;
}
int rows = cellSet.getRowCount() + 1;
int cols = cellSet.getColCount() + 1;
if (cols != cellX[0].length || rows != cellX.length) {
initCellLocations(rows, cols);
}
clearCoordinate();
boolean isSub = false;
if (isEditing) {
if (!isSub) {
g.clearRect(0, 0, 999999, 999999);
}
startRow = startCol = 1;
endRow = rows - 1;
endCol = cols - 1;
}
Rectangle displayWin = null;
if (onlyDrawCellInWin) {
displayWin = jsp.getViewport().getViewRect();
}
if (!isEditing && !isSub) {
g.setColor(Color.white);
if (onlyDrawCellInWin) {
g.fillRect(displayWin.x, displayWin.y, displayWin.width + 5,
displayWin.height + 5);
} else {
Dimension d = getPreferredSize();
g.fillRect(0, 0, d.width + 5, d.height + 5);
}
}
int x = 1, y = 1;
drawStartRow = startRow;
if (displayWin != null && displayWin.y >= 0) {
int i;
for (i = startRow; i < endRow; i++) {
if (!parser.isRowVisible(i)) {
continue;
}
int rh = parser.getRowHeight(i, scale);
if (y + rh > displayWin.y) {
break;
}
y += rh;
}
drawStartRow = i;
}
drawStartCol = startCol;
if (displayWin != null && displayWin.y >= 0) {
int i;
for (i = startCol; i < endCol; i++) {
if (!parser.isColVisible(i)) {
continue;
}
int rw = parser.getColWidth(i, scale);
if (x + rw > displayWin.x) {
break;
}
x += rw;
}
drawStartCol = i;
}
// ?????»???ʹ?õ?
float underLineSize = 0.75f;
underLineSize *= control.scale;
((Graphics2D) g).setStroke(new BasicStroke(underLineSize));
drawEndRow = endRow;
drawEndCol = endCol;
int tmpX = x;
for (int row = drawStartRow; row <= endRow; row++) {
if (displayWin != null && y >= displayWin.y + displayWin.height) {
drawEndRow = row - 1;
break;
}
if (!parser.isRowVisible(row)) {
continue;
}
x = tmpX;
for (int col = drawStartCol; col <= endCol; col++) {
if (displayWin != null && x >= displayWin.x + displayWin.width) {
drawEndCol = col - 1;
break;
}
if (!parser.isColVisible(col)) {
continue;
}
int colWidth = parser.getColWidth(col, scale);
int width = colWidth;
int height = parser.getRowHeight(row, scale);
if (displayWin != null && x + width <= displayWin.x) {
x += colWidth;
continue;
}
if (displayWin != null && y + height <= displayWin.y) {
x += colWidth;
continue;
}
cellX[row][col] = x;
cellW[row][col] = width;
cellY[row][col] = y;
cellH[row][col] = height;
// fill back color
Color bkcolor = parser.getBackColor(row, col);
if (bkcolor != null) {
g.setColor(bkcolor);
g.fillRect(x, y, width, height);
}
if (!ConfigOptions.bDispOutCell.booleanValue()) {
drawText(g, row, col, x, y, width, height, scale);
}
drawFlag(g, x, y, parser, row, col, scale);
// draw border
CellBorder.setEnv(g, borderStyle, row, col,
parser.getRowCount(), parser.getColCount(), isEditing);
CellBorder.drawBorder(x, y, width, height);
// draw selectedCell
if (isCellSelected(row, col)) {
/**
* ???ĵ?Ԫ??ɫ
*/
Color selectCellBkcolor;
if (ConfigOptions.getCellColor() != null) {
selectCellBkcolor = ConfigOptions.getCellColor();
} else {
selectCellBkcolor = Color.black;
g.setXORMode(XOR_COLOR);
}
g.setColor(selectCellBkcolor);
g.fillRect(cellX[row][col], cellY[row][col],
cellW[row][col], cellH[row][col]);
g.setPaintMode();
}
// draw refcell
if (editor != null && editor.isVisible()) {
if (editor == multiEditor) {
List refCells = multiEditor.getRefCells();
if (refCells != null && !refCells.isEmpty()) {
Color refCellColor = multiEditor.getRefCellColor(
row, col);
if (refCellColor != null) {
g.setColor(refCellColor);
Stroke oldStroke = ((Graphics2D) g).getStroke();
float lineSize = 2.0f;
lineSize *= control.scale;
((Graphics2D) g).setStroke(new BasicStroke(
lineSize));
g.drawRect(cellX[row][col], cellY[row][col],
cellW[row][col], cellH[row][col]);
// g.drawRect(cellX[row][col] + 1,
// cellY[row][col] + 1,
// cellW[row][col] - 2,
// cellH[row][col] - 2);
((Graphics2D) g).setStroke(oldStroke);
g.setPaintMode();
}
}
}
}
if (control.isBreakPointCell(row, col)) {
g.setColor(Color.magenta);
int r = row;
int c = col;
g.fillRect(cellX[r][c], cellY[r][c], cellW[r][c],
cellH[r][c]);
g.setPaintMode();
}
if (control.getStepPosition() != null) {
CellLocation cp = control.getStepPosition();
int r = cp.getRow();
int c = cp.getCol();
if (r == row && c == col) {
g.setColor(Color.blue);
g.fillRect(cellX[r][c], cellY[r][c], cellW[r][c],
cellH[r][c]);
g.setPaintMode();
if (!ConfigOptions.bDispOutCell.booleanValue()) {
drawText(g, row, col, x, y, width, height, scale);
}
}
}
x += colWidth;
}
y += parser.getRowHeight(row, scale);
}
if (this.control != null && ConfigOptions.bDispOutCell.booleanValue()) {
// ????????Ԫ????ʾ??????????֣?ʹ???????????ϲ?
for (int row = drawStartRow; row <= drawEndRow; row++) {
for (int col = drawStartCol; col <= drawEndCol; col++) {
if (cellX[row][col] == 0) {
continue;
}
// draw cell content
int height = parser.getRowHeight(row, scale);
int w = parser.getColWidth(col, scale);
int pw = getPaintableWidth(row, col, scale);
int px = cellX[row][col];
int py = cellY[row][col];
int halign = parser.getHAlign(row, col);
if (halign == IStyle.HALIGN_RIGHT) {
px = px + w - pw;
}
drawText(g, row, col, px, py, pw, height, scale);
}
}
}
if (control != null && control.getCopySourceArea() != null) {
if (bs == bs1) {
bs = bs2;
} else {
bs = bs1;
}
Area a = control.getCopySourceArea();
int copyBeginRow = a.getBeginRow();
// ???Ƶ?Area?п??ܳ????滭???
int copyEndRow = a.getEndRow();
int copyBeginCol = a.getBeginCol();
int copyEndCol = a.getEndCol();
x = -1;
y = -1;
if (copyBeginRow < drawStartRow) {
copyBeginRow = drawStartRow;
y = new Double(Math.abs(getY())).intValue();
}
if (copyBeginCol < drawStartCol) {
copyBeginCol = drawStartCol;
x = new Double(Math.abs(getX())).intValue();
}
if (y == -1) {
y = cellY[copyBeginRow][copyBeginCol];
}
if (x == -1) {
x = cellX[copyBeginRow][copyBeginCol];
}
int w = -1, h = -1;
if (copyEndRow > drawEndRow) {
h = new Double(getBounds().getMaxY() - getBounds().getMinY())
.intValue();
}
if (copyEndCol > drawEndCol) {
w = new Double(getBounds().getMaxX() - getBounds().getMinX())
.intValue();
}
if (w == -1) {
w = cellX[copyBeginRow][copyEndCol] - x
+ cellW[copyBeginRow][copyEndCol];
}
if (h == -1) {
h = cellY[copyEndRow][copyBeginCol] - y
+ cellH[copyEndRow][copyBeginCol];
}
Graphics2D g2 = (Graphics2D) g;
g2.setColor(Color.blue);
BasicStroke stroke = (BasicStroke) g2.getStroke();
g2.setStroke(bs);
g2.drawRect(x, y, w, h);
g2.setStroke(stroke);
}
/*
* ????Ϊtrue?????ػ?ʱװ?ؿؼ??ı??ᵼ?¹???û?????? false????װ????????????
* ???ػ?ʱ??װ?ؿؼ??ı?,?ƺ?ij??????????뷨????????ʱ??????
*/
initEditor(submitEditor(true));
drawSelectedRectBorder(g);
if (!isSub) {
g.dispose();
}
}
/**
* ??Ԫ???Ƿ?ѡ??
*
* @param row
* ?к?
* @param col
* ?к?
* @return
*/
protected boolean isCellSelected(int row, int col) {
if (control == null) {
return false;
}
Vector
© 2015 - 2024 Weber Informatics LLC | Privacy Policy