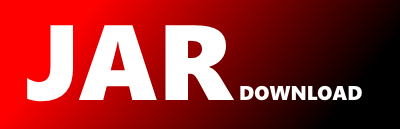
com.sdklite.sphere.hybrid.JSONPResponse Maven / Gradle / Ivy
package com.sdklite.sphere.hybrid;
import java.io.ByteArrayInputStream;
import java.util.Collection;
import java.util.Map;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
/**
* The {@link JSONPResponse} represent a JSON-P javascript resource
*
* @author johnsonlee
* @since 1.0.0
*/
public final class JSONPResponse extends JavaScriptResponse {
/**
* Create a JSON-P response with the specified callback and arguments
*
* @param callback
* The name of javascript callback
* @param args
* The arguments of the callback
*/
public JSONPResponse(final String callback, final Object... args) {
super(new ByteArrayInputStream((callback + "(" + wrapArguments(args) + ");").getBytes()));
}
private static String wrapArguments(final Object[] arguments) {
if (null == arguments || arguments.length <= 0) {
return "";
}
final StringBuilder args = new StringBuilder();
for (int i = 0; i < arguments.length; i++) {
if (i > 0) {
args.append(", ");
}
args.append(wrapArgument(arguments[i]));
}
return args.toString();
}
private static String wrapArgument(final Object o) {
if (o == null || o.equals(JSONObject.NULL)) {
return JSONObject.NULL.toString();
}
if (o instanceof JSONArray || o instanceof JSONObject || o instanceof Boolean) {
return o.toString();
}
try {
if (o instanceof Collection) {
return new JSONArray((Collection) o).toString();
} else if (o.getClass().isArray()) {
return new JSONArray(o).toString();
}
if (o instanceof Map) {
return new JSONObject((Map) o).toString();
}
if (o instanceof Number) {
return JSONObject.numberToString((Number) o);
}
if (o instanceof Character || o instanceof CharSequence) {
return JSONObject.quote(String.valueOf(o));
}
if (o.getClass().getPackage().getName().startsWith("java.")) {
return JSONObject.quote(o.toString());
}
} catch (final Exception ignored) {
}
return JSONObject.NULL.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy