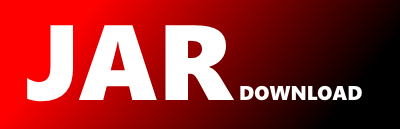
com.scudata.vdb.Section Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of esproc Show documentation
Show all versions of esproc Show documentation
SPL(Structured Process Language) A programming language specially for structured data computing.
package com.scudata.vdb;
import java.io.IOException;
import java.util.ArrayList;
import com.scudata.common.MessageManager;
import com.scudata.common.RQException;
import com.scudata.dm.Context;
import com.scudata.dm.DataStruct;
import com.scudata.dm.FileObject;
import com.scudata.dm.Record;
import com.scudata.dm.Sequence;
import com.scudata.dm.Table;
import com.scudata.expression.Expression;
import com.scudata.resources.EngineMessage;
/**
* ???ݿ???Ľ?
* @author WangXiaoJun
*
*/
class Section extends ISection {
private int header; // ???
private volatile HeaderBlock headerBlock; // ?????ݣ?ʵ???õ?ʱ????????
private Dir dir; // ?ڶ?Ӧ??Ŀ¼??Ϣ
private volatile VDB lockVDB; // ??????ǰ?ڵ?????
private boolean isModified; // ?Ƿ?????
// ?????½?
public Section(Dir dir) {
headerBlock = new HeaderBlock();
this.dir = dir;
}
public Section(Dir dir, int header, byte []bytes) throws IOException {
this.dir = dir;
this.header = header;
headerBlock = new HeaderBlock();
headerBlock.read(bytes, this);
}
// ?????ݿ??ȡ??
/*public Section(Library library, int header, Dir dir) {
this.header = header;
this.dir = dir;
headerBlock = readHeaderBlock(library, header);
}
// ????
private HeaderBlock readHeaderBlock(Library library, int block) {
try {
HeaderBlock headerBlock = new HeaderBlock();
byte []bytes = library.readBlocks(block);
headerBlock.read(bytes, this);
return headerBlock;
} catch (IOException e) {
throw new RQException(e.getMessage(), e);
}
}*/
public void setHeader(int header) {
this.header = header;
}
/**
* ȡ?ڶ?Ӧ??·??????
* @return
*/
public IDir getDir() {
return dir;
}
/**
* ???ش˽??Ƿ??б???
* @return
*/
public boolean isFile() {
return headerBlock.isFile();
}
/**
* ???ش˽??Ƿ???·???????Ƿ?????
* @return
*/
public boolean isDir() {
return headerBlock.isDir();
}
private boolean isNullSection() {
return headerBlock.isNullSection();
}
private boolean isLockVDB(VDB vdb) {
return lockVDB == vdb;
}
/**
* ȡ?ڵĸ???
* @return Section
*/
public Section getParentSection() {
if (dir == null) {
return null;
} else {
return dir.getParentSection();
}
}
/**
* ȡ?ӽ?
* @param vdb ???ݿ????
* @param path ??·??ֵ
* @return ?ӽ?
*/
public ISection getSub(VDB vdb, Object path) {
if (headerBlock.isKeySection()) {
Dir dir = headerBlock.getSubKeyDir(path);
ISection section = dir.getLastZone().getSection(vdb.getLibrary(), dir);
return section.getSub(vdb, path);
}
Dir subDir = headerBlock.getSubDir(path);
if (subDir == null) {
return null;
}
DirZone zone = subDir.getZone(vdb, isLockVDB(vdb));
if (zone == null) {
return null;
}
return zone.getSection(vdb.getLibrary(), subDir);
}
// ???????һ??Section
private Section getSubForWrite(VDB vdb, Sequence paths, Sequence names) {
int pcount = paths.length();
int diff = pcount;
// names?ij??ȿ??Ա?paths?Σ??Ӻ???ǰ??Ӧ
if (names != null) {
diff -= names.length();
}
Section sub = this;
for (int i = 1; i <= pcount; ++i) {
int index = i - diff;
String name = index > 0 ? (String)names.getMem(index) : null;
sub = sub.getSubForWrite(vdb, paths.getMem(i), name);
if (sub == null) {
return null;
}
}
return sub;
}
// д??ʱ??????λ?????????µģ????????û??ϵ
// ??????????????·???͵ȴ?
// ???·??????????????ס?????û????·??isLockָʾ?Ƿ????
private Section getSubForWrite(VDB vdb, Object path, String name) {
if (headerBlock.isKeySection()) {
Dir dir = headerBlock.getSubKeyDir(path);
Section section = dir.getLastZone().getSectionForWrite(vdb.getLibrary(), dir);
return section.getSubForWrite(vdb, path, name);
}
int result = lock(vdb);
if (result == VDB.S_LOCKTIMEOUT) {
return null;
}
try {
Library library = vdb.getLibrary();
Dir subDir = headerBlock.getSubDir(path);
if (subDir == null) {
if (result != VDB.S_LOCKTWICE) {
vdb.addModifySection(this);
}
isModified = true;
subDir = headerBlock.createSubDir(path, name, this);
DirZone zone = subDir.getLastZone();
return zone.getSectionForWrite(library, subDir);
}
DirZone zone = subDir.getLastZone();
if (zone == null || !zone.valid()) {
if (result != VDB.S_LOCKTWICE) {
vdb.addModifySection(this);
}
isModified = true;
zone = subDir.addZone(Dir.S_NORMAL);
return zone.getSectionForWrite(library, subDir);
} else {
if (result != VDB.S_LOCKTWICE) {
unlock();
}
return zone.getSectionForWrite(library, subDir);
}
} catch (Exception e) {
unlock();
return null;
}
}
/**
* ȡ?ӽ????????ƶ?????
* @param vdb ???ݿ????
* @param path ??·??ֵ
* @return ?ӽ?
*/
public Section getSubForMove(VDB vdb, Object path) {
if (headerBlock.isKeySection()) {
Dir dir = headerBlock.getSubKeyDir(path);
DirZone zone = dir.getLastZone();
Section section = zone.getSectionForWrite(vdb.getLibrary(), dir);
return section.getSubForMove(vdb, path);
}
Dir subDir = headerBlock.getSubDir(path);
if (subDir == null) {
return null;
}
DirZone zone = subDir.getLastZone();
if (zone == null || !zone.valid()) {
return null;
} else {
return zone.getSectionForWrite(vdb.getLibrary(), subDir);
}
}
/**
* ȡ?ӽ????????ƶ?????
* @param vdb ???ݿ????
* @param paths ??·??ֵ????
* @return ?ӽ?
*/
public Section getSubForMove(VDB vdb, Sequence paths) {
Section sub = this;
for (int i = 1, pcount = paths.length(); i <= pcount; ++i) {
sub = sub.getSubForMove(vdb, paths.getMem(i));
if (sub == null) {
return null;
}
}
return sub;
}
/**
* ??ס?????ܲ???Ȼ?????
* @param vdb
* @return
*/
public synchronized int lock(VDB vdb) {
if (lockVDB == null) {
lockVDB = vdb;
return VDB.S_SUCCESS;
} else if (lockVDB != vdb) {
try {
wait(Library.MAXWAITTIME);
if (lockVDB != null) { // ??ʱ
vdb.setError(VDB.S_LOCKTIMEOUT);
return VDB.S_LOCKTIMEOUT;
} else {
lockVDB = vdb;
return VDB.S_SUCCESS;
}
} catch (InterruptedException e) {
vdb.setError(VDB.S_LOCKTIMEOUT);
return VDB.S_LOCKTIMEOUT;
}
} else {
return VDB.S_LOCKTWICE;
}
}
/**
* ??ס????
*/
public synchronized int lockForWrite(VDB vdb) {
if (lockVDB == null) {
lockVDB = vdb;
vdb.addModifySection(this);
return VDB.S_SUCCESS;
} else if (lockVDB != vdb) {
try {
wait(Library.MAXWAITTIME);
if (lockVDB != null) { // ??ʱ
vdb.setError(VDB.S_LOCKTIMEOUT);
return VDB.S_LOCKTIMEOUT;
} else {
lockVDB = vdb;
vdb.addModifySection(this);
return VDB.S_SUCCESS;
}
} catch (InterruptedException e) {
vdb.setError(VDB.S_LOCKTIMEOUT);
return VDB.S_LOCKTIMEOUT;
}
} else {
return VDB.S_SUCCESS;
}
}
/**
* ??????ǰ??
*/
public synchronized void unlock() {
isModified = false;
lockVDB = null;
notify();
}
/**
* ??????ǰ??
* @param vdb ???ݿ????
*/
public synchronized void unlock(VDB vdb) {
if (lockVDB == vdb) {
if (isModified) {
headerBlock.roolBack(vdb.getLibrary());
}
isModified = false;
lockVDB = null;
notify();
}
}
/**
* ?ع???ǰ????????????
* @param library ???ݿ????
*/
public void rollBack(Library library) {
if (isModified) {
headerBlock.roolBack(library);
}
unlock();
}
/**
* ?г???ǰ???????е????ļ???
* @param vdb ???ݿ????
* @param opt d???г???Ŀ¼?ڣ?w?????????ļ???Ŀ¼ȫ???г???l????????ǰ??
* @return ?ӽ?????
*/
public Sequence list(VDB vdb, String opt) {
Dir []dirs = headerBlock.getSubDirs();
if (dirs == null) return null;
int size = dirs.length;
Sequence seq = new Sequence(size);
boolean isLockVDB = isLockVDB(vdb);
Library library = vdb.getLibrary();
boolean listFiles = true, listDirs = false;
if (opt != null) {
if (opt.indexOf('w') != -1) {
listFiles = false;
} else if (opt.indexOf('d') != -1) {
listDirs = true;
listFiles = false;
}
if (opt.indexOf('l') != -1) {
lockForWrite(vdb);
}
}
for (Dir dir : dirs) {
DirZone zone = dir.getZone(vdb, isLockVDB);
if (zone != null) {
ISection section = zone.getSection(library, dir);
if (listFiles) {
if (section.isFile()) {
seq.add(new VS(vdb, section));
}
} else if (listDirs) {
if (section.isDir()) {
seq.add(new VS(vdb, section));
}
} else {
seq.add(new VS(vdb, section));
}
}
}
return seq;
}
/**
* ??ȡ??ǰ?ڵı???
* @param vdb ???ݿ????
* @param opt l????????ǰ??
* @return ????????
* @throws IOException
*/
public Object load(VDB vdb, String opt) throws IOException {
if (opt != null && opt.indexOf('l') != -1) {
int result = lockForWrite(vdb);
if (result == VDB.S_LOCKTIMEOUT) {
return null;
}
}
Zone zone = headerBlock.getFileZone(vdb, isLockVDB(vdb));
if (zone != null) {
return zone.getData(vdb.getLibrary());
} else {
return null;
}
}
/**
* ????ֵ????ǰ??????
* @param vdb ???ݿ????
* @param value ֵ??ͨ????????
* @return
*/
public int save(VDB vdb, Object value) {
int result = lockForWrite(vdb);
if (result == VDB.S_LOCKTIMEOUT) {
return VDB.S_LOCKTIMEOUT;
}
isModified = true;
Library library = vdb.getLibrary();
int block = library.writeDataBlock(header, value);
headerBlock.createFileZone(library, block);
return VDB.S_SUCCESS;
}
/**
* ????ֵ???ӱ?????
* @param vdb ???ݿ????
* @param value ֵ??ͨ????????
* @param path ?ӽ?ֵ???ӽ?ֵ????
* @param name ?ӽ??????ӽ???????
* @return 0???ɹ?
*/
public int save(VDB vdb, Object value, Object path, Object name) {
Section sub;
if (path instanceof Sequence) {
Sequence paths = (Sequence)path;
Sequence names = null;
if (name instanceof Sequence) {
names = (Sequence)name;
for (int i = 1, len = names.length(); i <= len; ++i) {
Object obj = names.getMem(i);
if (!(obj instanceof String)) {
vdb.setError(VDB.S_PARAMTYPEERROR);
return VDB.S_PARAMTYPEERROR;
}
}
} else if (name instanceof String) {
names = new Sequence(1);
names.add(names);
} else if (name != null) {
vdb.setError(VDB.S_PARAMTYPEERROR);
return VDB.S_PARAMTYPEERROR;
}
sub = getSubForWrite(vdb, paths, names);
} else {
if (name != null && !(name instanceof String)) {
vdb.setError(VDB.S_PARAMTYPEERROR);
return VDB.S_PARAMTYPEERROR;
}
sub = getSubForWrite(vdb, path, (String)name);
}
if (sub != null) {
return sub.save(vdb, value);
} else {
return VDB.S_LOCKTIMEOUT;
}
}
/**
* ??????·??
* @param vdb ???ݿ????
* @param path ?ӽ?ֵ???ӽ?ֵ????
* @param name ?ӽ??????ӽ???????
* @return 0???ɹ?
*/
public int makeDir(VDB vdb, Object path, Object name) {
Section sub;
if (path instanceof Sequence) {
Sequence paths = (Sequence)path;
Sequence names = null;
if (name instanceof Sequence) {
names = (Sequence)name;
for (int i = 1, len = names.length(); i <= len; ++i) {
Object obj = names.getMem(i);
if (!(obj instanceof String)) {
vdb.setError(VDB.S_PARAMTYPEERROR);
return VDB.S_PARAMTYPEERROR;
}
}
} else if (name instanceof String) {
names = new Sequence(1);
names.add(name);
} else if (name != null) {
vdb.setError(VDB.S_PARAMTYPEERROR);
return VDB.S_PARAMTYPEERROR;
}
sub = getSubForWrite(vdb, paths, names);
} else {
if (name != null && !(name instanceof String)) {
vdb.setError(VDB.S_PARAMTYPEERROR);
return VDB.S_PARAMTYPEERROR;
}
sub = getSubForWrite(vdb, path, (String)name);
}
if (sub != null) {
return VDB.S_SUCCESS;
} else {
return VDB.S_LOCKTIMEOUT;
}
}
/**
* ?????????
* @param vdb ???ݿ????
* @param key ????????
* @param len ??ϣ????
* @return 0???ɹ?
*/
public int createSubKeyDir(VDB vdb, Object key, int len) {
// ??ס???ύ??
int result = lockForWrite(vdb);
if (result == VDB.S_LOCKTIMEOUT) {
return result;
}
Library library = vdb.getLibrary();
isModified = true;
Dir subDir = headerBlock.createSubDir(key, null, this);
DirZone zone = subDir.getLastZone();
Section section = zone.getSectionForWrite(library, subDir);
section.setKeySection(vdb, len);
return VDB.S_SUCCESS;
}
/**
* ???渽????ͨ????ͼƬ
* @param vdb ???ݿ????
* @param oldValues ??һ?ε??ôκ????ķ???ֵ
* @param newValues ?ĺ??ֵ
* @param name ????
* @return ֵ???У???????һ?ε??ô˺???
*/
public Sequence saveBlob(VDB vdb, Sequence oldValues, Sequence newValues, String name) {
ArrayList
© 2015 - 2024 Weber Informatics LLC | Privacy Policy