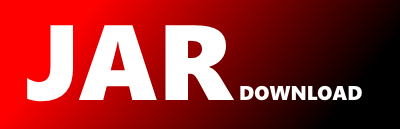
com.sdl.webapp.tridion.linking.AbstractLinkResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dxa-common Show documentation
Show all versions of dxa-common Show documentation
DXA Common project contains framework common classes shared between all other artifacts
package com.sdl.webapp.tridion.linking;
import com.google.common.base.Strings;
import com.sdl.dxa.caching.NamedCacheProvider;
import com.sdl.dxa.caching.statistics.CacheStatisticsProvider;
import com.sdl.dxa.common.util.PathUtils;
import com.sdl.dxa.tridion.annotations.impl.ValueAnnotationLogger;
import com.sdl.webapp.common.api.content.LinkResolver;
import com.sdl.webapp.common.util.TcmUtils;
import lombok.AllArgsConstructor;
import lombok.Getter;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.math.NumberUtils;
import org.jetbrains.annotations.Contract;
import org.jetbrains.annotations.Nullable;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.beans.factory.InitializingBean;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
import javax.cache.Cache;
import java.util.UUID;
@Slf4j
@Component
public abstract class AbstractLinkResolver implements LinkResolver, InitializingBean {
private static final Logger LOG = LoggerFactory.getLogger(AbstractLinkResolver.class);
@Autowired
private NamedCacheProvider namedCacheProvider;
@Autowired
private CacheStatisticsProvider cacheStatisticsProvider;
@Value("${dxa.web.link-resolver.remove-extension:#{true}}")
private boolean shouldRemoveExtension;
@Value("${dxa.web.link-resolver.strip-index-path:#{true}}")
private boolean shouldStripIndexPath;
@Value("${dxa.web.link-resolver.keep-trailing-slash:#{false}}")
private boolean shouldKeepTrailingSlash;
@Override
public String resolveLink(@Nullable String url, @Nullable String localizationId, boolean resolveToBinary, @Nullable String contextId) {
if (!isCacheEnabled()) {
return processLink(url, localizationId, resolveToBinary, contextId);
}
else {
String cacheKeyInput = String.format("%s%s%b%s", url, localizationId, resolveToBinary, contextId);
String cacheKey = UUID.nameUUIDFromBytes(cacheKeyInput.getBytes()).toString();
Cache
© 2015 - 2025 Weber Informatics LLC | Privacy Policy