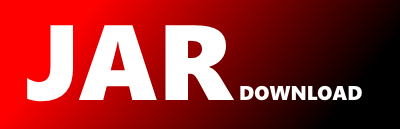
com.scudata.server.odbc.OdbcServer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.server.odbc;
import java.io.InputStream;
import java.io.InterruptedIOException;
import java.net.InetAddress;
import java.net.ServerSocket;
import java.net.Socket;
import com.scudata.app.config.RaqsoftConfig;
import com.scudata.common.Logger;
import com.scudata.common.StringUtils;
import com.scudata.dm.Env;
import com.scudata.ide.common.AppFrame;
import com.scudata.parallel.TempFileMonitor;
import com.scudata.parallel.UnitContext;
import com.scudata.resources.ParallelMessage;
import com.scudata.server.ConnectionProxyManager;
import com.scudata.server.IServer;
import com.scudata.server.StartUnitListener;
/**
* odbc????????ʵ?ֶ?odbc?ӿڵķ???
*
* @author Joancy
*
*/
public class OdbcServer implements IServer {
public static OdbcServer instance = null;
StartUnitListener listener = null;
private OdbcContext ctx = null;
private OdbcMonitor odbcMonitor = null;
TempFileMonitor tempFileMonitor = null;
volatile boolean stop = true;
static int objectId = 0;
static Object idLock = new Object();
ThreadGroup workers = new ThreadGroup("OdbcWorker");
private RaqsoftConfig rc = null;
/**
* ??????Ǭ????
*/
public void setRaqsoftConfig(RaqsoftConfig rc){
this.rc = rc;
}
/**
* ??ȡ??Ǭ?????ļ?????
*/
public RaqsoftConfig getRaqsoftConfig(){
return rc;
}
private OdbcServer() throws Exception {
}
/**
* ͨ????̬??????ȡΨһ??????ʵ??
* @return odbc??????ʵ??
* @throws Exception
*/
public static OdbcServer getInstance() throws Exception {
if (instance == null) {
instance = new OdbcServer();
}
return instance;
}
/**
* ??ֹ????
*/
public void terminate() {
shutDown();
}
/**
* ֹͣ??????
*/
public void shutDown() {
stop = true;
}
/**
* ???ط??????Ƿ???????״̬
*/
public synchronized boolean isRunning() {
return isAlive();
}
private synchronized void setStop(boolean b, int port) {
stop = b;
if (!stop && listener != null) {
listener.serverStarted(port);
}
}
/**
* ?????????Ƿ???
* @return ?????true??????false
*/
public boolean isAlive() {
return !stop;
}
/**
* ??????ID?ż???
* @return Ψһ??
*/
public static int nextId() {
synchronized (idLock) {
int c = ++objectId;
if (c == Integer.MAX_VALUE) {
objectId = 1;
c = 1;
}
return c;
}
}
/**
* ??ȡodbc??????
* @return odbc??????
*/
public OdbcContext getContext() {
return ctx;
}
/**
* ??ȡ??????Home·??
* @return home·??
*/
public static String getHome() {
String home = System.getProperty("start.home");
return home;
}
/**
* ???з?????
*/
public void run() {
ServerSocket ss = null;
Logger.info("Release date:"+AppFrame.RELEASE_DATE);
Logger.info(ParallelMessage.get().getMessage("OdbcServer.run1"));
Logger.info(ParallelMessage.get().getMessage("UnitServer.run2", getHome()));
try {
// ?ȼ?????????ļ??Ƿ????
InputStream is = UnitContext.getUnitInputStream(OdbcContext.ODBC_CONFIG_FILE);
is.close();
ctx = new OdbcContext();
} catch (Exception x) {
if (listener != null) {
listener.serverStartFail();
}
x.printStackTrace();
return;
}
String host = ctx.getHost();
int port = ctx.getPort();
try {
InetAddress add = InetAddress.getByName(host);
ss = new ServerSocket(port, 10, add);
int TimeOut = 3000;
ss.setSoTimeout(TimeOut);
} catch (Exception x) {
if(listener!=null){
listener.serverStartFail();
}
if(x instanceof java.net.BindException){
System.out.println(ParallelMessage.get().getMessage("DfxServerInIDE.portbind",host+":"+port));
}else{
x.printStackTrace();
}
return;
}
if (StringUtils.isValidString(Env.getTempPath())) {
int timeOut = ctx.getTimeOut();
int interval = ctx.getConPeriod();
tempFileMonitor = new TempFileMonitor(timeOut, interval);
tempFileMonitor.start();
}
odbcMonitor = new OdbcMonitor();
odbcMonitor.start();
Logger.info(ParallelMessage.get().getMessage("OdbcServer.run3", host + ":" + port));
setStop(false,port);
int c = 0;
try {
while (!stop) {
try {
Socket s = ss.accept();
OdbcWorker ow = new OdbcWorker(workers, "OdbcWorker-" + c++);
ow.setSocket(s);
ow.start();
} catch (InterruptedIOException e) {
// ??ʱ?????ж??쳣
}
}
Thread[] threads = new Thread[workers.activeCount()];
workers.enumerate(threads);
for (int i = 0; i < threads.length; i++) {
Thread t = threads[i];
if (t.isAlive()) {
((OdbcWorker) t).shutDown();
}
}
if (tempFileMonitor != null) {
tempFileMonitor.stopThread();
}
odbcMonitor.stopThread();
ConnectionProxyManager.getInstance().destroy();
Logger.info(ParallelMessage.get().getMessage("OdbcServer.stop"));
} catch (Exception x) {
x.printStackTrace();
Logger.info(ParallelMessage.get().getMessage("OdbcServer.error", x.getMessage()));
} finally {
try {
if (ss != null) {
ss.close();
}
} catch (Exception x) {
}
instance = null;
}
}
/**
* ???÷???????????
*/
public void setStartUnitListener(StartUnitListener listen) {
listener = listen;
}
/**
* ??ȡ??????IP???˿?
*/
public String getHost() {
return ctx.toString();
}
public boolean isAutoStart() {
if(ctx==null){
ctx = new OdbcContext();
}
return ctx.isAutoStart();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy