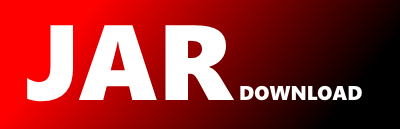
com.sdcalmes.sleeper.StatImpl Maven / Gradle / Ivy
package com.sdcalmes.sleeper;
import retrofit2.Response;
import retrofit2.Retrofit;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
/**
* The type Stat.
*/
class StatImpl
{
private final transient Stats statsEndpoint;
/**
* Instantiates a new Stat.
*
* @param retrofit the retrofit
*/
StatImpl (final Retrofit retrofit)
{
statsEndpoint = retrofit.create(Stats.class);
}
/**
* Gets weekly stats.
*
* @param seasonType the season type
* @param season the season
* @param week the week
* @return the weekly stats
* @throws SleeperError the sleeper error
* @throws IOException the io exception
*/
public Map getWeeklyStats(String seasonType, String season, String week) throws SleeperError, IOException
{
Map statMap;
Response
© 2015 - 2025 Weber Informatics LLC | Privacy Policy