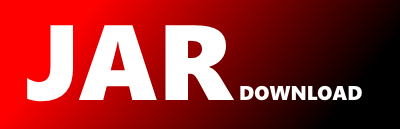
com.scudata.ide.spl.dialog.DialogCopyPresent Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.ide.spl.dialog;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.KeyboardFocusManager;
import java.awt.Rectangle;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Collections;
import java.util.Vector;
import javax.swing.JCheckBox;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.text.BadLocationException;
import javax.swing.text.JTextComponent;
import org.fife.ui.rsyntaxtextarea.RSyntaxTextArea;
import org.fife.ui.rsyntaxtextarea.SyntaxConstants;
import org.fife.ui.rtextarea.RTextScrollPane;
import com.scudata.common.MessageManager;
import com.scudata.ide.common.ConfigOptions;
import com.scudata.ide.common.GC;
import com.scudata.ide.common.GM;
import com.scudata.ide.common.dialog.RQDialog;
import com.scudata.ide.common.swing.JComboBoxEx;
import com.scudata.ide.spl.GVSpl;
import com.scudata.ide.spl.resources.IdeSplMessage;
/**
* ???ƿɳ??ִ???Ի???
*
*/
public class DialogCopyPresent extends RQDialog {
private static final long serialVersionUID = 1L;
/**
* ???캯??
*/
public DialogCopyPresent() {
super("Copy code for presentation", 600, 500);
try {
init();
GM.centerWindow(this);
} catch (Exception e) {
GM.showException(e);
}
}
/**
* ????ѡ??
*
* @param showException ?Ƿ????쳣
* @return
*/
private boolean saveOption(boolean showException) {
byte type = ((Number) jCBType.x_getSelectedItem()).byteValue();
if (type == ConfigOptions.COPY_TEXT) {
String sep = getSep();
if (sep == null || "".equals(sep)) {
if (showException)
// ?붨???зָ?????
GM.messageDialog(this,
mm.getMessage("dialogcopypresent.emptycolsep"));
return false;
}
ConfigOptions.sCopyPresentSep = sep;
}
ConfigOptions.iCopyPresentType = type;
ConfigOptions.bCopyPresentHeader = jCBHeader.isSelected();
return true;
}
/**
* ȷ?ϰ?ť?¼?
*/
protected boolean okAction(ActionEvent e) {
if (!saveOption(true)) {
return false;
}
return true;
}
/**
* ?رնԻ???
*/
protected void closeDialog(int option) {
super.closeDialog(option);
GM.setWindowDimension(this);
ConfigOptions.bTextEditorLineWrap = jCBLineWrap.isSelected();
}
/**
* ȡ?ָ???
*
* @return
*/
private String getSep() {
Object disp = jCBSep.getEditor().getItem();
if (disp != null) {
int index = disps.indexOf(disp);
if (index > -1) {
return (String) codes.get(index);
} else {
return disp.toString();
}
}
return "";
}
/**
* ??ʼ??
*/
private void init() {
panelCenter.setLayout(new GridBagLayout());
panelCenter.add(jLType, GM.getGBC(0, 0));
panelCenter.add(jCBType, GM.getGBC(0, 1));
GridBagConstraints gbc = GM.getGBC(1, 0);
gbc.gridwidth = 2;
panelCenter.add(jCBHeader, gbc);
panelCenter.add(jLSep, GM.getGBC(2, 0));
panelCenter.add(jCBSep, GM.getGBC(2, 1));
panelCenter.add(jLPreview, GM.getGBC(3, 0));
gbc = GM.getGBC(4, 0, true, true);
gbc.gridwidth = 2;
panelCenter.add(jSPPreview, gbc);
gbc = GM.getGBC(5, 0);
gbc.gridwidth = 2;
panelCenter.add(jCBLineWrap, gbc);
Vector
© 2015 - 2024 Weber Informatics LLC | Privacy Policy